Basic JavaScript
Advanced Functions
Functions are a fundamental pillar of JavaScript and play a crucial role in modern programming. In this chapter, we will explore some advanced features of functions, such as higher-order functions, arrow functions, and closures. These features will allow you to write more efficient and reusable code.
Higher-Order Functions
A higher-order function is a function that accepts other functions as arguments or returns a function. This is a key concept in functional programming and allows you to create more modular and reusable code.
Example of a Higher-Order Function
javascript
In this example, greet
is a higher-order function because it returns another function. This approach is useful for creating more specific functions from more general ones.
Arrow Functions
Arrow functions are a more concise syntax for defining functions. One of the key differences from traditional functions is how they handle the value of this
, which we will see later.
Arrow Function Syntax
The basic syntax of an arrow function is as follows:
javascript
When an arrow function has more than one line of code in its body, you must use braces {}
and the keyword return
to return a value.
javascript
Handling this
in Arrow Functions
One of the most important differences between arrow functions and traditional functions is how they handle the value of this
. In arrow functions, the value of this
is bound to the context in which the function was defined, not the context in which it is invoked.
javascript
Arrow functions should not be used as object methods if you need to access this
. In these cases, it is preferable to use regular functions.
Closures
A closure is a function that remembers the environment in which it was created, even after that environment has ceased to exist. This allows a function to access variables from an external function even after it has finished executing.
Example of a Closure
javascript
In this example, the inner function has access to the variable counter
because it is a closure. This technique is useful for creating functions that have private states.
Functions as Arguments
In JavaScript, functions are first-class citizens, meaning they can be passed as arguments to other functions. This allows for a high level of flexibility and power in programming.
Example of Functions as Arguments
javascript
This example demonstrates how you can pass functions as arguments to dynamically change a function's behavior.
Recursion
Recursion is a technique where a function calls itself. It is useful for solving problems that can be divided into similar subproblems.
Example of Recursion
javascript
Recursion is a powerful tool, but it can be difficult to understand and can cause performance issues if not used correctly.
Conclusion
In this chapter, we have covered advanced concepts about functions in JavaScript, including higher-order functions, arrow functions, closures, and recursion. These features will allow you to write more modular, reusable, and efficient code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
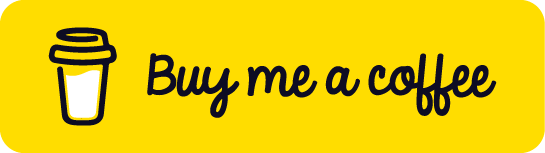
Chat with Chuck
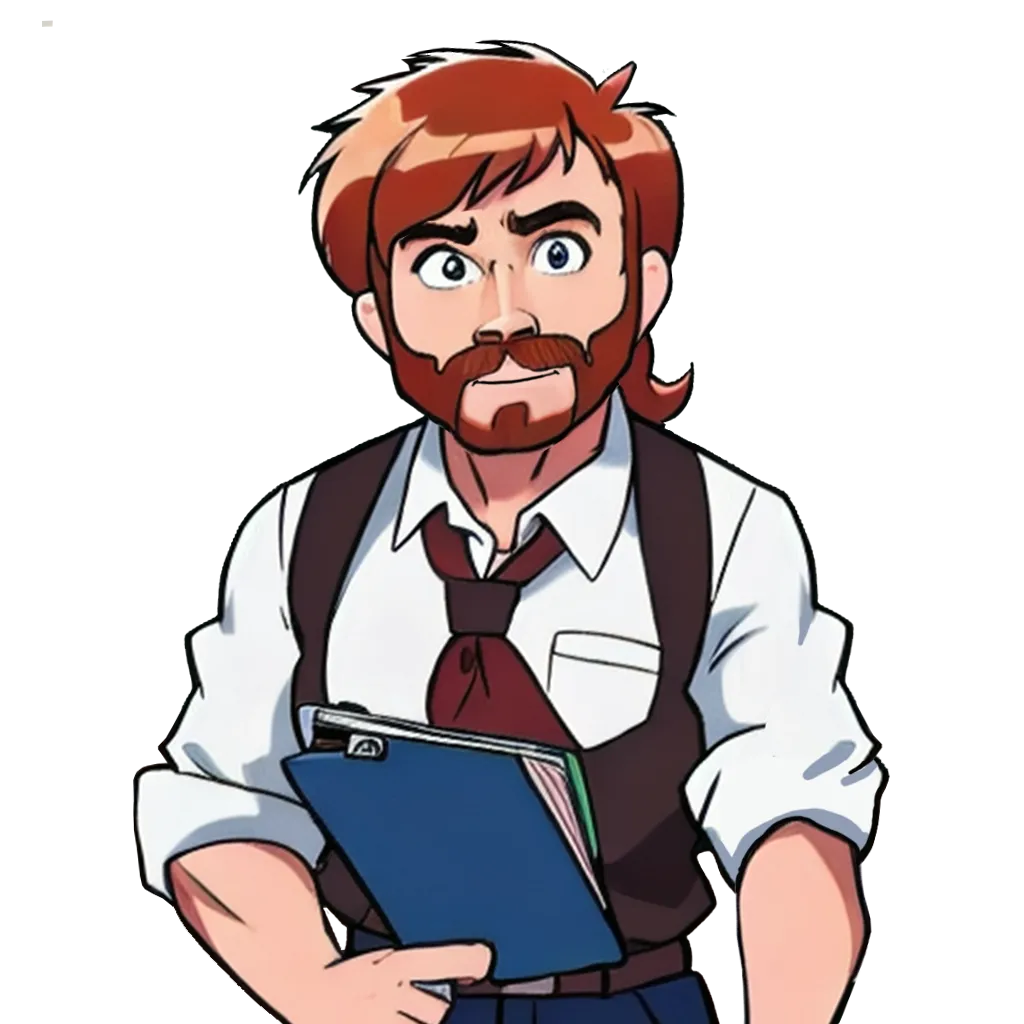
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript