Basic JavaScript
Control Structures
In this chapter, you will learn about control structures in JavaScript. These are fundamental for making decisions in your code and controlling the execution flow. The most common control structures are conditionals and loops, which allow you to execute blocks of code under certain conditions or repeatedly.
Conditionals
Conditional structures allow you to execute different blocks of code based on whether a condition is true or false. The most basic conditional in JavaScript is the if
.
If and Else
The if
statement evaluates a condition. If it is true, the block of code inside the if
is executed. If it is not, you can use an else
block to execute alternative code.
javascript
Else If
The else if
block allows you to evaluate multiple conditions, executing the code block corresponding to the first true condition.
javascript
Switch
Another useful conditional structure is the switch
. This structure compares an expression with various possible values (cases
) and executes the block of code that matches the value.
javascript
Loops
Loops allow you to execute a block of code repeatedly while a condition is satisfied or to iterate over a series of elements. JavaScript offers several types of loops: for
, while
, and do-while
.
For Loop
The for
loop is one of the most common and allows you to iterate a specific number of times. It consists of three parts: initialization, condition, and final expression.
javascript
While Loop
The while
loop repeats a block of code as long as the evaluated condition is true.
javascript
Do-While Loop
The do-while
loop is similar to the while
, but with a key difference: it always executes the code block at least once since the condition is evaluated after the first execution.
javascript
Exception Handling
In JavaScript, you can handle errors and exceptions using try
, catch
, and finally
. This allows you to prevent your application from failing abruptly.
Try and Catch
The try
block contains the code that may produce an error, while the catch
block is executed if an error occurs. In this way, you can safely handle the exception.
javascript
Finally
The finally
block always executes, whether an exception occurs or not. It is useful for releasing resources or performing cleanup tasks.
javascript
Conclusion
Control structures allow you to write code that can make decisions and repeat actions, which is crucial for the logic of any application. In this chapter, we have explored conditional structures and loops, as well as exception handling to avoid unexpected failures.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
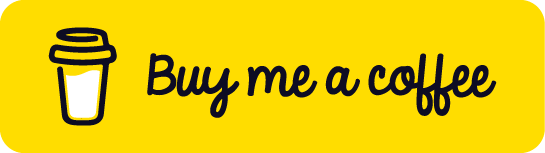
Chat with Chuck
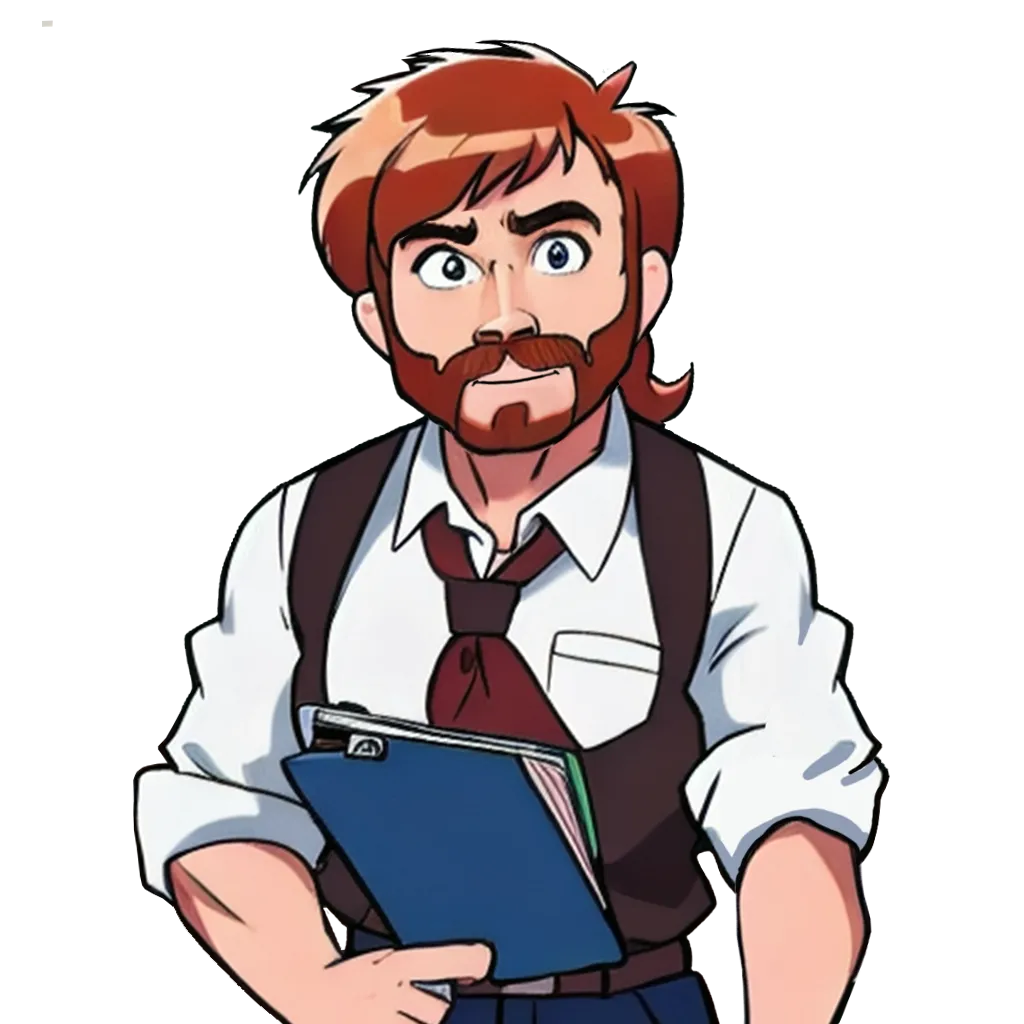
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript