Basic TypeScript
Generics in TypeScript
Generics are one of the most powerful features of TypeScript. They allow you to create components that work with a variety of data types, rather than being limited to a single type. In this chapter, we will explore how generics work in functions, classes, and interfaces to write more flexible and reusable code.
Introduction to Generics
Generics allow a function, class, or interface to work with multiple data types without specifying the exact type beforehand. This makes the code more flexible and reusable.
This image shows an example of Generics
Basic Example of Generics
Let's look at a simple example of a function that uses a generic:
typescript
When calling the function, TypeScript infers the type of the argument being passed:
typescript
Generics in Functions
Generics are very useful when writing functions that need to handle multiple types. We can also apply constraints to generics to limit the types that can be used.
Example with Constraints
We can constrain the generic type to ensure it meets certain conditions, using the extends
keyword.
typescript
Generics in Classes
Generics can also be applied to classes, allowing a class to work with different data types.
Example of a Generic Class
typescript
Generics in Interfaces
Interfaces can also be generic, allowing you to define data structures that work with multiple types.
Example of a Generic Interface
typescript
Using Generics with Default Types
It's possible to assign a default value to generic parameters, meaning if no specific type is provided, the default type will be used.
Example of Default Type
typescript
Conclusion
In this chapter, we have learned about generics in TypeScript and how they allow us to write more flexible and reusable functions, classes, and interfaces. Generics are a powerful tool for working with a variety of types without having to duplicate code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
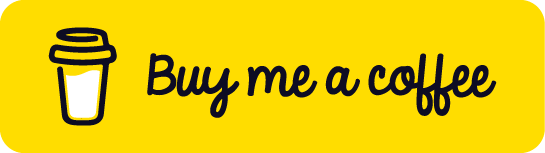
Chat with Chuck
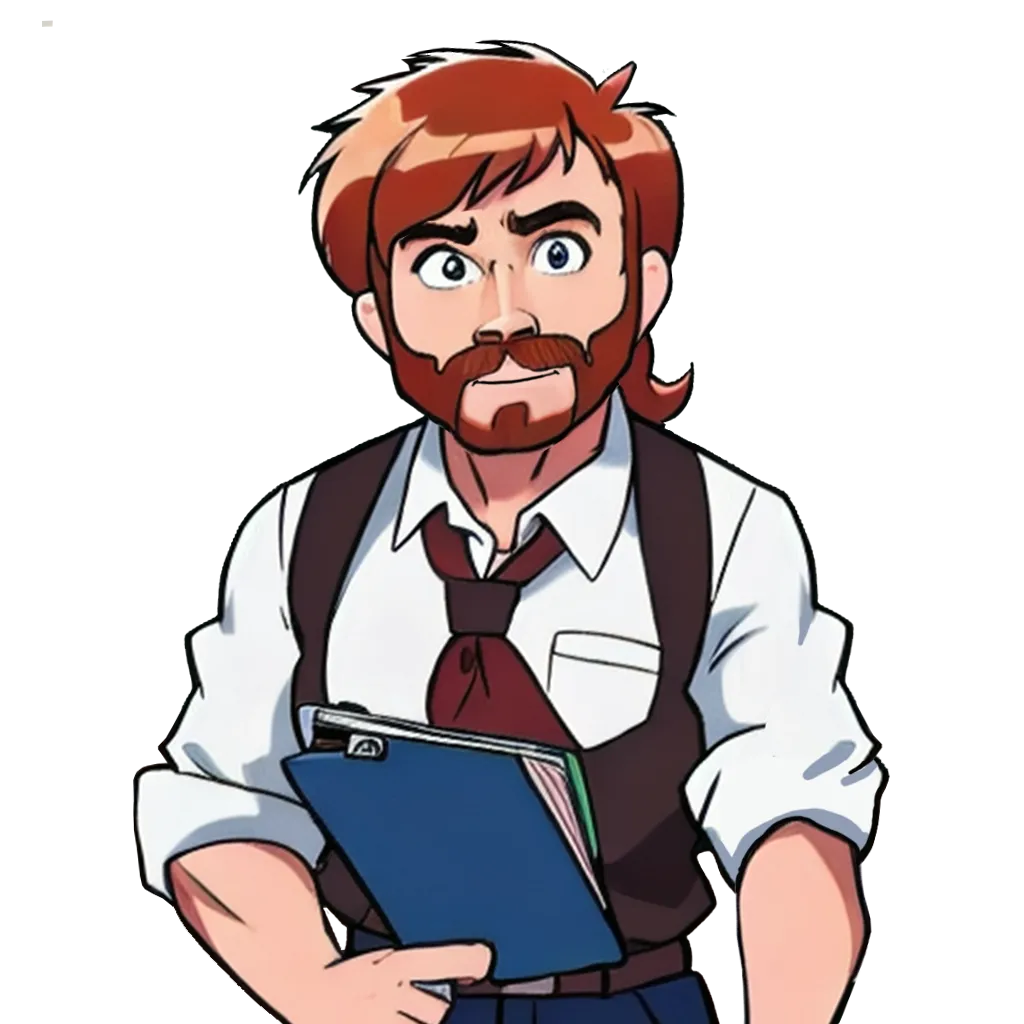
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps