Basic JavaScript
Introduction to Frameworks and Libraries
As web applications become more complex, writing all the code from scratch using only JavaScript can become complicated and inefficient. To help developers build applications more quickly and structurally, there are frameworks and libraries that make the work easier. In this chapter, we will provide you with an introduction to some of the most popular frameworks and libraries in the JavaScript ecosystem, and when it is appropriate to use them.
What is a Framework?
A framework is a set of predefined tools and structures that provides a solid foundation for developing applications. Frameworks typically include rules, structures, and conventions that guide how you organize your code and develop your applications.
Examples of Popular Frameworks
-
React (Although technically a library, it acts as a framework):
- Developed by Facebook, it's one of the most used tools to build user interfaces.
- Facilitates the development of interactive and reactive web applications through reusable components.
-
Vue.js:
- A progressive framework that is easy to learn and use.
- Has a gentle learning curve and is ideal for both small projects and larger applications.
-
Angular:
- Developed by Google, it's a complete framework for developing robust web applications.
- Includes features like dependency injection, advanced templating, and routing management.
What is a Library?
A library is a set of functions and tools that you can use to perform specific tasks within your application. Unlike frameworks, libraries do not impose a structure on how you should organize your application. You choose when and how to use them.
Examples of Popular Libraries
-
Lodash:
- A utility library that makes working with arrays, objects, and other data structures easier.
- Offers methods to manipulate and transform data more efficiently.
-
Axios:
- A popular library for making HTTP requests.
- Simplifies handling requests to APIs and servers and supports promises.
-
D3.js:
- Powerful library for creating graphs and data visualizations using HTML, SVG, and CSS.
- Ideal for creating interactive graphs and complex visualizations on the web.
javascript
When to Use a Framework or Library?
Deciding whether to use a framework or a library depends on the complexity of your project and your specific needs. Here are some cases where you might opt for one or the other:
- Use a Framework if your project requires a well-defined structure, is a large application that will grow over time, or if you need many integrated features like routing and state management.
- Use Libraries if your project is smaller or if you only need a specific solution for a task (like data manipulation or making HTTP requests).
Why You Shouldn't Always Use Frameworks?
While frameworks are extremely useful, they are not always necessary. If you are developing a simple web page or a small project, adding a full framework can add unnecessary complexity. Sometimes, the "vanilla JavaScript" approach (without libraries or frameworks) is sufficient and more efficient.
Framework Comparison
React vs Vue.js vs Angular
-
React:
- Moderate learning curve.
- Highly flexible but requires additional configuration for things like routing and state management.
-
Vue.js:
- Smooth learning curve, ideal for beginners.
- Provides a lighter and simpler structure compared to Angular.
-
Angular:
- Steeper learning curve.
- Provides a comprehensive solution ready for large enterprise applications.
Conclusion
Frameworks and libraries in JavaScript are powerful tools that allow you to build applications more quickly and with fewer errors. In this chapter, we have seen some of the most popular ones and when it is appropriate to use them.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
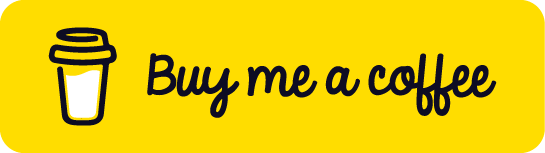
Chat with Chuck
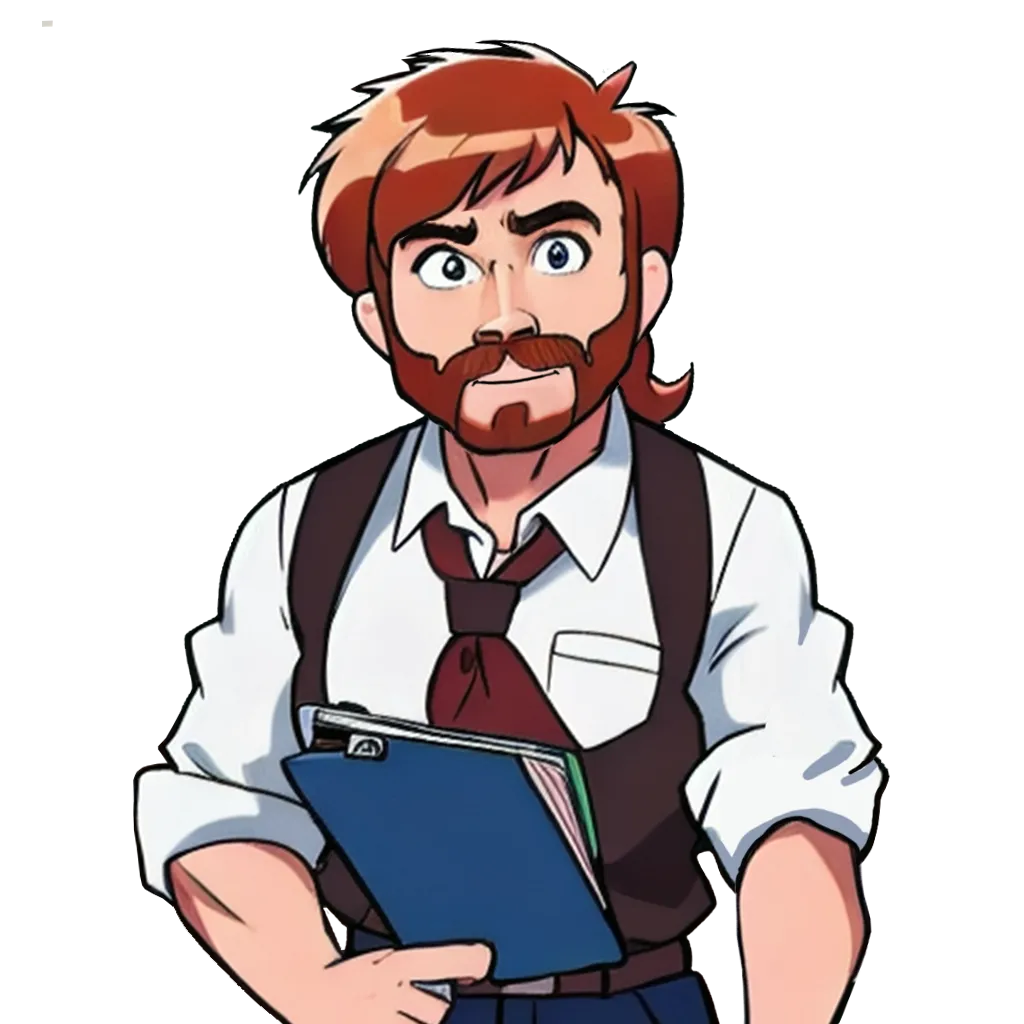
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript