Basic TypeScript
Modules and Namespaces in TypeScript
As applications grow in complexity, it's important to organize code into more manageable parts. TypeScript provides two main ways to achieve this: modules and namespaces. In this chapter, we will see how to use them to improve the organization of our projects.
Modules in TypeScript
A module in TypeScript is any file that contains code. Each file in TypeScript can act as an independent module. You can export any part of the code from a file and then import it into other files as needed.
Module Exporting
For a function, class, or variable to be available outside the file in which it is defined, you must export it. There are two main ways to export in TypeScript: named export and default export.
esta imagen muestra un cheatsheat de modulos en typescript
Named Export
With named export, you can export multiple items from a file and specify what you want to import into the other file.
typescript
Named Import
Once the items are exported, you can import them into other files using the import
syntax.
typescript
Default Export
The default export allows you to export a single item from a file. This item can be imported without using its exact name.
typescript
Default Import
When importing a default item, you can use any name to reference it in your file.
typescript
Namespaces in TypeScript
Namespaces are another way to organize code, especially when working on large projects. Unlike modules, namespaces group code within a single file or several combined files. Namespaces are useful when you want to keep all related code under the same global name.
Defining a Namespace
To define a namespace, use the namespace
keyword followed by a name. Inside this namespace, you can declare classes, functions, variables, etc.
typescript
Nested Namespaces
You can nest namespaces within other namespaces to better organize code.
typescript
Conclusion
In this chapter, we have learned how to use modules and namespaces to organize code in TypeScript. Modules are a modern and flexible way to divide code into different files, while namespaces provide a way to group code within a single file or multiple ones. Both are powerful tools that help keep code clean and structured.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
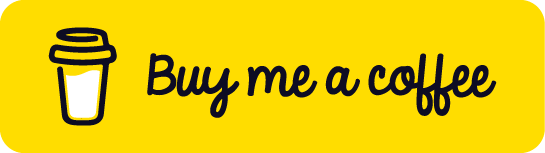
Chat with Chuck
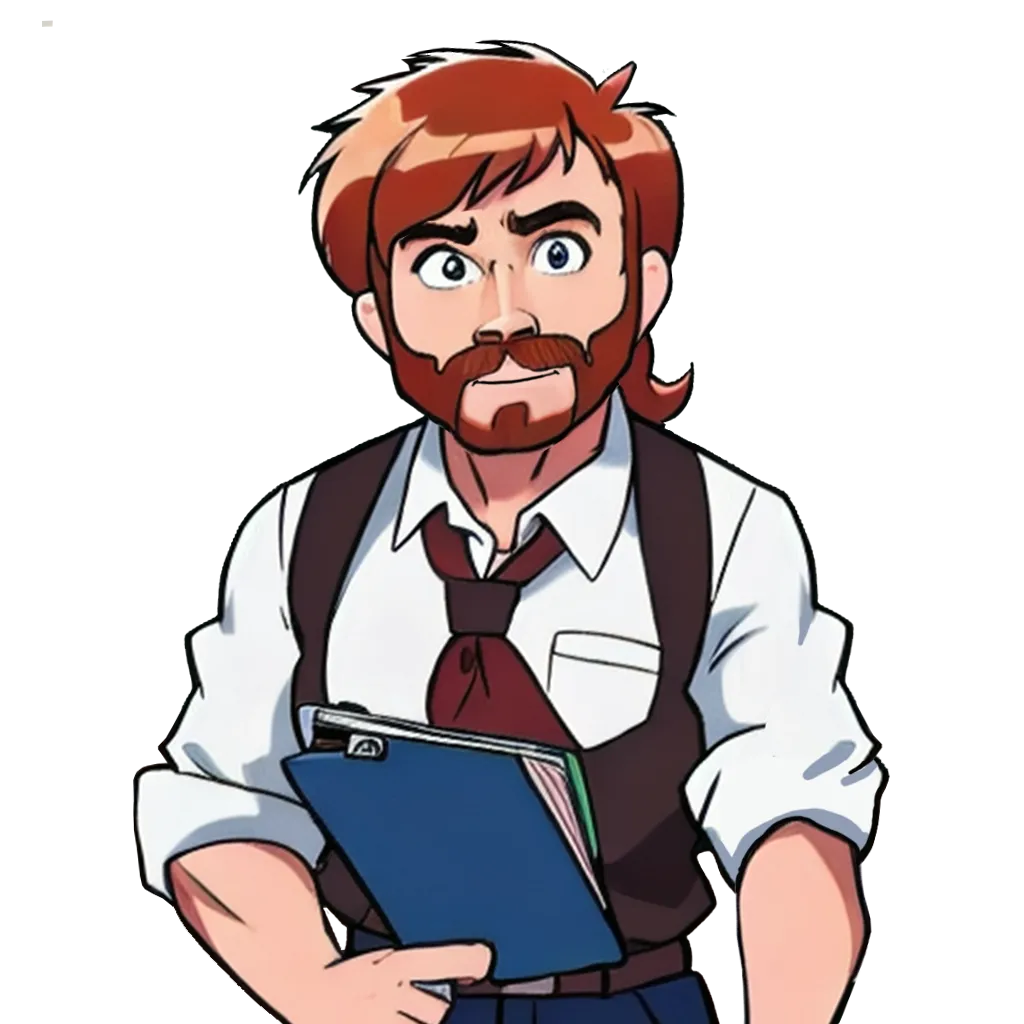
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps