Basic TypeScript
Good Practices and Patterns in TypeScript
Adopting good practices and design patterns when writing TypeScript code not only improves code readability and maintainability but also helps avoid common errors and optimize performance. In this chapter, we will explore some of the best practices and design patterns you can apply in your TypeScript projects.
Using Strict Typing
Using strict typing is essential to make the most of TypeScript's capabilities. Enabling strict
in the tsconfig.json
file activates several settings that help avoid type-related errors.
Strict Typing Configuration
json
Prefer Specific Types over any
One of the major advantages of TypeScript is static typing. Whenever possible, it is better to avoid using the any
type, as it removes the benefits of type checking. Instead, use more specific or generic types.
Example of Using Specific Types
typescript
Using Interfaces and Types
Interfaces and types are essential for structuring code and ensuring that objects have the correct properties. Use interfaces to define the shape of objects and ensure that all necessary properties are present.
Example of Using Interfaces
typescript
Immutability
Whenever possible, use immutable variables to avoid unexpected changes in state. In TypeScript, you can do this by using const
to declare variables that should not be reassigned.
Example of Immutability
typescript
Avoiding Implicit Types
Although TypeScript can infer the type of a variable, it's better to be explicit with the type, especially in large projects. This improves readability and makes debugging easier.
Example of Explicit Typing
typescript
Common Design Patterns in TypeScript
Singleton
The Singleton pattern ensures that a class has only one instance and provides a global access point to it. This pattern is useful when you need a single instance of a class to manage global state or application configuration.
Example of the Singleton Pattern
typescript
Factory
The Factory pattern is useful when you have complex logic for creating objects. It allows objects to be created without specifying the exact class of the object to be created.
Example of the Factory Pattern
typescript
Decorator
The Decorator pattern allows for dynamically adding new functionalities to objects by wrapping them in decorator objects.
Example of the Decorator Pattern
typescript
Using TSLint or ESLint
To ensure that the code follows best practices and standards, it is recommended to use linting tools like TSLint or ESLint. These tools analyze your code and help you correct errors or inconsistencies.
Installing ESLint
bash
Conclusion
In this chapter, we have explored various best practices and design patterns in TypeScript, including the use of strict typing, immutability, and patterns like Singleton, Factory, and Decorator. Applying these best practices will help you write cleaner, more maintainable, and scalable code in your TypeScript projects.
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps
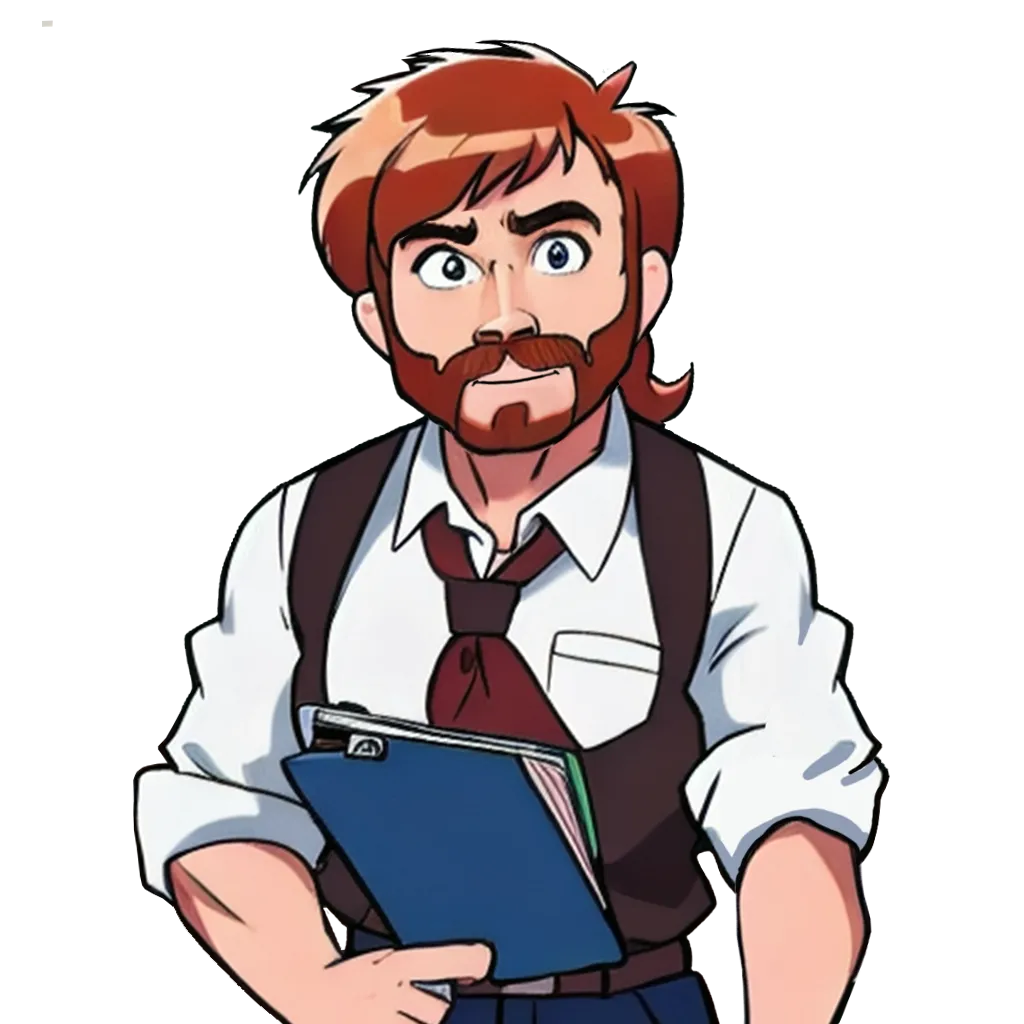