Basic JavaScript
Monitoring and Maintenance of JavaScript Projects
The development process doesn't end with the deployment of an application. Once your JavaScript project is in production, it is essential to monitor its performance and perform continuous maintenance to ensure it keeps running smoothly and meeting user expectations. In this chapter, we'll explore techniques and tools for monitoring and maintenance of JavaScript projects, focusing on error detection, performance analysis, and dependency updates.
Importance of Monitoring
Monitoring provides real-time information about your application's performance and user experience. Implementing a solid monitoring strategy is essential to:
- Detect and fix errors before they impact users.
- Identify performance bottlenecks and optimize the application.
- Improve user experience by monitoring load times, resource usage, and overall application behavior.
Monitoring Tools
1. Sentry
Sentry is a popular platform for real-time error and exception monitoring. It allows you to capture, track, and fix errors in your JavaScript code, providing detailed reports on the application's status.
How to Integrate Sentry into a JavaScript Project
bash
javascript
2. New Relic
New Relic is a monitoring tool that provides insights into application performance, such as response times, memory usage, and server performance. It also includes real-time alerts when performance issues are detected.
3. Google Analytics
Although Google Analytics is commonly used to track web traffic, it can also be used to monitor performance and user behavior in your JavaScript application.
javascript
JavaScript Project Maintenance
Maintaining an application is key to ensuring it continues to operate efficiently and securely in the long term. Some key maintenance areas include:
Updating Dependencies
Over time, libraries and dependencies you use in your project may become outdated or insecure. It's important to keep dependencies updated to ensure compatibility, security, and performance.
Using npm outdated
The npm outdated
command lets you see which dependencies in your project are outdated.
bash
bash
Security Audit Tools
Security vulnerabilities in dependencies can put your application at risk. Tools like npm audit and Snyk help you identify and address known vulnerabilities in your dependencies.
bash
Code Refactoring
Over time, you may find that some parts of the code become difficult to maintain or could be optimized. Refactoring involves rewriting parts of the code to improve readability, efficiency, and maintenance without changing its functionality.
Best Practices for Refactoring
- Avoid code duplication: If you find duplicated code fragments, consider abstracting them into reusable functions or modules.
- Follow SOLID principles: These principles help you write more flexible and maintainable code.
- Use linting and formatting tools: Tools like ESLint and Prettier help maintain consistent and error-free code style.
Performance Monitoring
The speed and performance of an application are key factors influencing user experience. Performance monitoring allows you to identify areas where the application might be running slowly or using too many resources.
Lighthouse
Lighthouse is a tool by Google that analyzes web application performance. It evaluates factors such as load times, CPU usage, and resource efficiency, and provides recommendations to improve performance.
bash
Memory and Resource Usage Analysis
Modern browsers provide developer tools that allow you to perform performance analysis, such as memory allocation and CPU usage tracking.
javascript
Alerts and Notifications
Setting up alerts in your monitoring tools is essential to receive automated notifications when something goes wrong with your application. This can include performance alerts, critical errors, or security vulnerabilities.
Example of Alerts in Sentry
In Sentry, you can configure alerts to notify you via email or Slack when recurring or critical errors are detected.
javascript
Conclusion
Monitoring and maintenance are essential to ensure that your JavaScript project operates efficiently and securely in production. By implementing monitoring tools and following good maintenance practices, you can proactively identify and fix issues.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
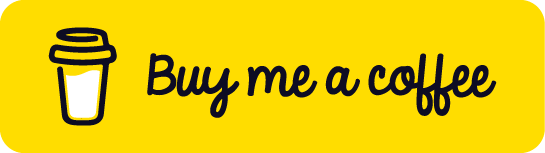
Chat with Chuck
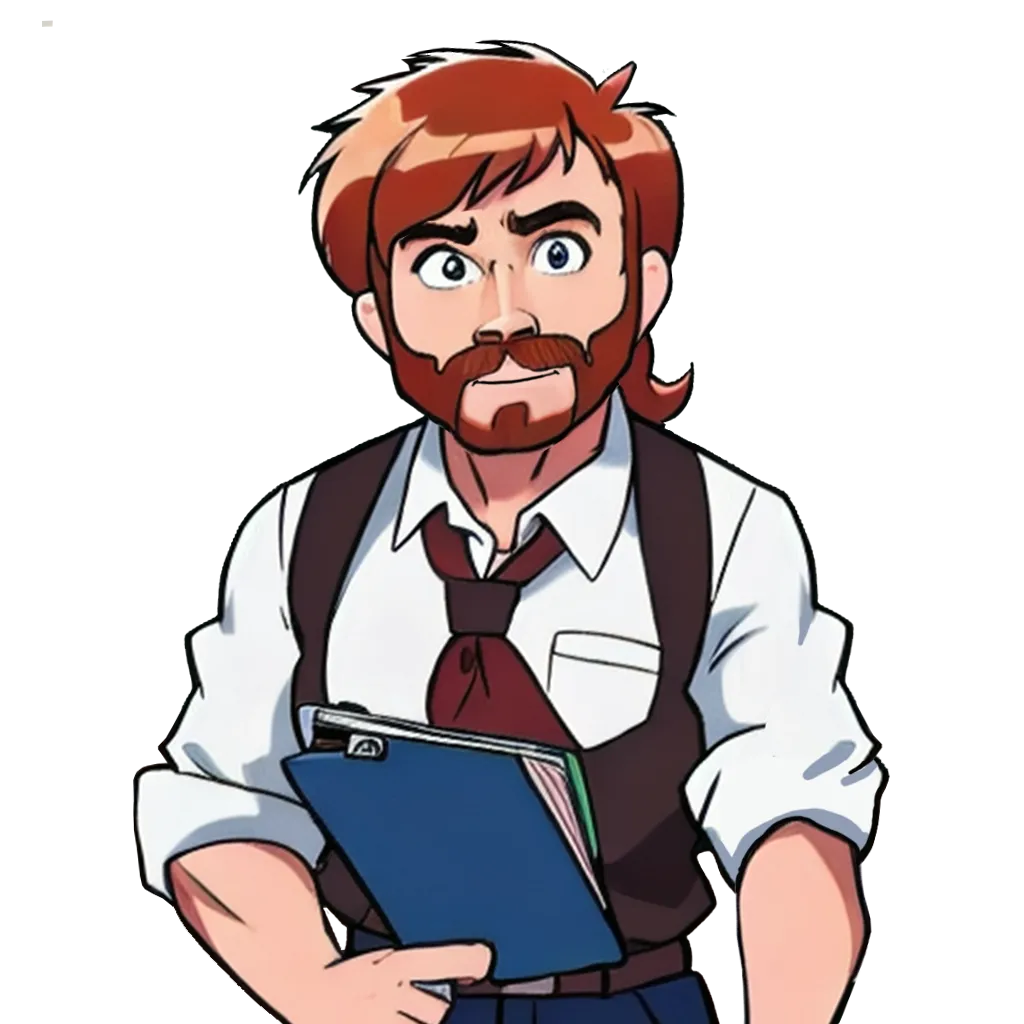
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript