Basic JavaScript
Handling Dates and Times in JavaScript
Working with dates and times is an important part of developing web applications. Whether you're displaying a date on an interface, calculating the difference between two dates, or handling times in different time zones, JavaScript provides various tools to manage these scenarios. In this chapter, we will learn how to work with dates and times using the Date
object and some popular libraries that simplify the process.
The Date Object
In JavaScript, the Date
object is the main mechanism for handling dates and times. With this object, you can easily create, manipulate, and format dates.
Creating a Date Object
To create a new instance of Date
, you can use the constructor without arguments, which will return the current date and time:
javascript
You can also create a Date
object from a specific date using a string or individual components:
javascript
Methods for Getting Date Components
The Date
object has several methods to get the different components of a date, such as the year, month, day, and more.
javascript
Methods for Modifying a Date
You can also modify the components of a Date
object using methods like setFullYear
, setMonth
, and setDate
.
javascript
Timestamps
A timestamp is the number of milliseconds that have elapsed since January 1, 1970 (known as the Unix epoch). The Date
object allows you to obtain and manipulate timestamps.
Getting a Timestamp
You can get the current timestamp using the getTime
method.
javascript
Comparing Dates Using Timestamps
Timestamps are also useful for comparing dates. You can subtract two timestamps to get the difference in milliseconds.
javascript
Formatting Dates
Formatting a date to display it in a readable format can be tricky using just the Date
object. Although JavaScript does not have a native method for flexibly formatting dates, you can use methods like toLocaleDateString
to format dates according to language and regional preferences.
Date Formatting Example
javascript
Popular Libraries for Date Handling
Although the Date
object is useful, handling dates and times in JavaScript can become complicated, especially when dealing with time zones or more complex dates. To simplify handling dates, there are popular libraries like Moment.js
and Day.js
that offer a more user-friendly API.
Moment.js (Deprecated)
Moment.js
was one of the most used libraries for date handling in JavaScript, although it is now in maintenance mode and has been replaced by lighter alternatives. Nevertheless, it is important to know its functionality.
javascript
Day.js
Day.js
is a modern, lightweight alternative to Moment.js, offering similar functionality with a much smaller footprint.
javascript
Time Zones
Handling different time zones can be complicated when working with international applications. The JavaScript Date
object handles dates in the system's local time zone, but you can use the Intl.DateTimeFormat
library to work with other time zones.
Formatting Dates in Different Time Zones
javascript
Conclusion
Working with dates and times is a crucial part of web application development. In this chapter, we've explored how to use the Date
object in JavaScript to create, manipulate, and format dates, as well as how to work with timestamps and time zones. We've also looked at some popular libraries that simplify date handling in JavaScript.
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript
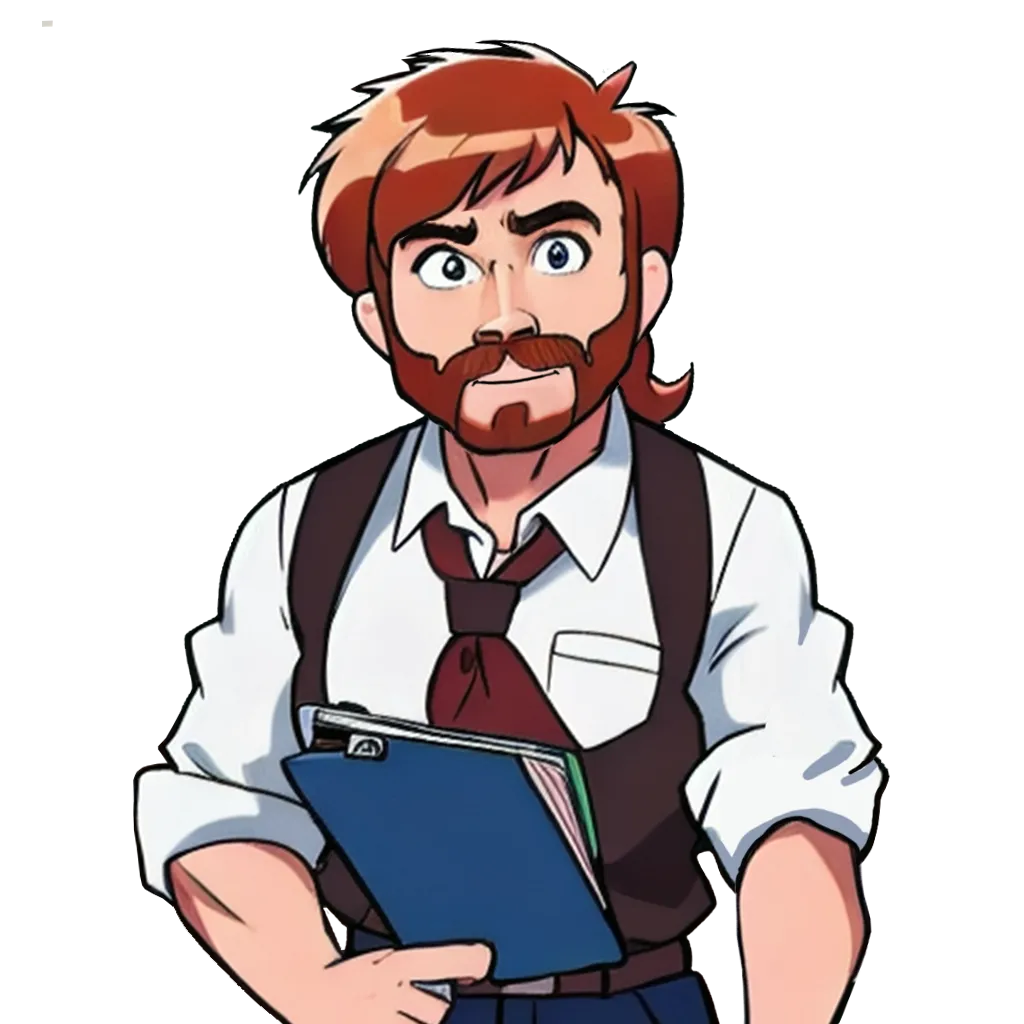