Basic JavaScript
Introduction to JavaScript
JavaScript is one of the most popular and essential programming languages in web development. It was originally created to add interactivity to web pages and, over time, has become one of the key technologies for modern web development. In this chapter, we will explore what JavaScript is, its history, and how it integrates into a website.
What is JavaScript?
JavaScript is an interpreted programming language that runs in the user's browser. Unlike languages like PHP, which run on the server, JavaScript runs on the client side, allowing you to modify the content of a web page without needing to reload it.
Main characteristics of JavaScript:
- It is a high-level language, which means it is easy to understand and write for humans.
- JavaScript is dynamic, allowing you to change the values of variables and objects at runtime.
- JavaScript is multi-paradigm: you can program functionally, object-oriented, or procedurally.
- It is compatible with all modern browsers, making it a crucial tool for web development.
History and Evolution
JavaScript was created in 1995 by Brendan Eich while working at Netscape. Since then, the language has gone through several iterations and has been standardized through the ECMA (European Computer Manufacturers Association) organization.
The JavaScript standard is known as ECMAScript, and the most recent version at the time of writing this course is ECMAScript 2023.
How to Add JavaScript to a Web Page
There are several ways to add JavaScript to a website. The most common is to include JavaScript code directly within an HTML file. Here's a basic example:
html
Other Ways to Integrate JavaScript
In addition to including JavaScript directly in the HTML, you can also link to an external JavaScript file. This is the preferred option when the code is extensive or reused on multiple pages:
html
And the script.js
file could have something like the following:
javascript
Development Tools
To develop and debug JavaScript code, the browser offers various tools that facilitate the developer's work. The most important is the browser console, where you can run JavaScript code in real-time and review error or warning messages.
To open the console in Chrome, for example, you can press Ctrl + Shift + J
(on Windows) or Cmd + Option + J
(on macOS). This will open the development tools, where you can interact with the code.
In addition to the console, you can use specialized text editors like Visual Studio Code or Sublime Text. These editors offer syntax highlighting, autocomplete, and other useful features to write JavaScript code more efficiently.
javascript
Conclusion
JavaScript is a fundamental tool for any front-end developer. Its ability to manipulate web page content in real-time, along with its versatility, makes it an essential language. In this chapter, you have learned what JavaScript is, how it was created, and how you can integrate it into your web pages.
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript
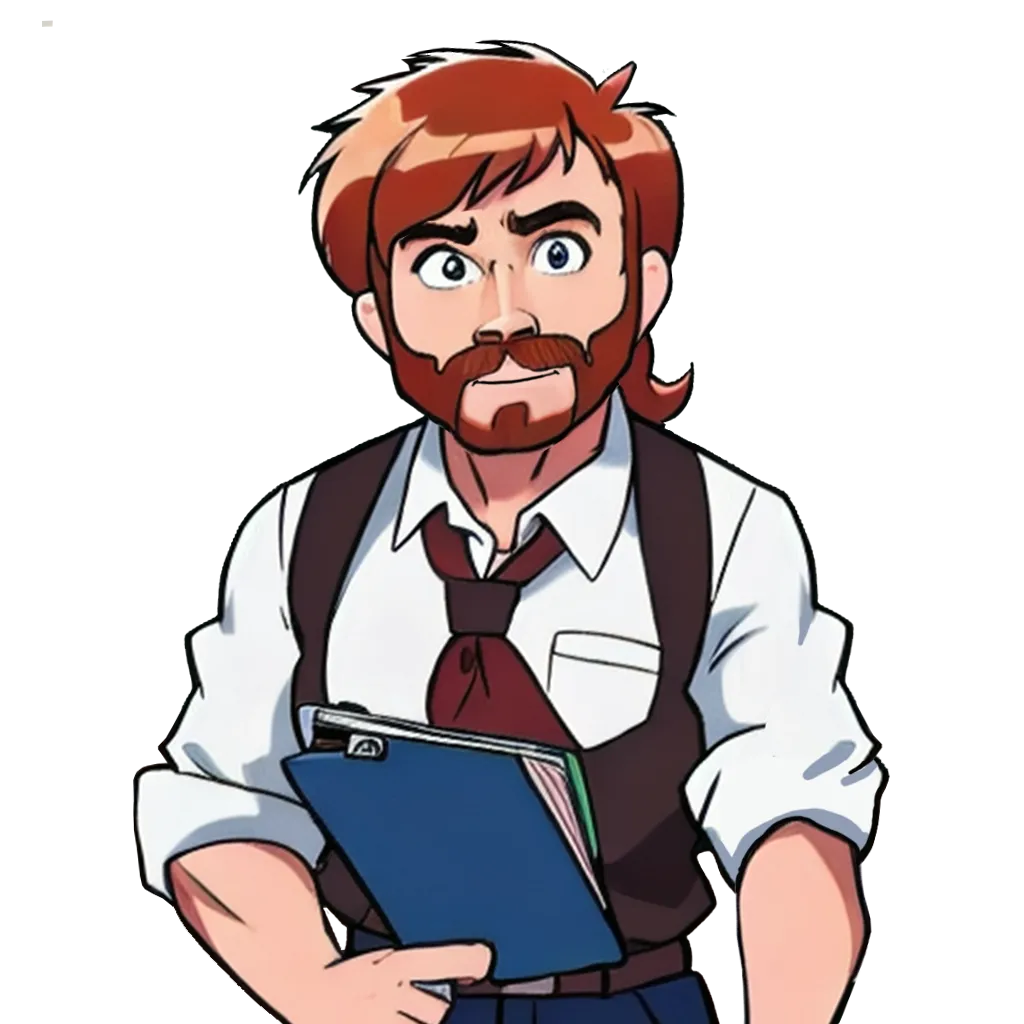