Basic React
Introduction to React
React is a JavaScript library created by Facebook, designed specifically to build interactive and dynamic user interfaces. Since its release in 2013, it has gained significant popularity due to its declarative approach and its ability to build high-performance web applications.
One of the main features of React is its ability to break down a complex interface into reusable and self-contained components. These components allow for organizing and structuring the code in a cleaner and more maintainable way.
Why React?
React offers many advantages in modern web development, some of the main reasons developers choose React are:
- Declarative: With React, you define how the user interface looks for a specific state, and React efficiently updates it when that state changes.
- Reusable Components: You can create independent components that can be reused in different parts of the application, reducing code duplication.
- Unidirectional Data Flow: The one-way data flow ensures data flows predictably and makes debugging and understanding application behavior easier.
- Large Ecosystem: React has a vast ecosystem of tools, libraries, and communities supporting it, making it easier to integrate new technologies and improve the development experience.
How React Works
At the core of React is the concept of Components. A component is a function or class that returns a piece of user interface (usually written in JSX). Let's look at a basic example of a functional component in React:
jsx
This example illustrates how a component receives "props" (properties) and returns a user interface using JSX, an extension of JavaScript that allows writing code that looks very much like HTML.
JSX: The Syntax of React
JSX is one of the most striking features of React. It is a syntax that allows combining JavaScript logic with HTML markup, greatly facilitating interface development. For example:
jsx
JSX is not mandatory in React, but it is widely used because it makes the code more intuitive and easier to read. Although JSX looks like HTML, under the hood it transforms into calls to the React API.
The Virtual DOM
Another key innovation of React is its efficient DOM handling through the Virtual DOM. Each time a component's state changes, React creates a virtual representation of the DOM in memory and then compares this version with the previous one. Only the parts that have changed are updated in the actual DOM, which significantly improves performance.
Basic Setup of a React Application
The first step to creating an application with React is to set up your development environment. The easiest way to start is by using the Create React App tool, which automates many of the initial configurations.
To install it, you need to have Node.js installed. Once you have it, you can run the following command in the terminal:
bash
This command initializes a React application with a basic file structure and a running development server.
Conclusion
React has revolutionized user interface development with its declarative approach, component-based architecture, and efficiency in DOM manipulation through the Virtual DOM. When starting with React, it is important to understand its fundamental concepts, such as components, JSX, and the Virtual DOM.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
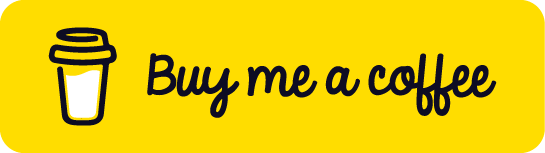
Chat with Chuck
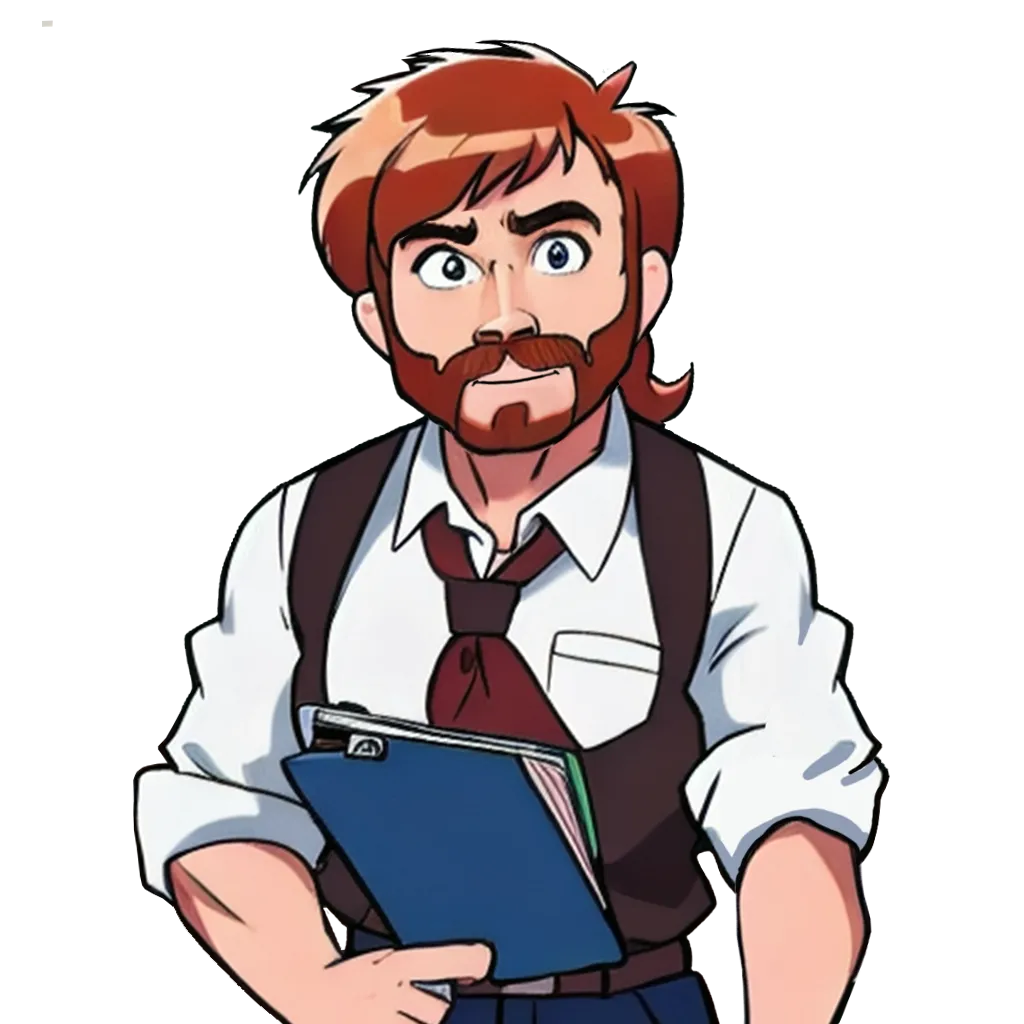
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps