Basic TypeScript
Testing in TypeScript
Testing is an essential part of software development, and TypeScript provides various tools and libraries to efficiently perform testing. In this chapter, we will learn how to set up a testing environment for TypeScript and how to write unit and integration tests to ensure that our code works correctly.
Setting Up the Testing Environment
The first step to writing tests in TypeScript is to set up a suitable environment. There are several testing libraries that are compatible with TypeScript, such as Jest and Mocha. In this chapter, we will use Jest as an example since it is one of the most popular libraries.
Installing Jest with TypeScript
This image shows a logo of Typescript plus jest
To start, we need to install Jest and the necessary tools to integrate it with TypeScript.
bash
After installing the dependencies, we need to create a configuration file for Jest:
bash
Configuring jest.config.js
The jest.config.js
file allows customizing the behavior of Jest. Here is an example of a basic configuration:
javascript
Writing Unit Tests
Unit tests verify the behavior of individual units of code, such as functions or classes. Let's see how to write a basic unit test using Jest and TypeScript.
Example of a Unit Test
Suppose we have the following function in a math.ts
file:
typescript
Now we will write a test for this function in a math.test.ts
file:
typescript
Running the Tests
To run the tests, simply execute the following command:
bash
Asynchronous Tests
In TypeScript, we can also write tests for asynchronous functions using async
and await
. Let's see an example of a test for an asynchronous function that makes a request to an API.
Example of an Asynchronous Test
Suppose we have the following function in an api.ts
file:
typescript
The test for this function would be as follows:
typescript
Mocking in TypeScript
Mocking is a technique used to simulate external dependencies in tests, which allows isolating the unit being tested. Jest provides tools to mock functions and modules.
Example of Mocking
Suppose we have a function that depends on a call to an external API:
typescript
We can simulate the API response using mocks in our tests:
typescript
Code Coverage
Code coverage measures what percentage of our code is being tested. Jest includes a built-in tool to generate code coverage reports.
To generate a coverage report, execute the following command:
bash
Conclusion
In this chapter, we have learned how to set up a testing environment in TypeScript using Jest, how to write unit and asynchronous tests, and how to use mocks to simulate external dependencies. We also saw how to measure code coverage to ensure that our project is well tested.
- Introduction to TypeScript
- Data Types in TypeScript
- Strict and Optional Typing in TypeScript
- Functions in TypeScript
- Interfaces and Types in TypeScript
- Clases y Orientación a Objetos en TypeScript
- Modules and Namespaces in TypeScript
- Generics in TypeScript
- Advanced Typing in TypeScript
- Decorators in TypeScript
- Error Handling in TypeScript
- TypeScript Project Setup
- Integration with JavaScript Libraries
- Testing in TypeScript
- Modularization and Dependency Management in TypeScript
- Webpack and TypeScript Configuration
- TypeScript in React
- TypeScript in Node.js
- Good Practices and Patterns in TypeScript
- Migration from JavaScript to TypeScript
- Conclusions and Next Steps
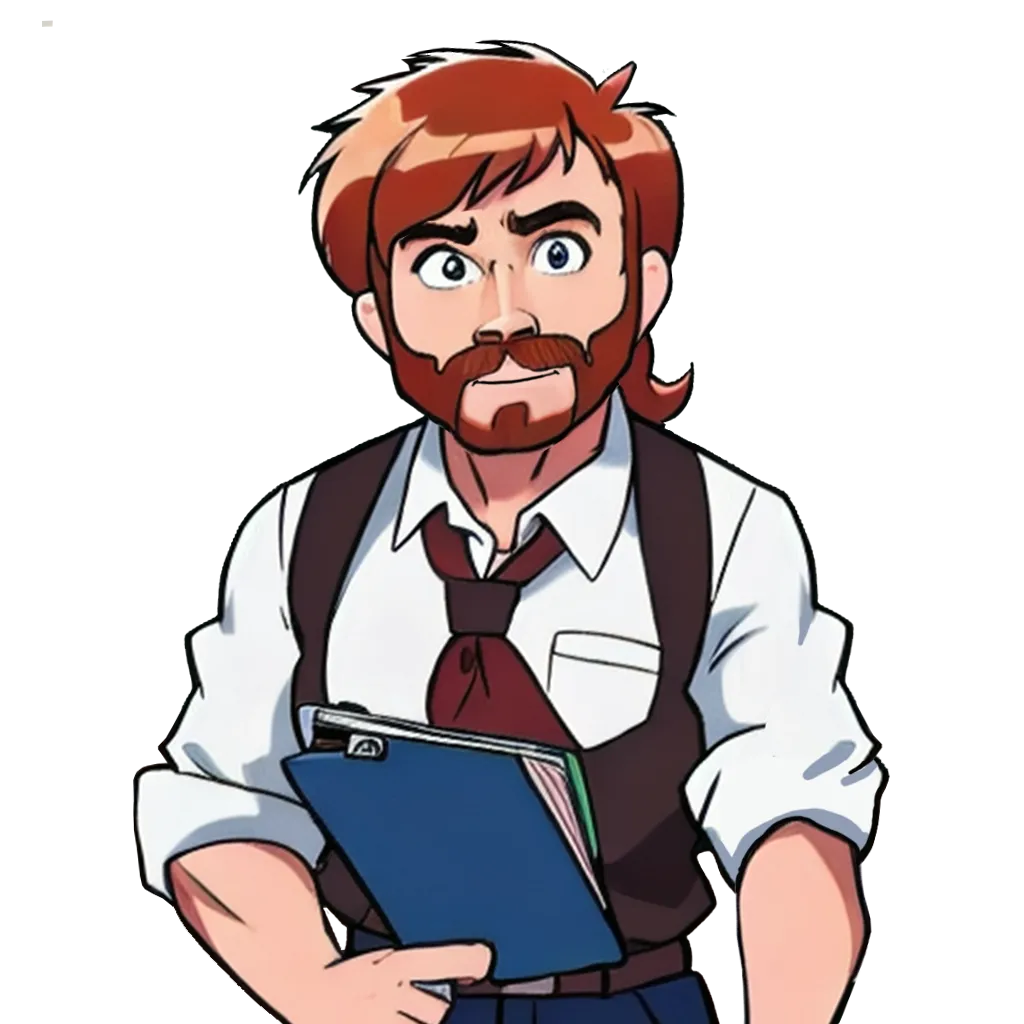