Basic TypeScript
Integration with JavaScript Libraries
TypeScript makes it easier to use JavaScript libraries within projects that utilize its static typing. However, working with libraries not written in TypeScript can present some challenges. In this chapter, we will explore how to integrate TypeScript with JavaScript libraries and how to handle typing issues that may arise.
Using JavaScript Libraries with TypeScript
TypeScript allows you to use any JavaScript library, but to take full advantage of static typing, it's helpful if these libraries provide type definitions. When a library does not have included type definitions, we can install type definition files from the DefinitelyTyped
repository.
Installing Library Types
Many popular libraries have type definitions available in DefinitelyTyped
, which can be installed using npm or yarn. The type definition files are in packages whose names start with @types
.
bash
Using Libraries with Type Definitions
Once the types are installed, you can use the library in TypeScript just as you would in a normal JavaScript project, but with the advantages of static typing.
typescript
Libraries without Type Definitions
If a library doesn't have type definitions available, you can still use it in TypeScript, although you'll lose the benefits of static typing. For this, you can use the type any
to disable type checking.
Example of Use with any
typescript
However, this can make the code more prone to errors. Therefore, a better solution is to create custom type definitions.
Creating Custom Type Definitions
If the library you are using doesn't have type definitions, you can create your own definition files to help TypeScript understand how the library works.
Basic Type Definitions
Type definitions are stored in files with the extension .d.ts
. These files define how TypeScript should interpret the functions and variables of the library.
typescript
Using Custom Definitions
Once you've created the definition file, you can use the library as if it were fully typed.
typescript
Compatibility between TypeScript and JavaScript
TypeScript is fully compatible with JavaScript, which means you can mix JavaScript and TypeScript code within the same project. This is useful when working on projects that are gradually migrating from JavaScript to TypeScript.
Using JavaScript Code in TypeScript Projects
To include JavaScript files in a TypeScript project, simply place them in the project paths and ensure the tsconfig.json
file includes them in its configuration.
json
Conclusion
In this chapter, we explored how to integrate JavaScript libraries into TypeScript projects, including how to handle libraries that do not provide type definitions. We learned how to install existing type definitions and to create our own custom definitions when necessary.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
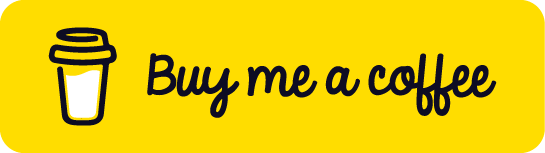
Chat with Chuck
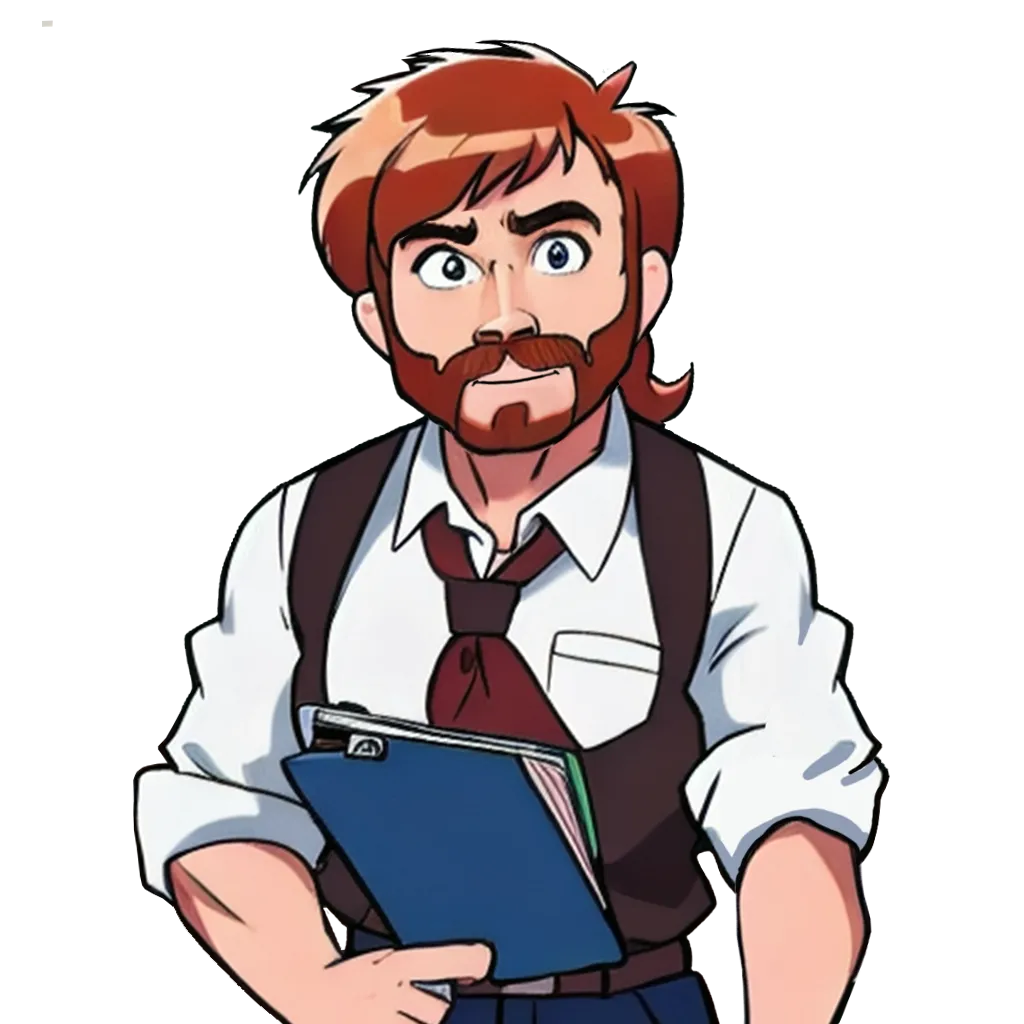
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps