Basic JavaScript
Modularization and Dependency Management
As JavaScript applications become larger and more complex, it becomes essential to organize the code efficiently. Modularization and dependency management allow you to divide the code into reusable and manageable parts, making it easier to maintain and scale. In this chapter, you will learn the concepts of modularization in JavaScript and how to efficiently manage dependencies using modern tools.
What is Modularization?
Modularization is the process of dividing a program into smaller modules, each with a specific responsibility. This helps improve code readability, reusability, and maintainability.
Modules in JavaScript (ES6)
Since ES6, JavaScript has native support for modules. This allows exporting and importing functions, objects, or values between JavaScript files.
Exporting and Importing Modules
Export a Module
You can export functions, variables, or classes using the export
keyword. There are two types of exports: named export and default export.
javascript
Import a Module
Once you have exported a module, you can import it into another file using the import
keyword.
javascript
Default Export
The default export allows exporting a single default value per file. It's useful when you want to export a single main function or class from a file.
javascript
Advantages of Modularization
- Maintainability: Modular code is easier to maintain because each module has a clear responsibility.
- Reusability: Modules can be reused in different parts of the application or even in other projects.
- Scalability: Modularization allows applications to grow without becoming an unmanageable tangle of code.
Dependency Management
As projects grow, it's common to use external libraries or third-party modules. Dependency management refers to the process of managing these libraries and ensuring they are available and up-to-date in your project.
NPM (Node Package Manager)
NPM is the most widely used package manager in the JavaScript ecosystem. It allows you to install, update, and manage external dependencies for your project.
Initialize a Project with NPM
The first step to using NPM is to initialize a project with the npm init
command, which generates a package.json
file containing information about the project and its dependencies.
bash
Install Dependencies
To install a library or package in your project, you can use the npm install
command. This will add the library to the node_modules
folder and update the package.json
file.
bash
Dependency Versions
NPM allows you to specify exact versions or version ranges for dependencies. This ensures that your project uses compatible versions and does not suffer from errors due to unexpected updates.
json
Yarn
Yarn is an alternative to NPM that is also used to manage dependencies in JavaScript projects. It offers similar features to NPM but with a focus on speed and more precise determination of dependency versions.
bash
Common Packages in JavaScript Projects
Some common packages you can use to enhance your workflow in JavaScript projects include:
- webpack: A module bundler that allows compiling JavaScript and other files into a single bundle to improve application performance.
- Babel: A JavaScript compiler that lets you use the latest language features in older browsers.
- ESLint: A tool for detecting and fixing problems in JavaScript code, ensuring it follows best practices.
bash
Conclusion
Modularization and dependency management are fundamental to keeping your code organized and efficient in modern JavaScript applications. Using tools like NPM and Webpack, you can divide your code into reusable parts and effectively manage external libraries.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
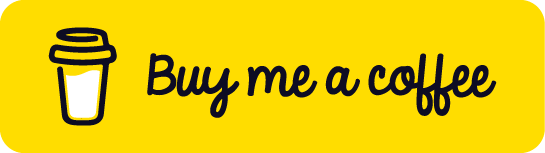
Chat with Chuck
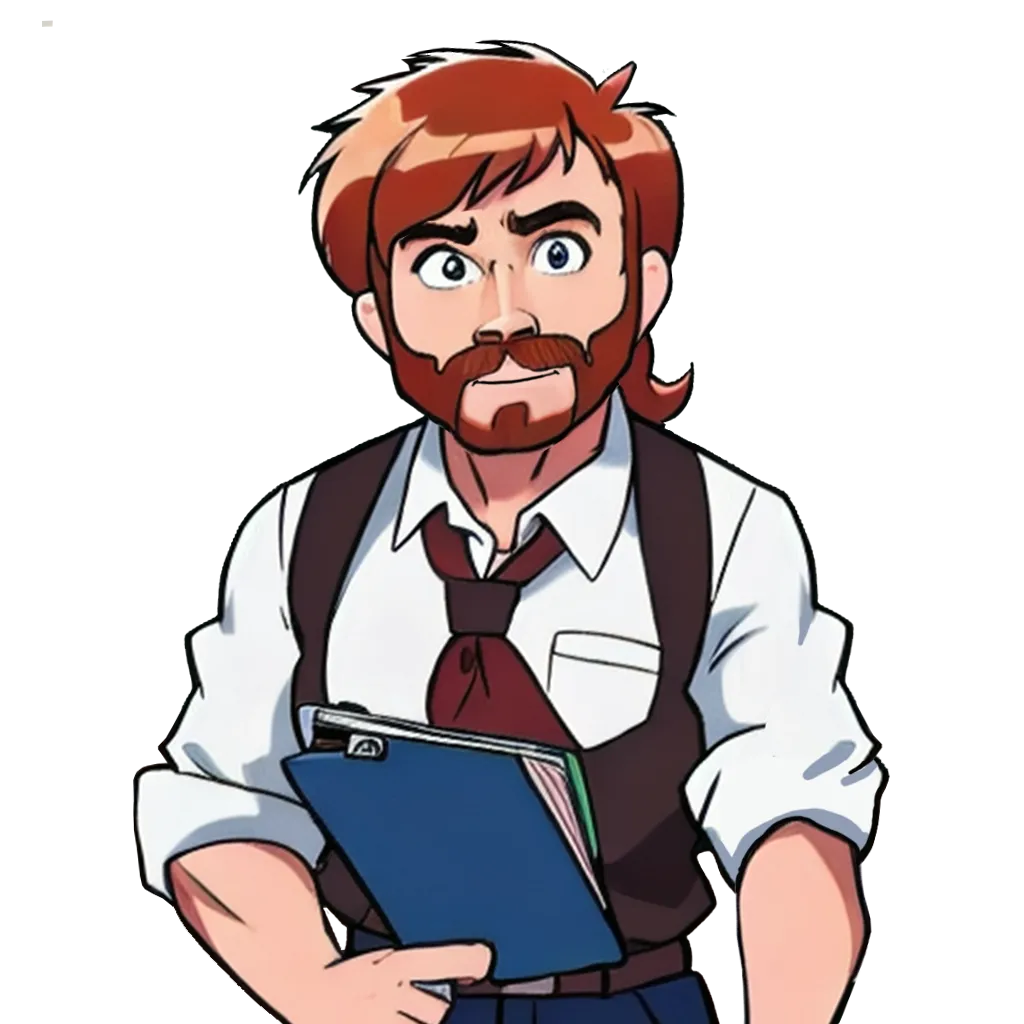
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript