Basic React
Handling APIs and Fetch in React
Handling external data through APIs is a common task in modern web applications. In React, you can use the fetch
function or libraries such as Axios to make HTTP requests to APIs and retrieve data to integrate into your components. In this chapter, we will learn to handle the lifecycle of asynchronous requests using fetch, manage errors, and how to update your application's state in response to retrieved data.
Introduction to fetch
fetch
is a native JavaScript API that allows you to make HTTP requests from the browser. It's simple to use and promise-compatible, making it easier to handle asynchronous operations.
Basic Example of fetch
Here is a basic example of how to use fetch
to make a GET request to an API and display the data in a React component:
jsx
In this example, the useEffect
hook makes the API request when the component mounts. If the request is successful, the data is stored in the state and displayed. If there's an error, it's handled by showing an error message.
Error Handling
It's important to handle errors correctly when working with fetch
. An error can occur if the network fails or if the API returns a response with an HTTP error code. In the previous example, we handled both types of errors: network issues and non-ok
responses.
Loading State Management
It's good practice to display a loading indicator (like a "Loading..." message) while waiting for API data to be available. In the previous example, the loading
state is used to manage this indicator.
Using POST
and Other HTTP Methods
In addition to GET
requests, you might need to send data to an API using the POST
method. Here's an example of how to make a POST
request with fetch
:
jsx
In this example, we use the POST
method to send data to the API. The request body is defined as a JSON string, and the HTTP headers are set to indicate that JSON content is being sent.
Using External Libraries: Axios
Although fetch
is a very powerful native API, libraries like Axios offer simpler syntax and additional features like request cancellation and automatic JSON response configuration. You can install Axios with the following command:
bash
Here is an example of how to make a request with Axios:
jsx
Axios simplifies error handling and JSON response parsing, making it a good choice for projects requiring more functionality.
Performance Considerations and Best Practices
When making API requests, it's important to consider performance and user experience:
-
Avoid Unnecessary Requests: Ensure that API requests are made only when necessary. You can use dependencies in
useEffect
to control when a request should be made. -
Caching Handling: To improve performance, consider implementing a caching system to avoid making unnecessary repeated API requests.
-
Pending Request Control: In cases where the component might unmount before the request is complete, use techniques to cancel pending requests with
AbortController
(forfetch
) or Axios's cancel function.
Conclusion
Handling APIs in React is essential for most modern applications. Whether you use fetch
or an external library like Axios, it's important to properly handle loading states, errors, and API responses.
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps
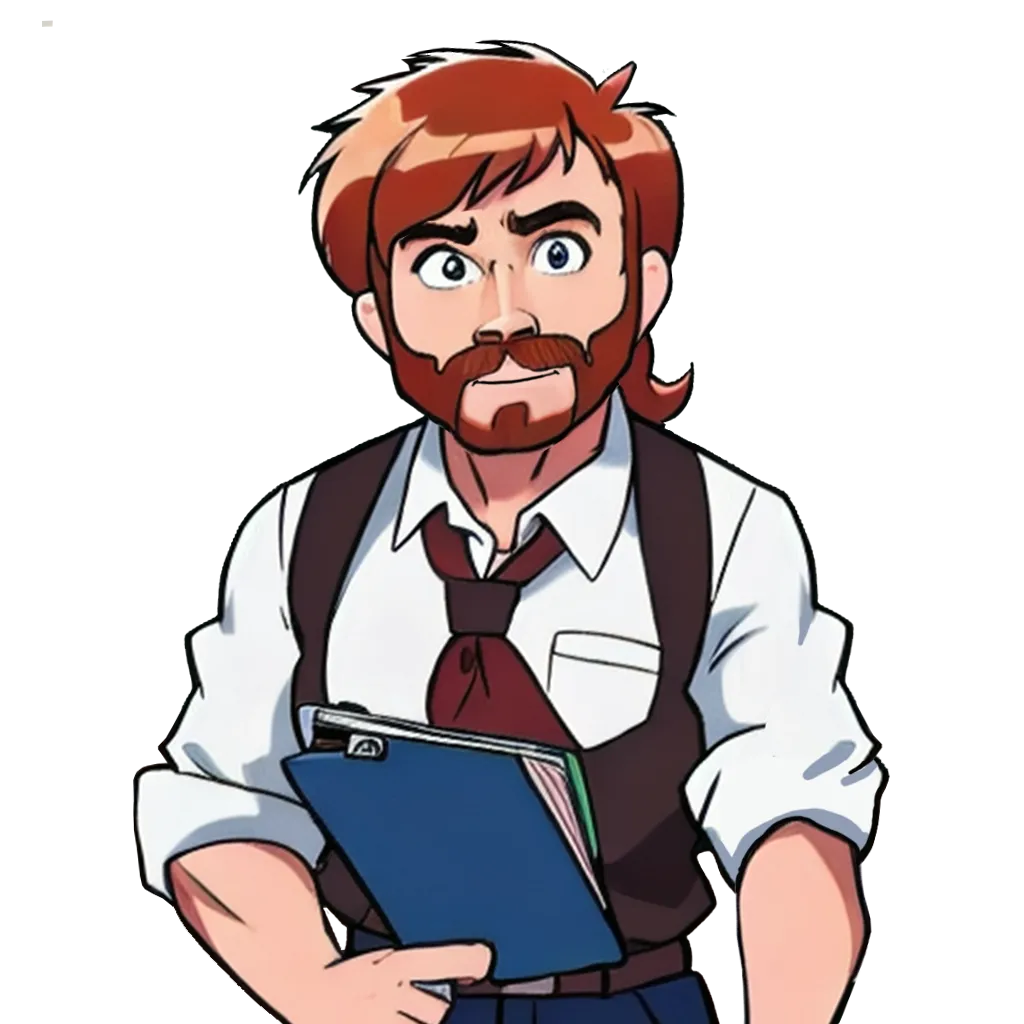