Basic JavaScript
Destructuring in JavaScript
Destructuring is a feature of JavaScript that allows you to extract values from arrays or properties from objects and assign them to variables in a much more concise way. In this chapter, you will learn how to use destructuring to make your code cleaner and easier to read.
Array Destructuring
Array destructuring allows you to assign elements of an array to individual variables concisely.
Basic Example
javascript
Skipping Elements in Destructuring
You can also skip elements when destructuring an array by using commas to indicate which positions to ignore.
javascript
Using Rest Operator
You can use the rest
operator (...
) to group the remaining elements into a single array.
javascript
Object Destructuring
Object destructuring allows you to extract values from an object and assign them to variables with the same name as the properties.
Basic Example
javascript
Assigning New Names to Variables
If you want to assign the object's properties to variables with different names, you can easily do so during destructuring.
javascript
Default Values
You can also assign default values to variables when a property does not exist in the object.
javascript
Nested Object Destructuring
You can perform destructuring on nested objects to extract values from properties within other objects.
javascript
Use in Functions
Destructuring is also useful when working with function parameters. You can receive objects or arrays and destructure them directly in the function parameters.
Destructuring in Function Parameters
javascript
Conclusion
Destructuring in JavaScript is a powerful tool that allows you to write more concise and readable code, especially when working with complex arrays and objects. Leveraging destructuring can make your code easier to maintain and improve its clarity.
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript
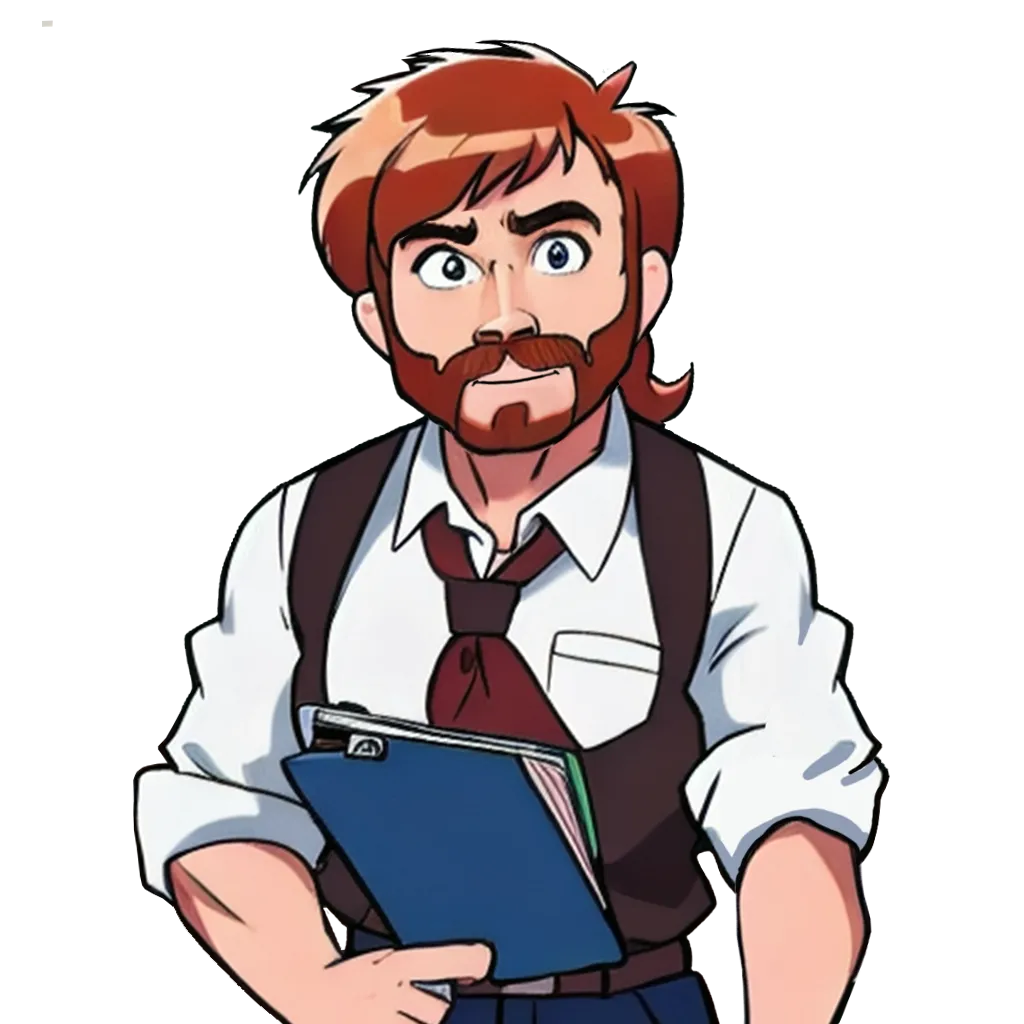