Basic JavaScript
Object-Oriented Programming in JavaScript
Object-Oriented Programming (OOP) is a programming paradigm based on the idea of organizing code into objects. JavaScript supports this paradigm and allows the creation of objects and classes, along with features like inheritance and encapsulation. In this chapter, you will learn the basic concepts of OOP and how to implement them in JavaScript.
Classes and Objects
A class is a blueprint or template from which objects are created. An object is an instance of a class, with its own properties and methods.
Defining a Class
In JavaScript, you can define a class using the class
keyword. Here is an example of a class called Person
:
javascript
Properties and Methods
Properties are variables that belong to an object and methods are functions that belong to an object. In the previous example, name
and age
are properties, and greet
is a method.
Classes as Templates for Objects
Every time you create an instance of a class using the new
keyword, you are creating an object based on that class. Each object can have its own values for the properties.
javascript
Encapsulation
Encapsulation is the concept of restricting direct access to some of the properties and methods of an object to protect the data. In JavaScript, properties can be considered public or private. Although JavaScript doesn't have access modifiers like other languages, it's possible to simulate encapsulation by using underscores to indicate that a property is private, or by using getter and setter functions.
javascript
Inheritance
Inheritance is a key principle in OOP that allows a class to inherit properties and methods from another class. In JavaScript, you can use the extends
keyword to create a class that inherits from another.
javascript
In this example, Dog
inherits the name
property from Animal
, but overrides the speak
method to provide a specific implementation for dogs.
Polymorphism
Polymorphism allows methods from different classes to be called in the same way, although the behavior is different depending on the class. As we saw in the inheritance example, the Dog
class overrides the speak
method from the Animal
class, showing different behavior.
javascript
This is an example of polymorphism, where both Dog
and Cat
have a speak
method, but each class implements it differently.
Abstraction
Abstraction is the concept of hiding complex system details and exposing only what is necessary. In JavaScript, abstraction is achieved by designing classes and methods with clear interfaces, while internal details are encapsulated. There is no direct way to define abstract classes in JavaScript, but you can use base classes to establish interfaces.
javascript
In this example, the Vehicle
class acts as an abstract base class that defines an interface for its subclasses.
Conclusion
Object-Oriented Programming in JavaScript provides a powerful and structured way to organize and manage code. In this chapter, you have learned about classes, objects, encapsulation, inheritance, polymorphism, and abstraction. These techniques will allow you to write more modular and reusable code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
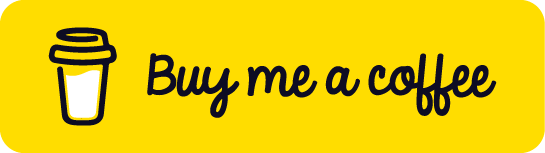
Chat with Chuck
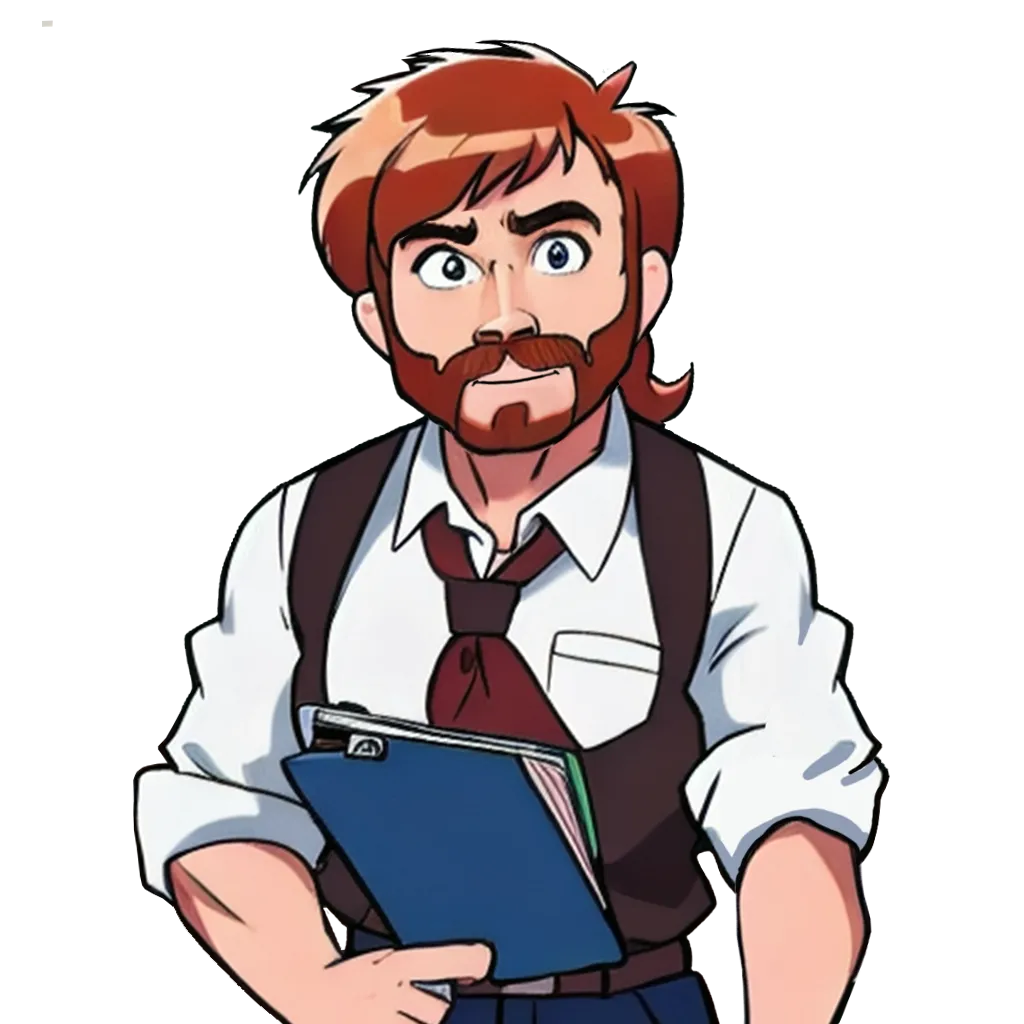
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript