Basic JavaScript
DOM Manipulation
DOM (Document Object Model) manipulation is one of the key skills you need to master as a frontend developer. The DOM is a structured representation of the webpage, allowing JavaScript to access and modify its content and structure in real-time. In this chapter, we will learn how to select, modify, and respond to events in HTML elements using JavaScript.
What is the DOM?
The DOM is a programming interface that represents the structure of an HTML or XML document as a tree of nodes. Each HTML element becomes a node in this tree, and JavaScript can access these nodes to read or change their content, attributes, and style.
Selecting DOM Elements
To manipulate elements on a webpage, we first need to select them. JavaScript provides several methods to do this.
getElementById
This method selects an element in the DOM using its id
attribute.
javascript
querySelector
and querySelectorAll
The querySelector
method selects the first element that matches a CSS selector, while querySelectorAll
selects all elements that match the selector.
javascript
getElementsByClassName
and getElementsByTagName
These methods allow you to select elements based on their class or HTML tag.
javascript
Modifying Content and Attributes
Once you have selected an element, you can modify its content, attributes, and styles using JavaScript.
Modifying Text
You can change the text content of an element using textContent
or innerHTML
.
javascript
Modifying Attributes
You can modify an element's attributes using setAttribute
or by directly accessing the element's properties.
javascript
Styles and Classes
JavaScript also allows you to change the CSS styles and classes of elements.
Modifying Inline Styles
You can modify styles directly using the element's style
property.
javascript
Adding and Removing Classes
To modify an element's CSS classes, you can use the methods classList.add
, classList.remove
, or classList.toggle
.
javascript
JavaScript Events
Events allow JavaScript to respond to user interactions like clicking a button, moving the mouse, or submitting a form. You can use the addEventListener
method to listen for events and execute a function when a specific event occurs.
Click Event
javascript
Other Common Events
Some common events in JavaScript are:
mouseover
: when the mouse moves over an element.keydown
: when a key is pressed.submit
: when a form is submitted.
javascript
Conclusion
In this chapter, you have learned how to manipulate the DOM using JavaScript to select, modify, and react to events in HTML elements. The ability to dynamically interact with the webpage is what makes JavaScript so powerful.
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript
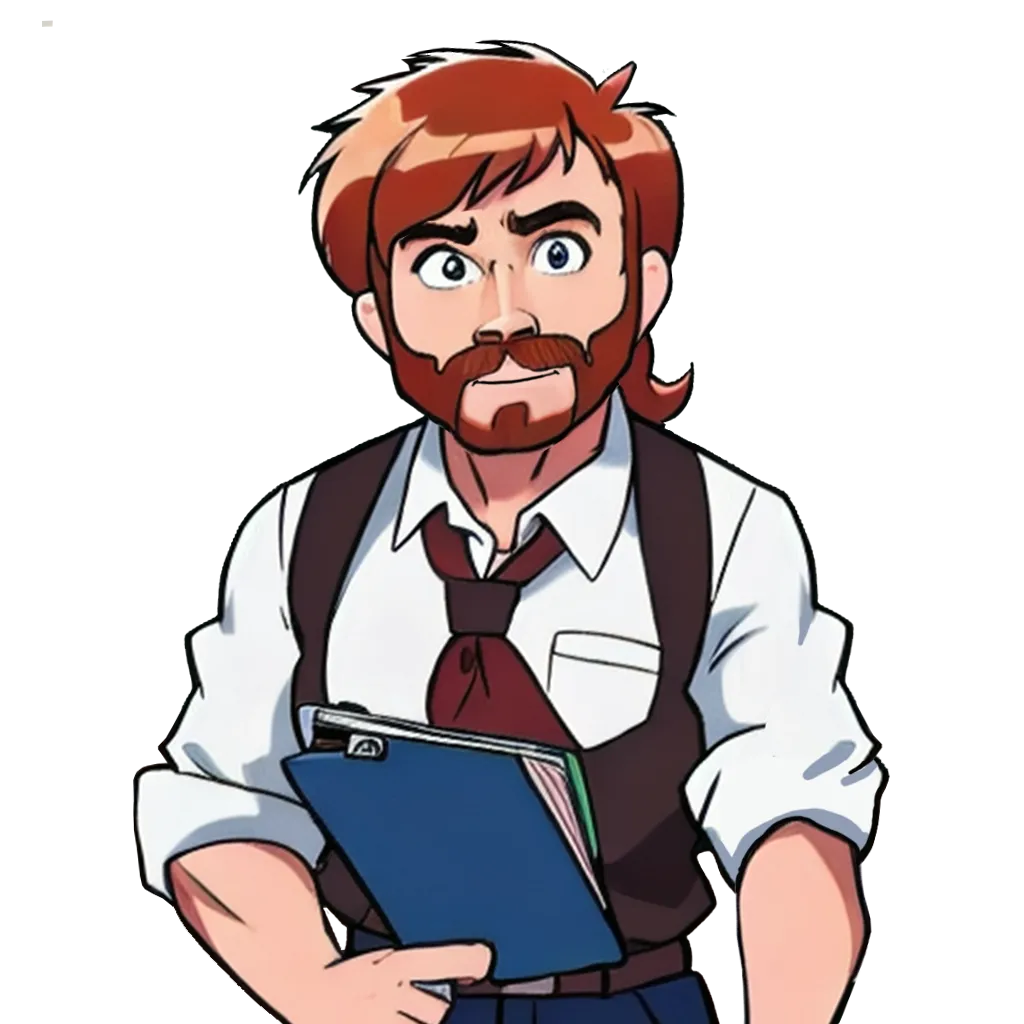