Basic React
Testing in React
Testing is an essential part of application development to ensure that code functions correctly and that changes don't introduce errors. In React, there are several tools and approaches for testing components, from unit tests to integration tests. In this chapter, we will learn how to test React components using Jest and React Testing Library.
Tools for Testing React
Jest
Jest is a JavaScript testing framework that integrates seamlessly with React. Jest allows you to perform unit tests, integration tests, and has advanced features like mocks, fake timers, and code coverage.
React Testing Library
React Testing Library is a library that makes it easier to create tests for React components, focusing on user interaction rather than implementation details. It is known for its simplicity and focus on user behavior-based tests.
Setting up Jest and React Testing Library
If you are using Create React App, Jest and React Testing Library are already set up. If not, you can install them manually with the following command:
bash
With this setup, you can start writing tests for your React components.
Unit Tests in React
Unit tests are the simplest and focus on testing a specific part of the application, such as a component or a function.
Unit Test Example
Let's assume we have a simple component called Greeting
that displays a welcome message:
jsx
Here's a basic unit test for the Greeting
component:
jsx
This test verifies if the Greeting
component correctly renders the welcome message. We use screen.getByText
to find the text in the DOM and expect
to verify it is present.
Simulating Events with React Testing Library
Besides verifying rendered content, you can also simulate events like clicks or input changes. Let's say we have a button that increments a counter when clicked:
jsx
The test for this component will simulate a click on the button and verify that the counter has incremented:
jsx
Here, fireEvent.click
simulates a click on the button, and we verify that the counter's text updates correctly.
Testing Asynchronous Components
In React, it's common to work with asynchronous data like API requests. To test components that rely on this data, you can use functions like waitFor
to handle the asynchronous nature of the tests.
Asynchronous Test Example
Let's say we have a component that displays data after an asynchronous request:
jsx
The test for this component can wait for the data to become available using waitFor
:
jsx
In this test, we check that the loading message is displayed first, and then we use waitFor
to wait for the asynchronous data to appear.
Integration Tests
Integration tests verify how different components and functions interact with each other. For example, you might test how a form-handling component interacts with a validation component.
Integration Test Example
Let's say we have a simple form that shows a message when submitted successfully:
jsx
We can write an integration test to verify that the form submits and the success message appears:
jsx
This test verifies that the form is handled correctly and that the success message appears after submission.
Code Coverage with Jest
Jest also provides tools to generate code coverage reports, allowing you to see which parts of your application are being tested and which are not. To generate a code coverage report, simply run:
bash
This command runs the tests and generates a coverage report in the coverage
directory, where you can see which files and functions are fully tested.
Conclusion
Testing in React is essential for ensuring your application's quality. With Jest and React Testing Library, you can perform unit tests, simulate events, and handle asynchronous components easily.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
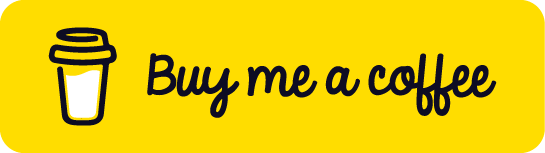
Chat with Chuck
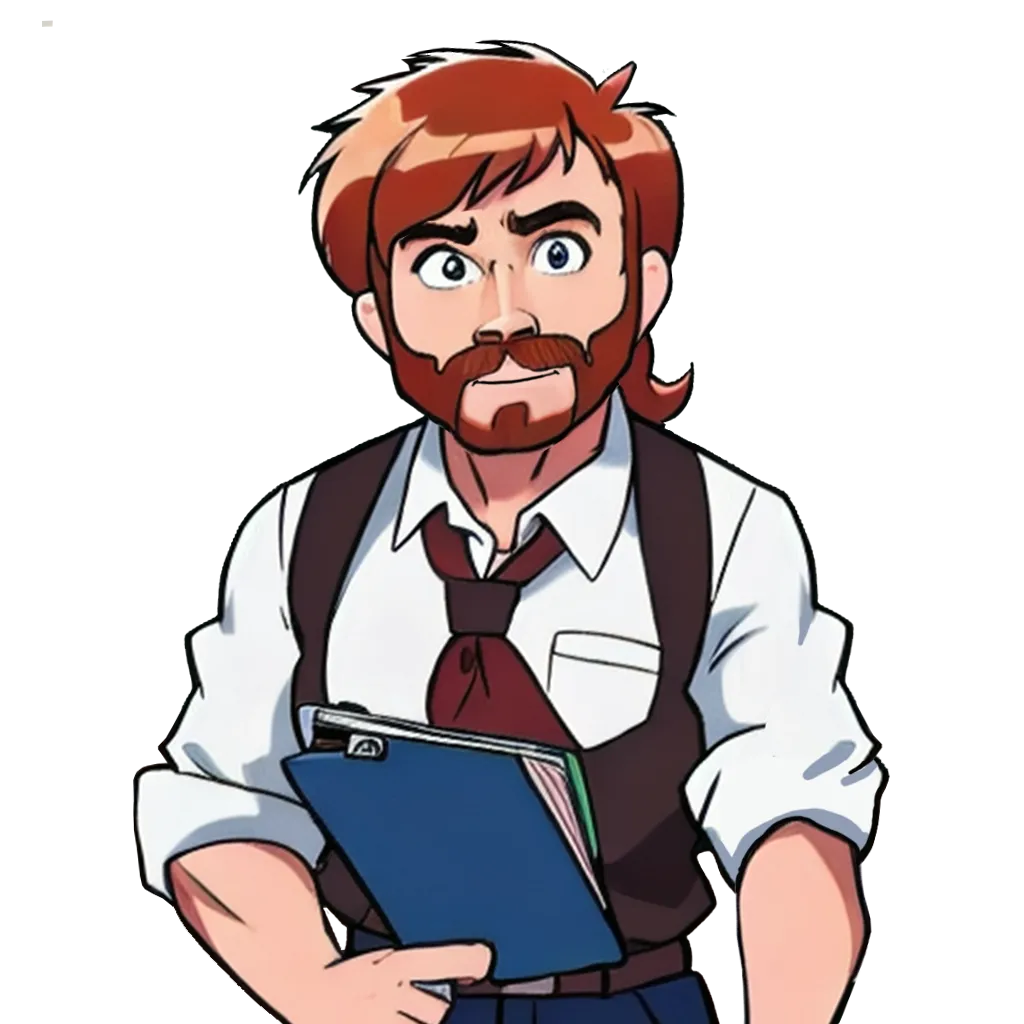
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps