Basic JavaScript
Task Automation and Build Tools
In modern JavaScript development, build tools and task automation have become essential for optimizing workflow and improving web application performance. These tools allow you to compile, minify, bundle, and perform other automated operations that facilitate development and deployment. In this chapter, we will explore some of the most popular tools, how they are used, and when you should integrate them into your project.
What is Task Automation?
Task automation involves using tools that automatically perform repetitive tasks such as:
- Code compilation (for example, using Babel).
- Minification of JavaScript and CSS files.
- Bundling modules into a single file.
- Image optimization.
- Automated testing.
These tasks can be performed manually, but using automation tools reduces human errors and speeds up the development process.
Popular Build and Automation Tools
Webpack
Webpack is a widely used tool for bundling JavaScript modules. Its main function is to transform, combine, and minimize code files, making it a fundamental tool for large projects.
How Webpack Works
Webpack treats everything in your project as a module (JavaScript files, CSS, images, etc.), and then bundles them into one or more "bundles" optimized for loading by browsers.
bash
Basic Webpack Configuration Example
javascript
Babel
Babel is a compiler that allows you to use the latest JavaScript features, such as ES6 or ES7, in browsers that do not yet support these language versions. You can also use it with Webpack to compile your code automatically.
bash
Example of Babel Integration with Webpack
javascript
Gulp
Gulp is a task automation tool that uses workflow streams. You can use Gulp to perform tasks such as file minification, converting Sass to CSS, and automatic browser reload.
bash
Basic Task Example with Gulp
javascript
Minification and Optimization
JavaScript Minification
Minification consists of removing unnecessary characters like white spaces and comments from the code to reduce file size. Webpack and other build tools can do this automatically.
bash
javascript
Image Optimization
Images can take up significant space, so it's important to optimize them to reduce the overall website size. Tools like imagemin can automate this process.
bash
javascript
Live Reload and Watchers
One of the advantages of modern build tools is that they allow the browser to reload automatically when code changes are made. This is possible thanks to watchers that observe file changes and execute necessary tasks.
Automatic Reload Example with Webpack Dev Server
bash
javascript
Conclusion
Task automation and the use of build tools are essential for improving development efficiency and optimizing web applications. Tools like Webpack, Babel, and Gulp simplify the process of code compilation, minification, and optimization, allowing you to focus on developing new features.
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript
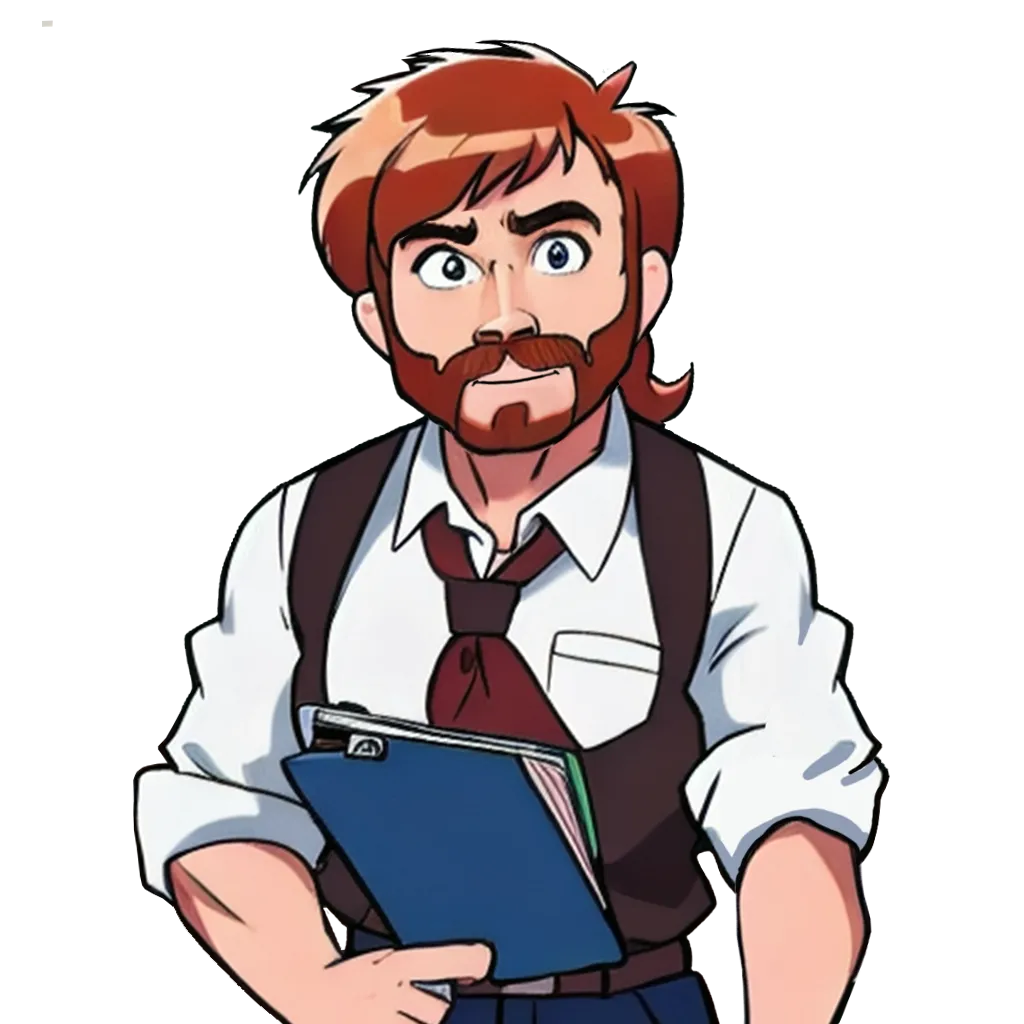