Basic JavaScript
Working with Arrays
Arrays are a fundamental data structure in JavaScript that allows you to store a list of elements, which can be of any type. This chapter covers the basic concepts of arrays and some advanced techniques to work with them efficiently.
Creating Arrays
Array Literal Syntax
The most common way to create an array is using brackets []
:
javascript
Arrays with the Constructor
Another way to create an array is using the Array
constructor.
javascript
Common Array Methods
slice() and splice()
The slice()
method returns a shallow copy of a portion of an array, whereas splice()
can modify the contents of an array by removing or adding elements.
javascript
Mutable vs. Immutable
Some operations on arrays are mutable (they modify the original array), while others are immutable (they return a new array). For example, push
is mutable, whereas concat
is immutable.
javascript
Conclusion
Arrays in JavaScript are extremely flexible and offer a wide range of methods to handle data collections.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
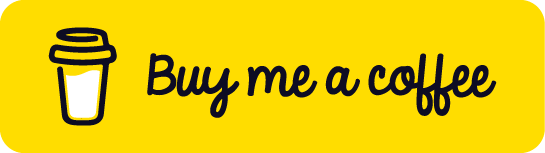
Chat with Chuck
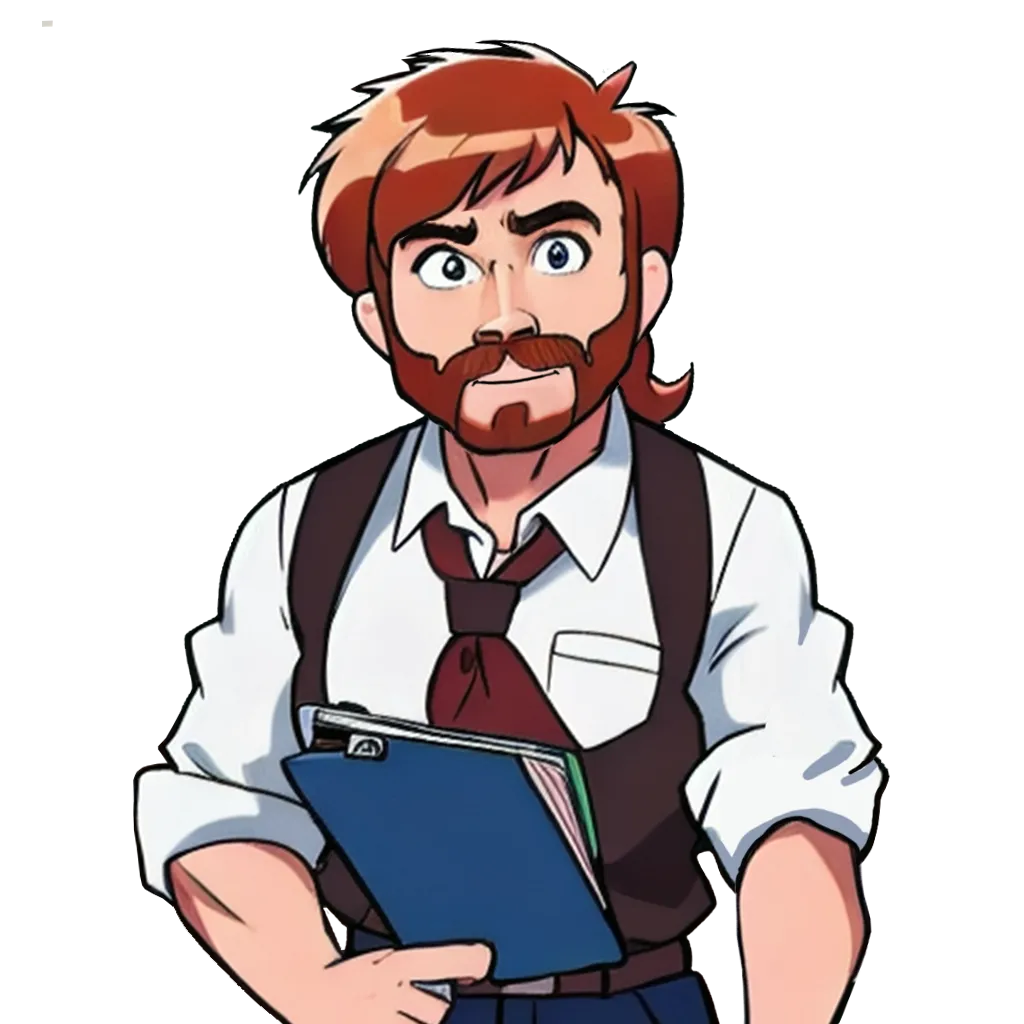
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript