Basic JavaScript
Functions forEach and map
The forEach
and map
functions are two essential methods for iterating and transforming arrays in JavaScript. In this chapter, you will learn how to use these functions with practical examples and common use cases, including some details on performance and best practices.
forEach
The forEach
method executes a function on each element of an array. It is useful for performing actions on the elements of an array but does not return a new array.
Example of forEach
javascript
Performance Considerations
Although forEach
is simple and easy to use, it may not be the best choice if your goal is to transform an array. This is because forEach
does not return a new array, and if you need to mutate the original array, other techniques like map
or reduce
might be more suitable.
map
The map
method creates a new array from the results of applying a function to each element of the original array. It is perfect when you want to transform data without mutating the original array.
Example of map
javascript
Using map on Arrays of Objects
The map
method is particularly useful when working with arrays of objects, as it allows you to easily transform values within those objects.
javascript
Conclusion
The forEach
and map
functions are powerful tools for iterating and transforming arrays. While forEach
is ideal for executing actions on the elements, map
is the best choice when you want to transform an array without modifying the original.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
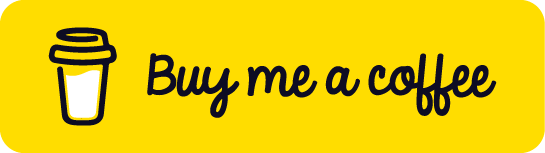
Chat with Chuck
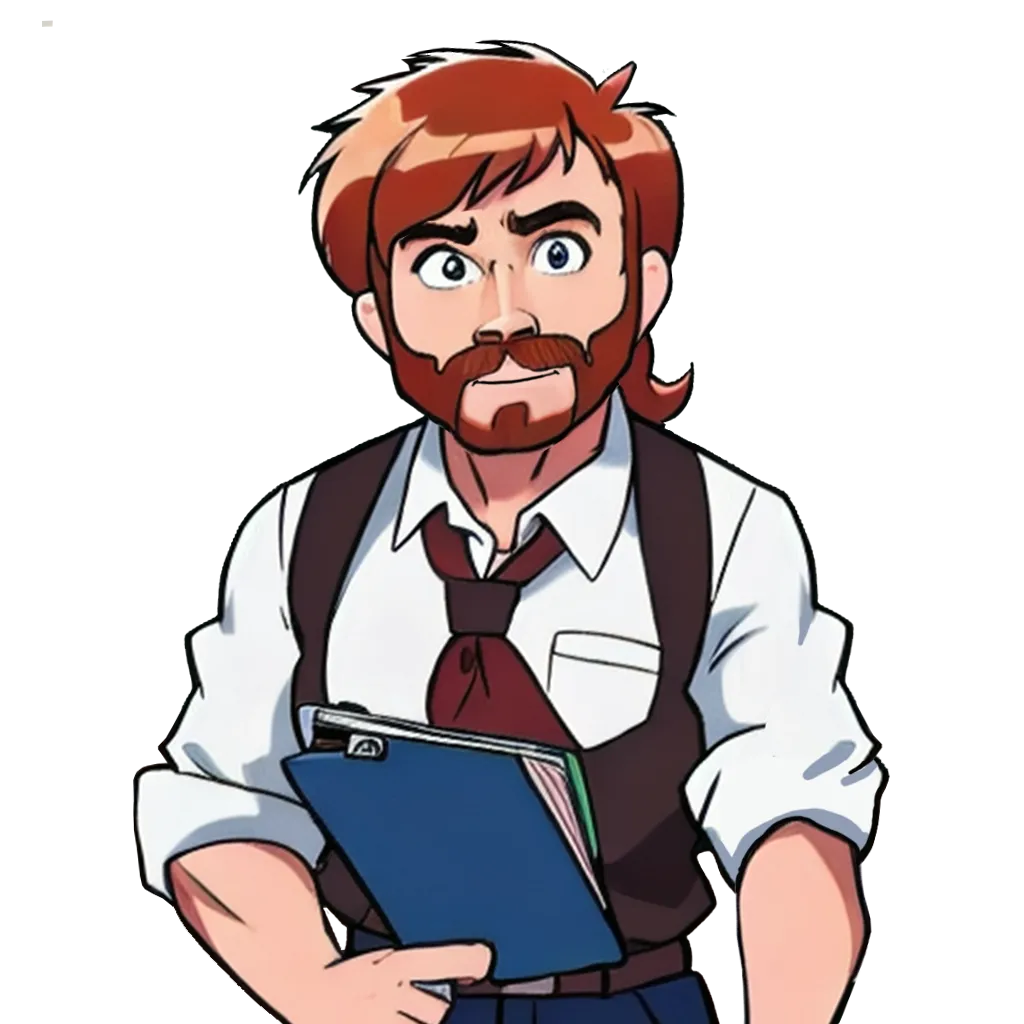
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript