Basic JavaScript
Asynchrony in JavaScript
Asynchrony is one of the most important concepts in JavaScript because it allows the language to handle operations that take time to complete, such as server requests, file handling, or timers. In this chapter, we will explore how JavaScript handles asynchrony and how you can write asynchronous code using callbacks, promises, and the async/await
syntax.
The JavaScript Execution Model
JavaScript uses a single-threaded execution model, which means it can only execute one thing at a time. However, to handle multiple tasks that cannot be completed immediately, such as a network request, JavaScript uses a mechanism known as the event loop. This allows code to keep executing while waiting for the response of an asynchronous operation.
Callbacks
A callback is a function that is passed as an argument to another function and is executed once a task is completed. It is one of the oldest and most basic ways to handle asynchrony in JavaScript.
Callback Example
javascript
Callbacks are useful, but when you have multiple asynchronous operations chained together, they can lead to what is known as callback hell, where the code becomes difficult to read and maintain.
Promises
To avoid callback hell, JavaScript introduced promises. A promise is an object that represents the eventual completion or failure of an asynchronous operation. Promises can be in one of three states:
- Pending: The promise has not yet been resolved or rejected.
- Fulfilled: The operation completed successfully.
- Rejected: The operation failed.
Promise Example
javascript
Promises are a significant improvement over callbacks as they provide a clearer way to handle asynchronous operations, especially when combining multiple promises.
Promise Chaining
One of the great advantages of promises is that you can chain them to handle multiple asynchronous operations sequentially.
javascript
Async and Await
Although promises improve the readability of asynchronous code, the introduction of async
and await
in ES2017 simplified them even further. async/await
allows you to write asynchronous code that looks a lot like synchronous code, making it easier to read and understand.
Async and Await Functions
A function marked with async
always returns a promise. Inside an async
function, you can use the await
keyword to wait for the resolution of a promise before proceeding to the next step.
javascript
The use of async/await
is generally preferred for its simplicity and readability, especially when you have multiple asynchronous operations that need to be executed in sequence or in parallel.
Parallel Execution with Promise.all
If you have several promises that can run in parallel, you can use Promise.all
to wait for all of them to resolve before proceeding.
javascript
Conclusion
Asynchrony is a key aspect of JavaScript and is fundamental for creating efficient and responsive web applications. In this chapter, we have explored how to handle asynchrony using callbacks, promises, and the async/await
syntax.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
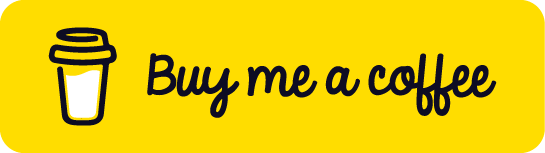
Chat with Chuck
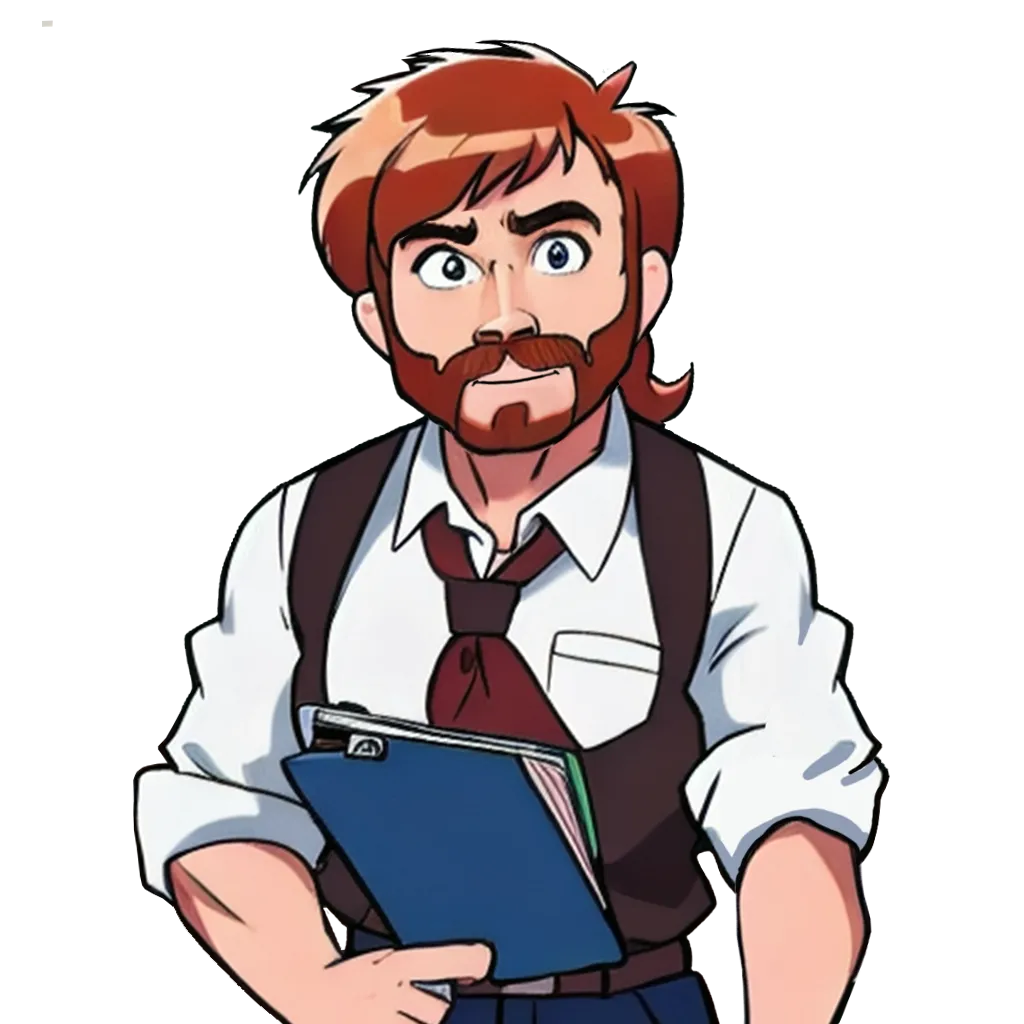
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript