Basic JavaScript
Working with Objects
Objects in JavaScript are one of the most important and flexible data structures. An object is a collection of key-value pairs, where the keys are strings (or Symbols), and the values can be any data type, including other objects, functions, or arrays. This chapter will dive deep into how to create, modify, and work with objects in JavaScript, as well as some edge cases and best practices.
Creating Objects
Object Literal Syntax
The most common way to create an object is by using the object literal syntax:
javascript
Checking for Properties
You might need to check if a property exists in an object before using it. This can be done using the in
operator or the hasOwnProperty
method.
javascript
Useful Object Methods
Object.keys() and Object.values()
JavaScript provides methods like Object.keys()
and Object.values()
to get the keys or the values of an object as arrays.
javascript
Deep Copy of Objects
When copying an object, it is important to understand if you're making a deep copy or a shallow copy. A shallow copy only copies references to other objects. To create a deep copy of an object, you can use JSON.parse
and JSON.stringify
or external libraries like lodash
.
javascript
Conclusion
Objects are the foundation for organizing complex data in JavaScript. As you progress in development, understanding how to work with objects efficiently will help you write cleaner and more maintainable code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
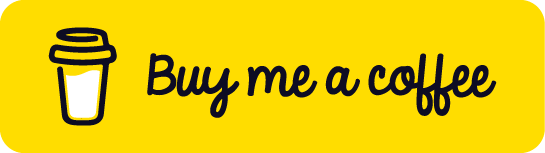
Chat with Chuck
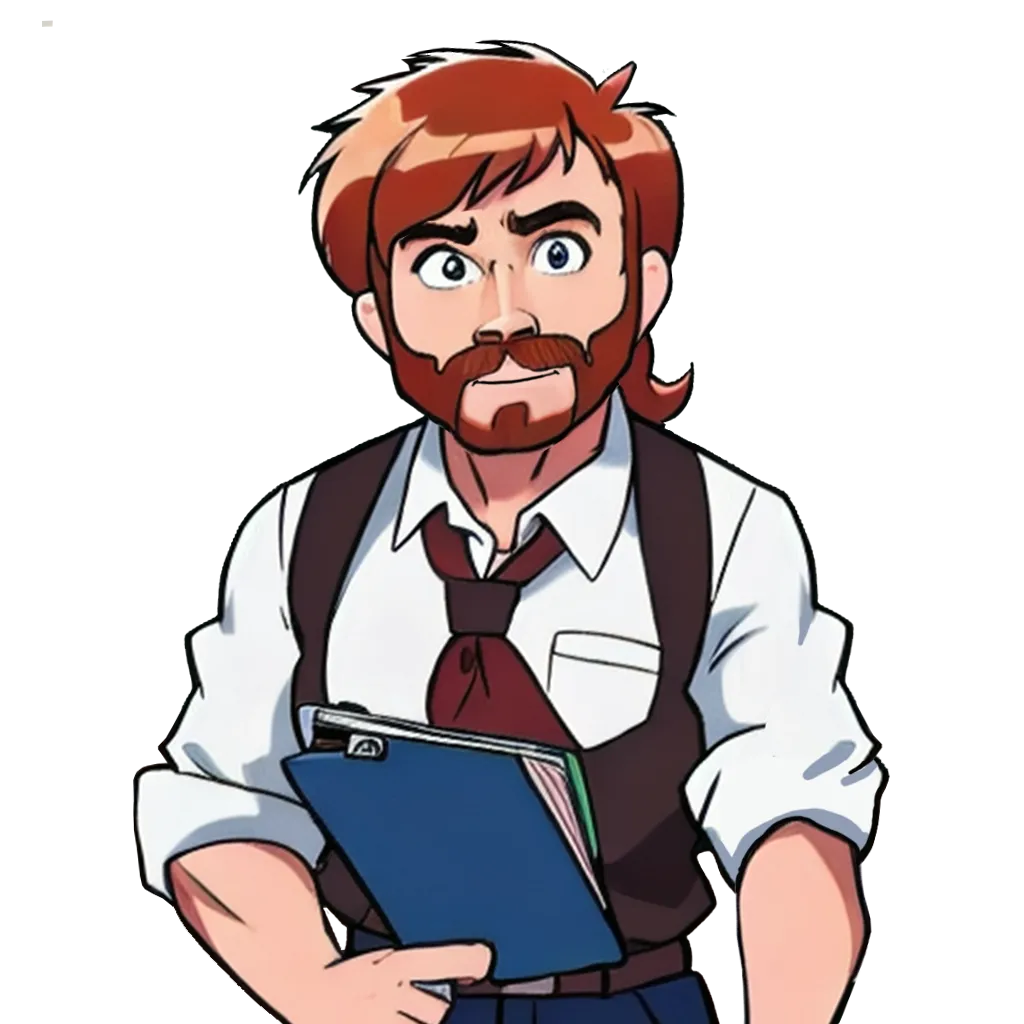
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript