Basic JavaScript
Filter, reduce, and other functions
Functions like filter
, reduce
, some
, every
, and others allow you to perform complex and advanced operations on arrays. In this chapter, you will learn how to use them effectively to handle data, with practical examples and performance considerations.
filter
The filter
method creates a new array with all the elements that pass a specific test.
Example of filter
javascript
Using filter on Object Arrays
You can use filter
on object arrays to create subsets based on specific conditions.
javascript
reduce
The reduce
method applies a function to an accumulator and each element of the array to reduce it to a single value. It is useful for calculating values like sums, products, or even more complex data structures.
Example of reduce
javascript
Reduce to Create Objects
The reduce
method can also be used to transform arrays into objects.
javascript
some and every
The methods some
and every
allow you to check if some or all the elements of an array meet a condition.
Example of some
javascript
Example of every
javascript
Conclusion
The functions filter
, reduce
, some
, and every
are powerful tools for performing advanced operations on arrays in JavaScript. They allow you to manipulate and transform data efficiently, improving the clarity and performance of your code. These functions are fundamental for working with complex data in modern web applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
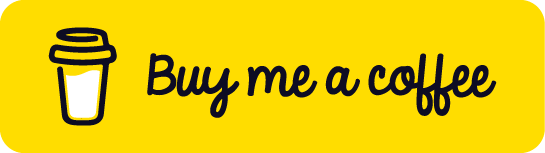
Chat with Chuck
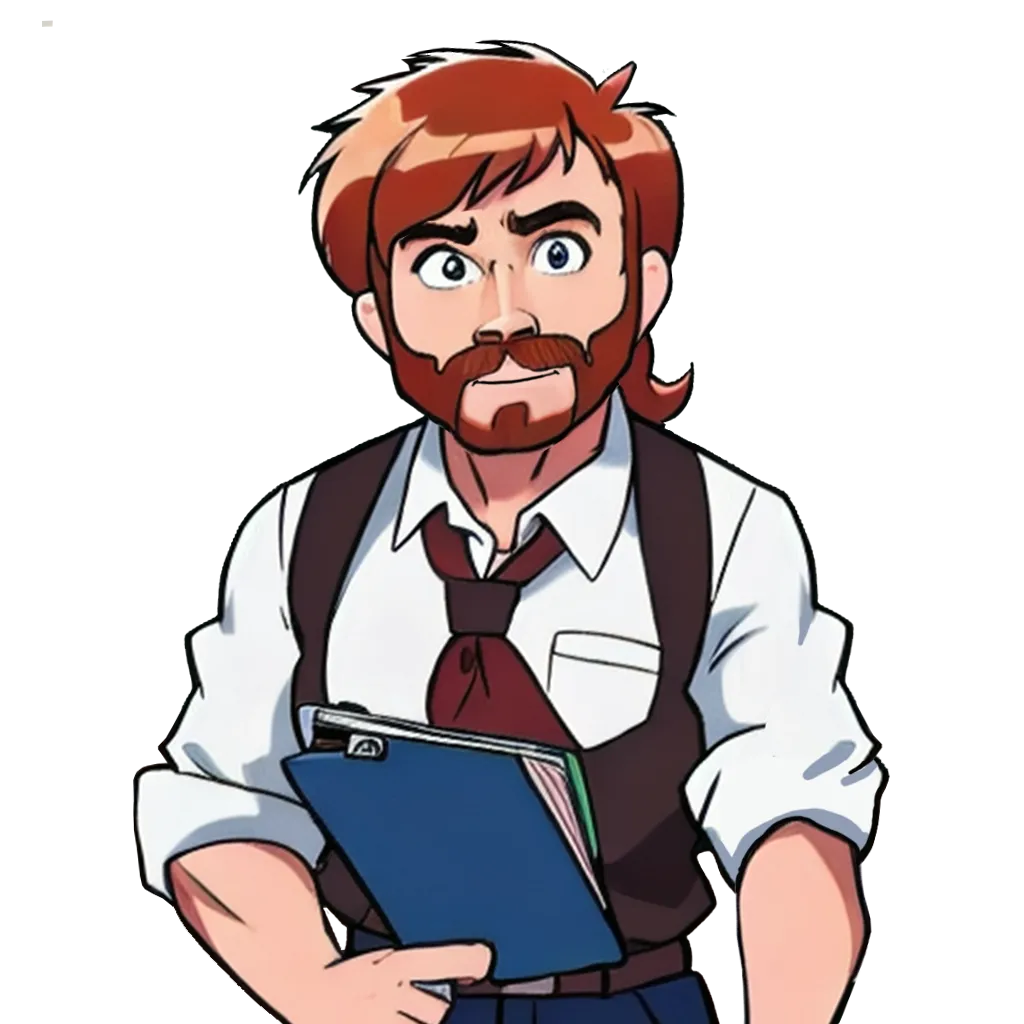
- Introduction to JavaScript
- JavaScript Fundamentals
- Control Structures
- Working with Objects
- Working with Arrays
- Destructuring in JavaScript
- Functions forEach and map
- Filter, reduce, and other functions
- DOM Manipulation
- Advanced Functions
- Object-Oriented Programming in JavaScript
- Asynchrony in JavaScript
- Working with APIs
- Browser Storage
- Handling Dates and Times in JavaScript
- Best Practices and Optimizations
- Introduction to Frameworks and Libraries
- Testing in JavaScript
- Modularization and Dependency Management
- Task Automation and Build Tools
- JavaScript Project Deployment
- Monitoring and Maintenance of JavaScript Projects
- Course Conclusion of JavaScript