Basic JavaScript
JavaScript Fundamentals
In this chapter, we will cover the essential fundamentals of JavaScript, which will help you understand how this language works at a basic level. We will learn about the language's syntax, the data types you can handle, the variables, and the operators that allow you to perform operations on these data.
Basic Syntax
JavaScript, like other programming languages, has its own syntax, a set of rules that determine how code should be written and structured. Here are some key points of the syntax:
- Each JavaScript statement generally ends with a semicolon
;
, although it is not strictly necessary in many cases. - Braces
{}
are used to group code blocks, for example in functions or control structures. - Single-line comments begin with
//
, while multi-line comments are enclosed between/*
and*/
.
javascript
Data Types and Variables
JavaScript has several data types that you can use to store information. The most common data types are:
- String: for text.
- Number: for numbers (integers and decimals).
- Boolean: for true or false values.
- Null: represents the intentional absence of a value.
- Undefined: a declared variable but with no assigned value.
Variable Declaration
In JavaScript, variables can be declared using the keywords var
, let
, or const
. The main differences are scope and whether the value can change.
javascript
Value Assignment
A variable is assigned a value using the assignment operator =
. The value can be any data type, such as a number or a text string.
javascript
Operators
Operators allow you to perform operations on variables and values. In JavaScript, there are several types of operators:
Arithmetic Operators
These operators are used to perform mathematical operations:
+
Addition-
Subtraction*
Multiplication/
Division
javascript
Comparison Operators
Comparison operators allow you to compare two values. They return true
or false
depending on whether the condition is true.
==
Equality===
Strict equality (checks data type and value)!=
Inequality<
,>
,<=
,>=
Numeric comparisons
javascript
Functions
A function in JavaScript is a block of code designed to perform a specific task. You can define your own functions using the keyword function
, followed by the function name and parentheses ()
.
javascript
You can also create functions that take parameters and return a value:
javascript
Conclusion
In this chapter, we have covered the foundational concepts of JavaScript, from its basic syntax to the use of variables, data types, operators, and functions. These are the pillars upon which your JavaScript knowledge will be built."
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
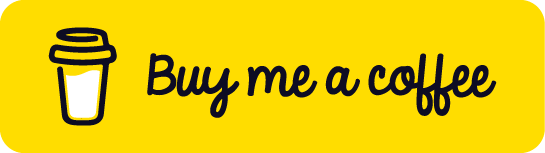
Chat with Chuck
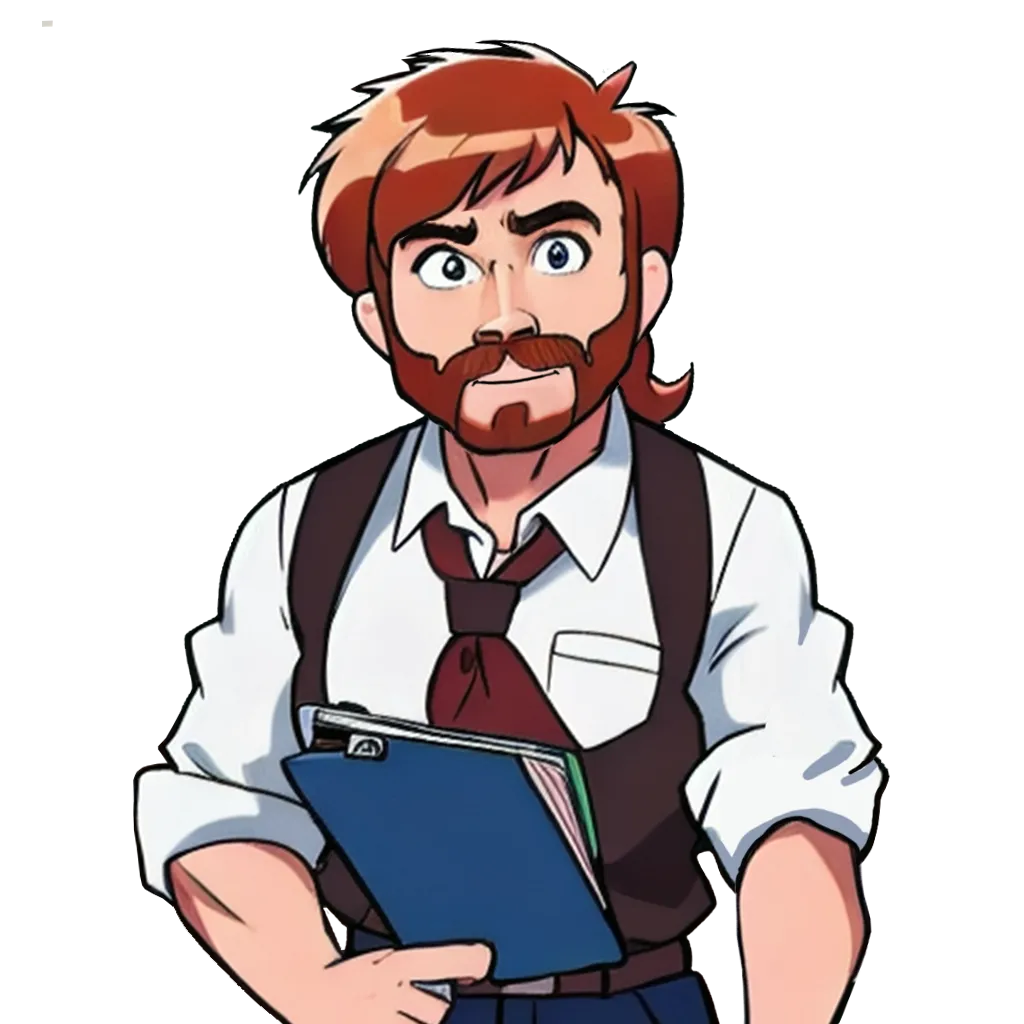
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript