Basic JavaScript
JavaScript Project Deployment
Deploying JavaScript projects involves taking the code you've developed to a production environment so that it is accessible to the public. This process includes optimizing the code, setting up servers, and ensuring that the application works properly in the live environment. In this chapter, we will explore the key steps to deploy a JavaScript application, as well as best practices to ensure a successful deployment.
Deployment Preparation
Before deploying a project, it is important to ensure that the code is optimized and that potential errors have been fixed. Some previous steps include:
- Comprehensive Testing: Make sure that the code has been properly tested with unit tests, integration tests, and E2E (end-to-end) tests.
- Minification and Compilation: Use build tools like Webpack to bundle and minify JavaScript code. This reduces file sizes and improves load times.
- Resource Optimization: Compress images, remove unnecessary files, and ensure that CSS and JavaScript files are minified.
Deployment Options
There are several options for deploying JavaScript projects, depending on the project's needs. The most common options include traditional servers, cloud services, and JavaScript-specific platforms like Netlify or Vercel.
Deployment on Traditional Servers
If you are using a traditional server like Apache or Nginx, the deployment process generally involves:
- Uploading files to the server: You can use tools like FTP or SSH to transfer files to the server.
- Server configuration: Ensure the server is properly configured to serve static files and handle routes.
bash
Deployment on JavaScript Hosting Platforms
Platforms like Netlify and Vercel are optimized for JavaScript projects and provide a simple way to deploy your applications. These platforms offer continuous integration, automatic deployment from Git repositories, and performance optimization.
Deployment with Netlify
Netlify makes it easy to deploy static applications and frontend frameworks like React or Vue.js.
- Create an account on Netlify.
- Connect your Git repository.
- Configure build settings (e.g., specify the output directory, like
/dist
). - Automatically deploy your application.
bash
Deployment with Vercel
Vercel is another popular platform for deploying JavaScript applications and provides support for modern frontend projects.
- Create an account on Vercel.
- Connect your Git repository.
- Configure project settings (Vercel automatically detects frameworks like Next.js).
- Deploy your application with a single click or using the CLI.
bash
Deployment on Cloud Services
Cloud platforms like AWS, Google Cloud Platform, and Azure offer more robust solutions for large-scale projects. These services allow configuring servers, databases, and other necessary resources for more complex applications.
Deployment with AWS S3 and CloudFront
If you are working with a static application, you can easily deploy it on AWS S3 and use CloudFront as a CDN (Content Delivery Network) to improve performance.
- Create a bucket in S3 and upload your project files.
- Configure CloudFront to serve files from the S3 bucket.
bash
Best Practices for Deployment
-
Deployment Automation: Use CI/CD tools like GitHub Actions or CircleCI to automate the deployment process. This ensures that the code is deployed consistently and avoids human errors.
-
Post-Deployment Monitoring: Implement monitoring tools like New Relic or Sentry to track application performance and detect errors after deployment.
-
Environment Variable Management: Ensure that sensitive variables (such as API keys or credentials) are well protected and not part of the public code.
bash
- Using CDNs: Use CDNs to serve static files like images, CSS, and JavaScript. This reduces server load and improves load times for users.
Conclusion
The deployment of JavaScript applications can vary depending on the environment and tools you choose to use. From traditional servers to modern platforms like Netlify and Vercel, there are many options available to ensure that your application is online and accessible.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
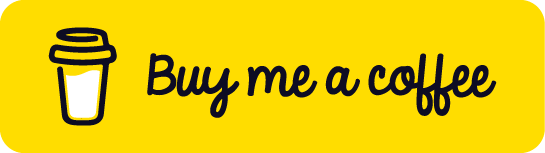
Chat with Chuck
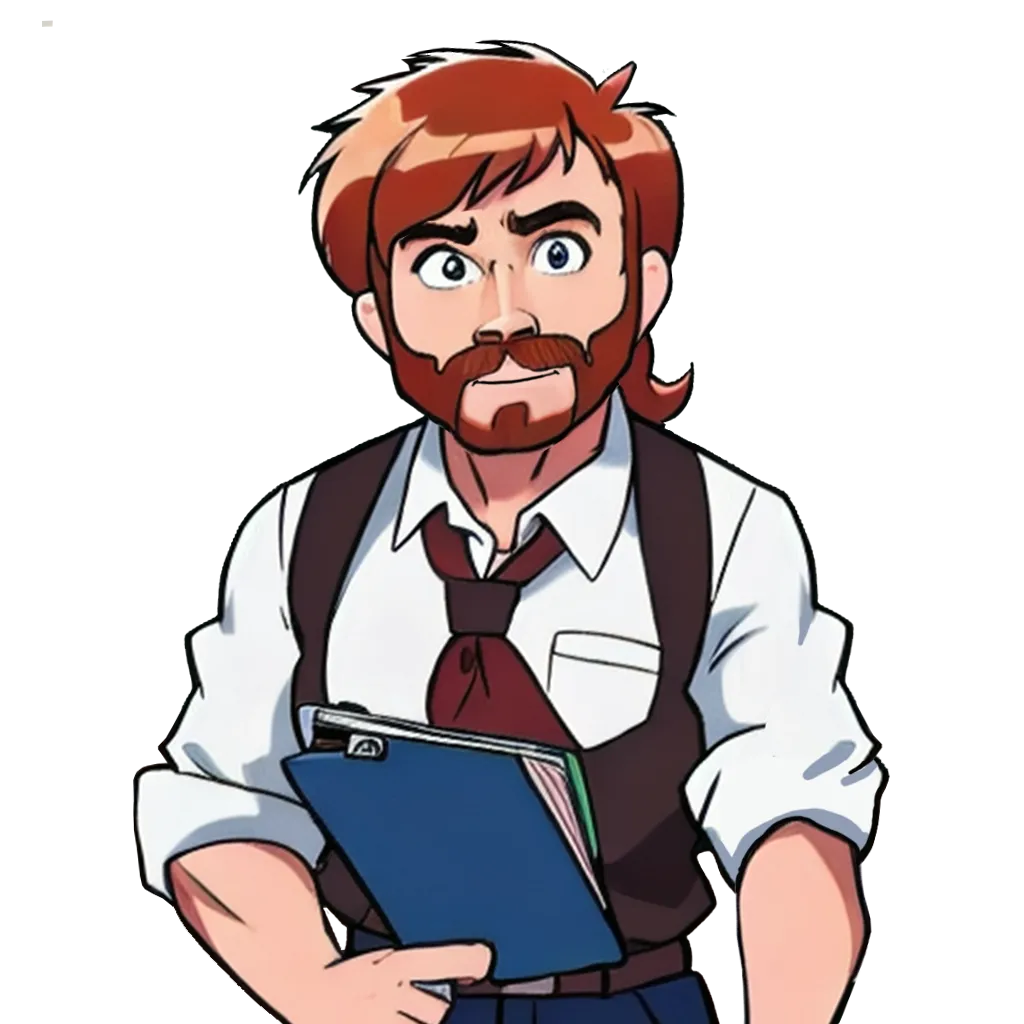
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript