Basic JavaScript
Testing in JavaScript
Testing is a fundamental part of software development. It ensures that the code works as expected and prevents errors that could occur in production. In this chapter, you will learn how to perform testing in JavaScript, the types of tests that exist, and the most commonly used tools and frameworks to automate these tests.
Importance of Testing
Testing helps detect errors and ensures that your application's functions meet the defined expectations. Some key benefits include:
- Prevention of regressions: Tests ensure that old functionalities are not affected when new code is introduced.
- More reliable code: Tests allow you to detect errors early in the development process, improving the quality of the final product.
- Facilitates maintenance: A well-structured set of tests makes it easier to make changes or refactor the code without fear of breaking existing functionalities.
Types of Testing
1. Unit Testing
Unit testing verifies the behavior of individual functions or small units of code in isolation. These tests ensure that a function, module, or class works correctly without relying on other components.
javascript
2. Integration Testing
Integration testing verifies that different parts of the system work correctly when combined. It ensures that modules interact as expected.
javascript
3. Functional Testing
Functional testing verifies that the application functions according to the specifications and business requirements. It generally simulates how users interact with the system.
4. End-to-End (E2E) Testing
End-to-end tests ensure that the application works correctly from the user's perspective, testing the entire system rather than individual functions.
Tools and Frameworks for Testing in JavaScript
1. Jest
Jest is one of the most popular testing frameworks for JavaScript. It was developed by Facebook and provides a comprehensive solution for unit, integration, and snapshot testing. It's easy to configure and comes with many useful features, like parallel test execution and automatic mocks.
javascript
2. Mocha and Chai
Mocha is a flexible framework for running tests in JavaScript, while Chai is an assertion library that makes it easy to verify test results.
javascript
3. Cypress
Cypress is an end-to-end testing tool that allows functional tests to be performed directly in the browser. It is ideal for simulating the user's experience.
javascript
4. Sinon
Sinon is a library used to create mocks, spies, and stubs in JavaScript. It is very useful for simulating the behavior of external dependencies or functions during tests.
javascript
Best Practices for Testing
-
Write Small and Isolated Tests: Ensure that each test verifies a single unit of functionality to avoid confusion and false positives.
-
Write Tests Before Coding: Adopting the practice of Test-Driven Development (TDD) helps you write more robust code from the start.
-
Automate Your Tests: Integrate automation tools to run your tests continuously, for example, using CI/CD pipelines.
-
Keep It Simple: Ensure that your tests are easy to read and maintain. Avoid overloading tests with too many checks in a single function.
Conclusion
Testing in JavaScript is crucial to ensure the quality, reliability, and stability of web applications. In this chapter, we have explored the different types of tests, the most popular tools, and how to implement them to ensure that your code works correctly.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
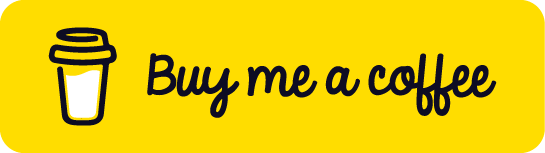
Chat with Chuck
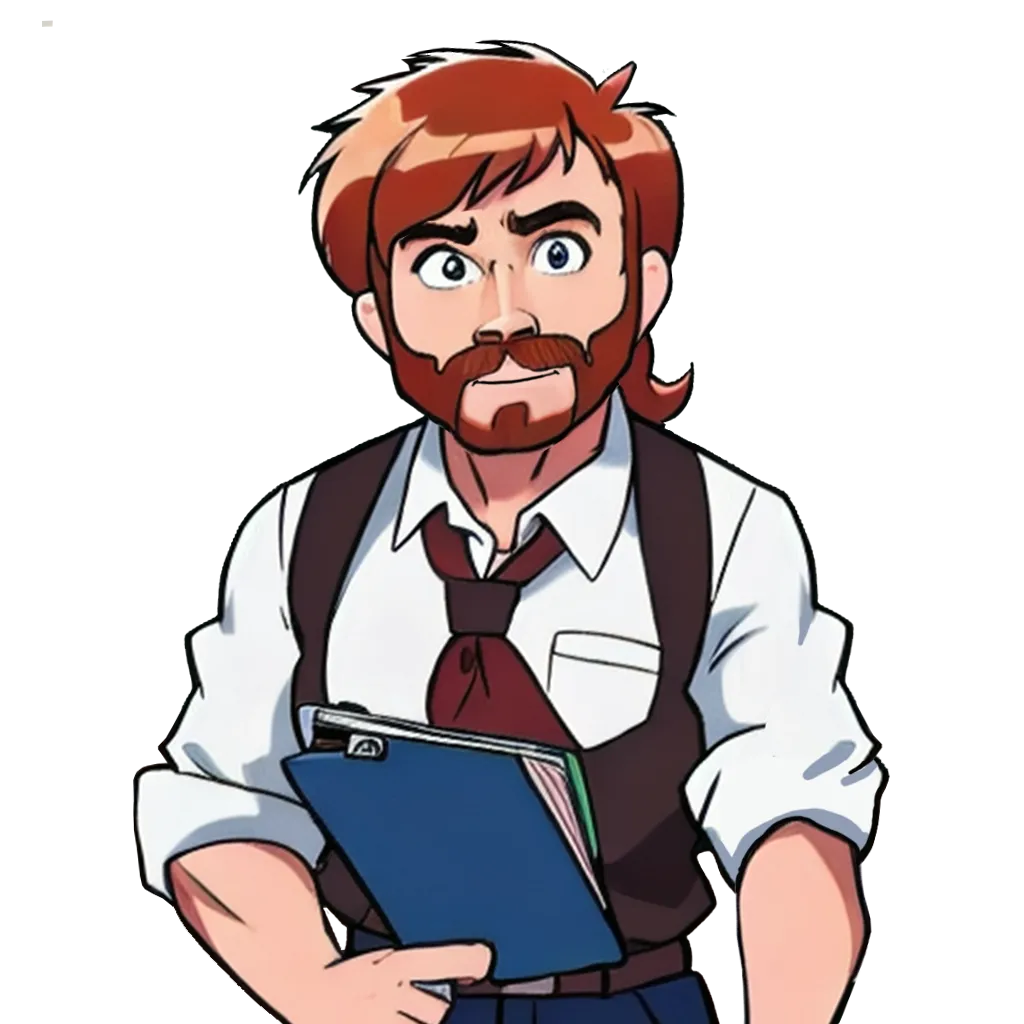
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript