Basic JavaScript
Best Practices and Optimizations
Writing clean, efficient, and maintainable JavaScript code is crucial for developing scalable applications. In this chapter, we will cover some of the best practices and optimizations that will help you write better code, improve performance, and ensure readability.
Writing Clean Code
Use Descriptive Names
One key principle of writing clean code is to use descriptive names for variables, functions, and classes. A clear and descriptive name makes it easier to understand the code without having to read every line.
javascript
Avoid Unnecessary Comments
Comments are useful when they explain the intention of the code, but they should be avoided if they only describe what is already evident. The goal is for the code to be self-explanatory.
javascript
Performance Optimization
Avoid Direct DOM Manipulation
Manipulating the DOM is costly in terms of performance, especially when many modifications are made. It is preferable to make modifications in a document fragment and then insert the fragment into the DOM all at once.
javascript
Avoid Reflows and Repaints
Reflow occurs when the browser recalculates the positions and dimensions of elements on the page, while repaint occurs when changes in the DOM affect the visual appearance. To minimize these effects, avoid requesting computed styles repeatedly inside loops.
javascript
Avoid Inefficient Loops
When working with large amounts of data, inefficient loops can significantly impact performance. Using methods like map
, filter
, and reduce
is preferable over traditional loops because they are more declarative and improve readability.
javascript
Using Promises and async/await
When working with asynchronous operations, it is important to correctly use promises or the async/await
syntax to avoid the so-called callback hell, which can make the code difficult to follow.
Use async/await instead of Promise chaining
javascript
Avoid Using Global Variables
Global variables can cause naming conflicts and unpredictable behavior in code. It is better to limit the scope of variables using let
or const
instead of var
.
javascript
Optimization Tools
Code Minification
Minification is the process of removing whitespace, comments, and reducing variable names to make the code smaller and therefore faster to load. Tools like UglifyJS
or Terser
can help you minify your code.
Lazy Loading
Lazy loading allows resources to be loaded only when needed, instead of loading them all at the start. This can significantly improve performance, especially on pages with many resources.
javascript
Conclusion
Best practices and optimizations are essential to ensure that your JavaScript code is efficient, maintainable, and scalable. By applying these principles in your daily work, you will improve both the quality of your code and the end-user experience.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
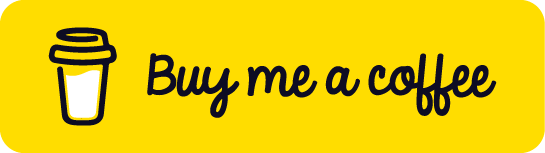
Chat with Chuck
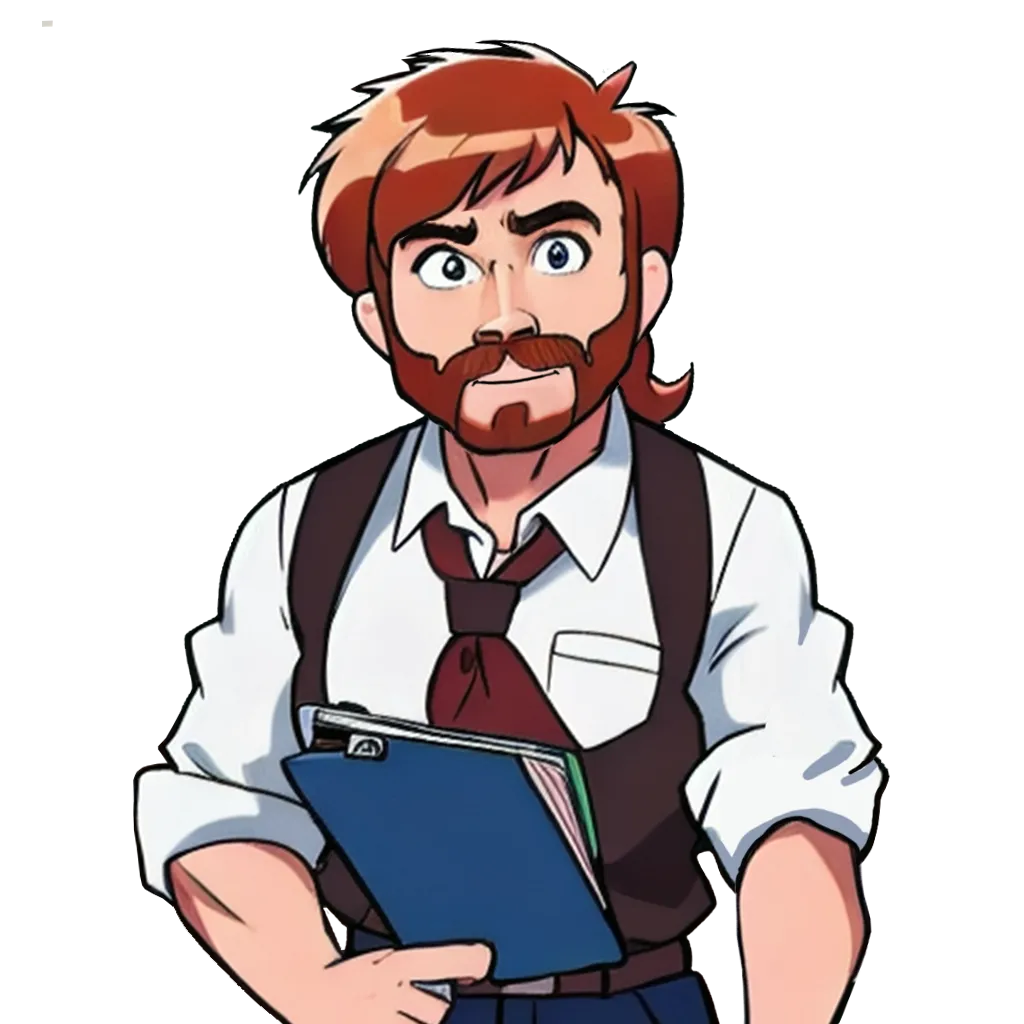
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript