Basic JavaScript
Browser Storage
Browser storage is an important functionality for creating more interactive and efficient web applications. It allows us to store data on the client side, which means we can maintain information between sessions, reduce server requests, and improve user experience. In this chapter, we will explore the different storage mechanisms available in JavaScript, including cookies, Local Storage, Session Storage, and IndexedDB.
Cookies
Cookies are small pieces of data that a server can send to the browser, and the browser stores to send them back in subsequent requests. Cookies are commonly used for session tracking, storing user information, and authentication.
Creating a Cookie
To create a cookie in JavaScript, you can use document.cookie
. Cookies have a key-value format, and you can specify an expiration date and other options.
javascript
Reading Cookies
You can access all cookies using document.cookie
. However, this method returns all cookies as a single string, so you will need to parse it to get individual values.
javascript
Deleting Cookies
To delete a cookie, you can set its expiration date in the past.
javascript
Local Storage
Local Storage allows data to be stored persistently in the browser without expiring. Data stored in Local Storage persists even after the user closes the tab or browser.
Storing Data in Local Storage
You can store data in Local Storage using the setItem
method.
javascript
Reading Data from Local Storage
To read data from Local Storage, use getItem
.
javascript
Removing Data from Local Storage
You can remove a specific item from Local Storage using removeItem
.
javascript
Clearing All Local Storage
If you need to remove all data stored in Local Storage, you can use clear
.
javascript
Session Storage
Session Storage is similar to Local Storage, but the data it stores persists only during the browser session. Once the user closes the tab or browser, the data is deleted.
Storing Data in Session Storage
Like Local Storage, you can store data in Session Storage using setItem
.
javascript
Reading and Removing Data from Session Storage
The methods for reading and removing data in Session Storage are identical to those in Local Storage.
javascript
IndexedDB
IndexedDB is an object-oriented database that allows storing large amounts of structured data, including files and blobs. Unlike Local Storage and Session Storage, IndexedDB is more suitable for complex applications that need to store large data or perform advanced queries.
Opening a Database
To use IndexedDB, you first need to open a database using indexedDB.open
. This process is asynchronous and requires handling success and error events.
javascript
Storing Data in IndexedDB
To store data in IndexedDB, you must create a transaction and use an object store.
javascript
IndexedDB is very powerful, but it is also more complex than Local Storage and Session Storage, so it is mainly used for applications that need more advanced data storage.
Conclusion
Browser storage is an essential tool for creating interactive and efficient web applications. In this chapter, we have explored different client-side storage methods, including cookies, Local Storage, Session Storage, and IndexedDB.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
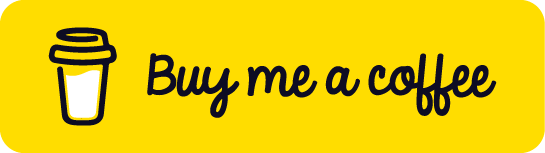
Chat with Chuck
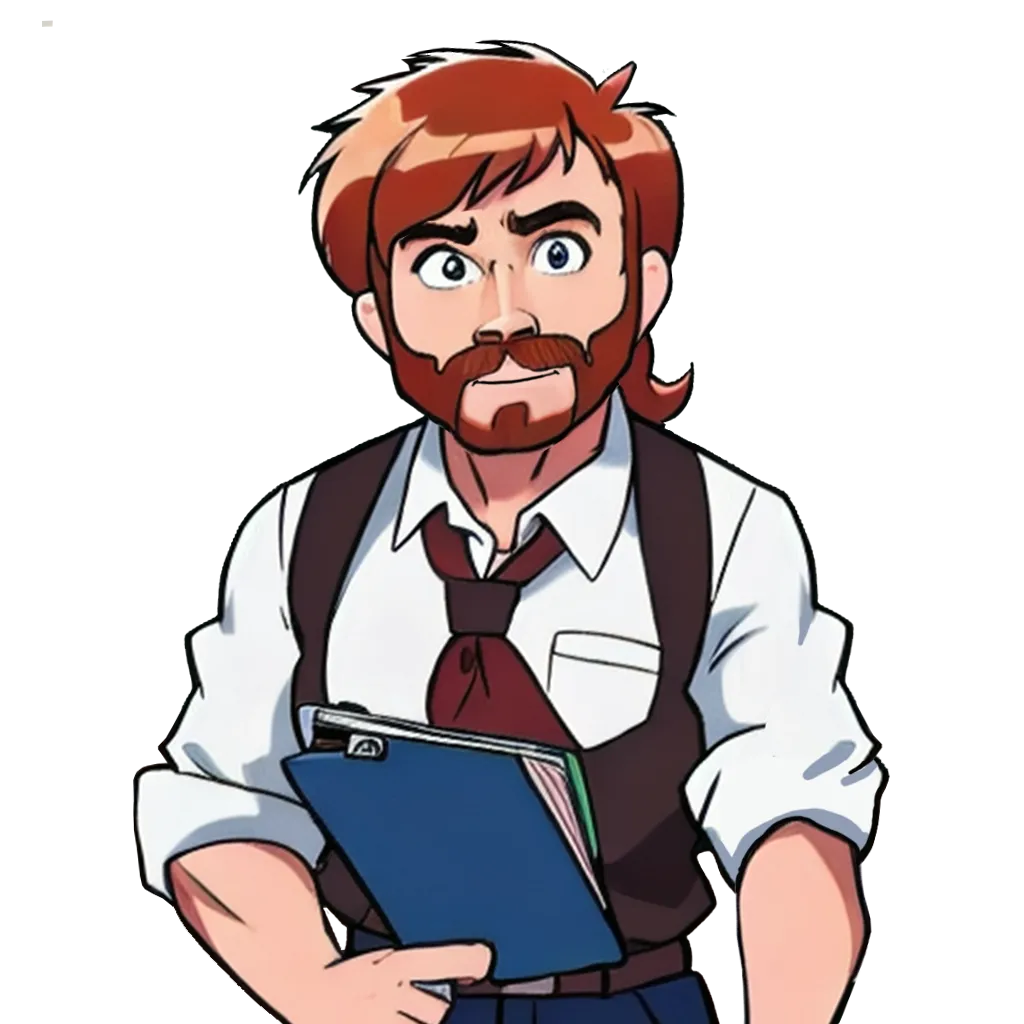
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript