Basic JavaScript
Working with APIs
APIs (Application Programming Interfaces) allow different applications to communicate with each other. In web development, APIs are crucial for exchanging data between the client and the server or between different services. In this chapter, you will learn how to work with APIs using JavaScript, especially how to make HTTP requests to retrieve data and how to process the responses.
What is an API?
An API is a set of rules and protocols that allow different applications to communicate with each other. In the context of web development, APIs often provide a structured way to access server data through HTTP requests. For example, you might use an API to get weather data, access a user database, or send data to a server.
HTTP Requests
HTTP requests are the foundation of communication with a web API. The most common HTTP methods you will use are:
- GET: To retrieve data from the server.
- POST: To send data to the server.
- PUT: To update data on the server.
- DELETE: To delete data from the server.
Basic Example of a GET Request
You can make HTTP requests in JavaScript using the fetch
API, which is native to modern browsers.
javascript
Basic Example of a POST Request
A POST request is used when you need to send data to the server, such as the details of a new user or a new blog post. With fetch
, you can make a POST request as follows:
javascript
Handling Responses and Errors
When making an HTTP request, it is important to handle both successful responses and possible errors. In addition to network errors, APIs can return HTTP status codes indicating whether the request was successful or not.
Handling HTTP Status Codes
HTTP status codes are useful for determining if the request was successful. For example:
- 200: The request was successful.
- 201: Resource successfully created (for POST).
- 400: Invalid request.
- 404: Resource not found.
- 500: Server error.
You can check the status code in the response before processing the data.
javascript
Authentication in APIs
Some APIs require authentication to ensure that only authorized users can access the data. This is achieved through authentication tokens, which are sent in the request headers.
Authentication with Tokens
An authentication token is usually provided after a user authenticates in a system and must be sent in the headers of each subsequent request.
javascript
Public and Private APIs
There are two main types of APIs:
- Public APIs: These are accessible to any user without restrictions. Examples include weather or news APIs.
- Private APIs: These require authentication and only allow access to authorized users or applications. These are common in internal applications or services that handle sensitive data.
Example of a Public API
javascript
REST APIs vs. GraphQL
In the world of APIs, there are different architectural styles. The most common is REST, which organizes resources in URLs and uses HTTP methods to interact with them. However, there is also GraphQL, which allows clients to specify exactly what data they want in a single request.
Key Differences between REST and GraphQL
- REST: Each resource has its own endpoint. You need to make multiple requests to obtain related data.
- GraphQL: Everything is centralized in a single endpoint, and the client specifies exactly what data is needed in a single query.
While REST remains the most common option, GraphQL is gaining popularity due to its flexibility and efficiency in handling complex data.
Conclusion
Working with APIs is an essential skill for any modern web developer. In this chapter, you have learned how to make HTTP requests with JavaScript using fetch
, handle responses and errors, and how to work with APIs that require authentication.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
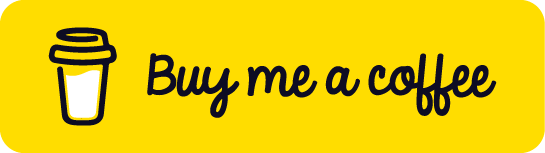
Chat with Chuck
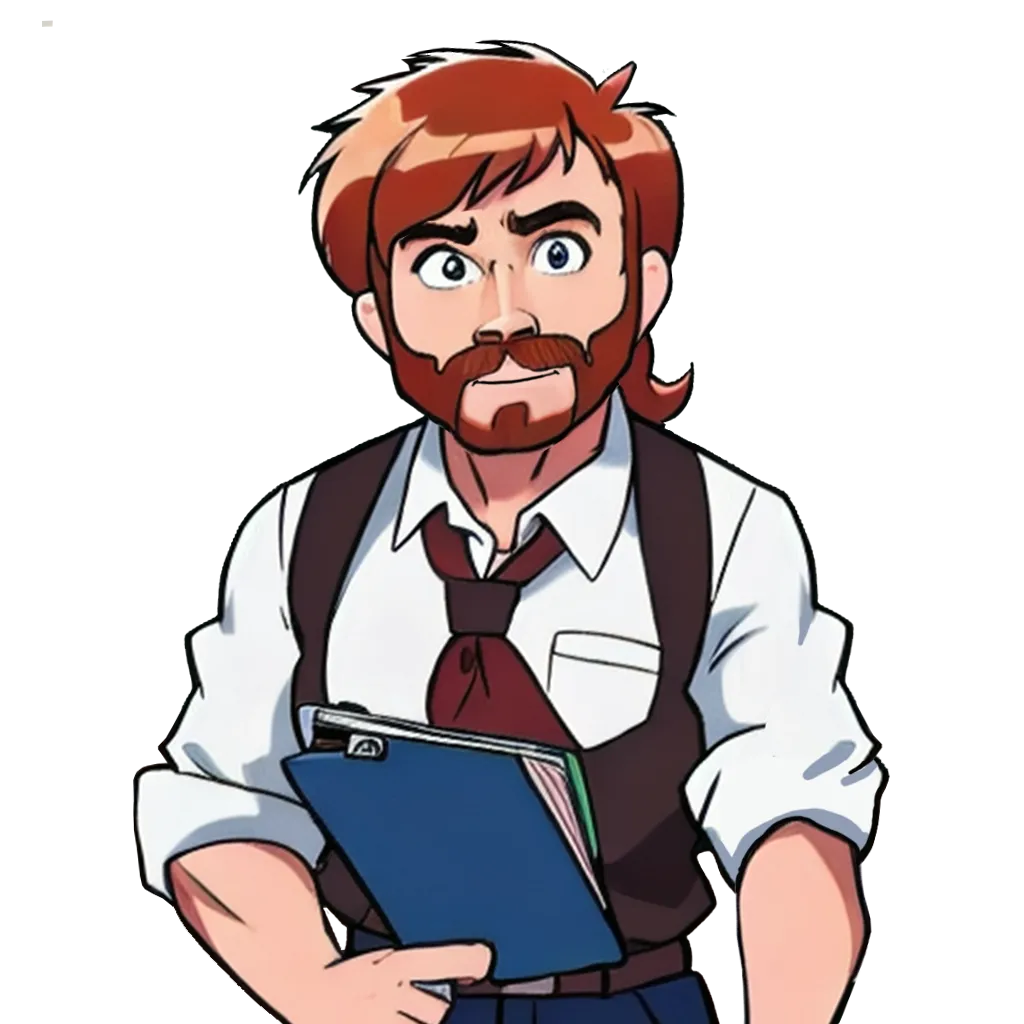
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript