Basic React
Best Practices and Patterns in React
To keep React applications efficient, scalable, and easy to maintain, it is essential to follow best practices and apply design patterns that help organize the code coherently. In this chapter, we will explore a series of recommendations that will help you write cleaner and more robust React code, as well as some common patterns used in React development.
Best Practices in React
1. Organize Code by Components
React is component-based, so it is important to organize the code into reusable, focused components with a single responsibility. Each component should be easy to understand and test in isolation.
Example of Componentization
Instead of having one large component that handles the entire flow of the application, divide the logic into smaller, manageable components:
jsx
Organizing the code this way not only improves readability but also helps reuse components in different parts of the application.
2. Keep Components Simple and Functional
Whenever possible, use functional components instead of class components, as they are easier to write and understand, and offer access to hooks, which provide a more modern and efficient way to handle state and side effects.
3. Keep State Localized
Although the use of global state is sometimes necessary, try to keep the state as localized as possible. Components that only need to manage their own state should use the useState
hook, while state that is shared across multiple components should be handled using the Context API or solutions like Redux.
Example of Using useState
for Local State
jsx
4. Keep Code Clean with Destructuring
Use destructuring of objects and arrays to write cleaner and more readable code. This is especially useful when dealing with props and state.
Example of Props Destructuring
jsx
Destructuring props and state makes the code easier to read and reduces the amount of repeated references to this.props
or this.state
.
5. Properly Handle Side Effects
Side effects, like data fetching or event subscriptions, should be handled carefully using the useEffect
hook. Ensure to clean up effects when the component unmounts to avoid memory leaks.
Example of Correct useEffect
Usage
jsx
6. Testing and Test Coverage
Testing is essential to ensure code quality. Use Jest and React Testing Library to write unit and integration tests for your components. Ensure the tests cover both success and error cases.
Common Patterns in React
Render Props Pattern
The render props pattern is a technique to share logic between components using a function passed as a prop. This pattern is used to create reusable components that handle common logic, such as form management or API interactions.
Example of Render Props
jsx
Container and Presentation Pattern
The container and presentation pattern separates the logic of components that handle data (containers) from components that are only responsible for presentation (presentation components).
Example of Container and Presentation Component
jsx
This pattern facilitates the reuse of presentation components and keeps the business logic separate, making the code easier to maintain and test.
Conclusion
Following best practices and applying common patterns in React is essential to maintain clean, scalable, and easy-to-maintain code. By organizing your code efficiently and using techniques like destructuring, hooks, and design patterns, you can write more robust and scalable applications. You are now ready to apply these best practices in your own React projects!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
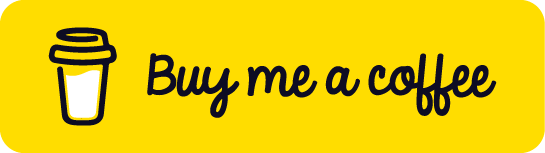
Chat with Chuck
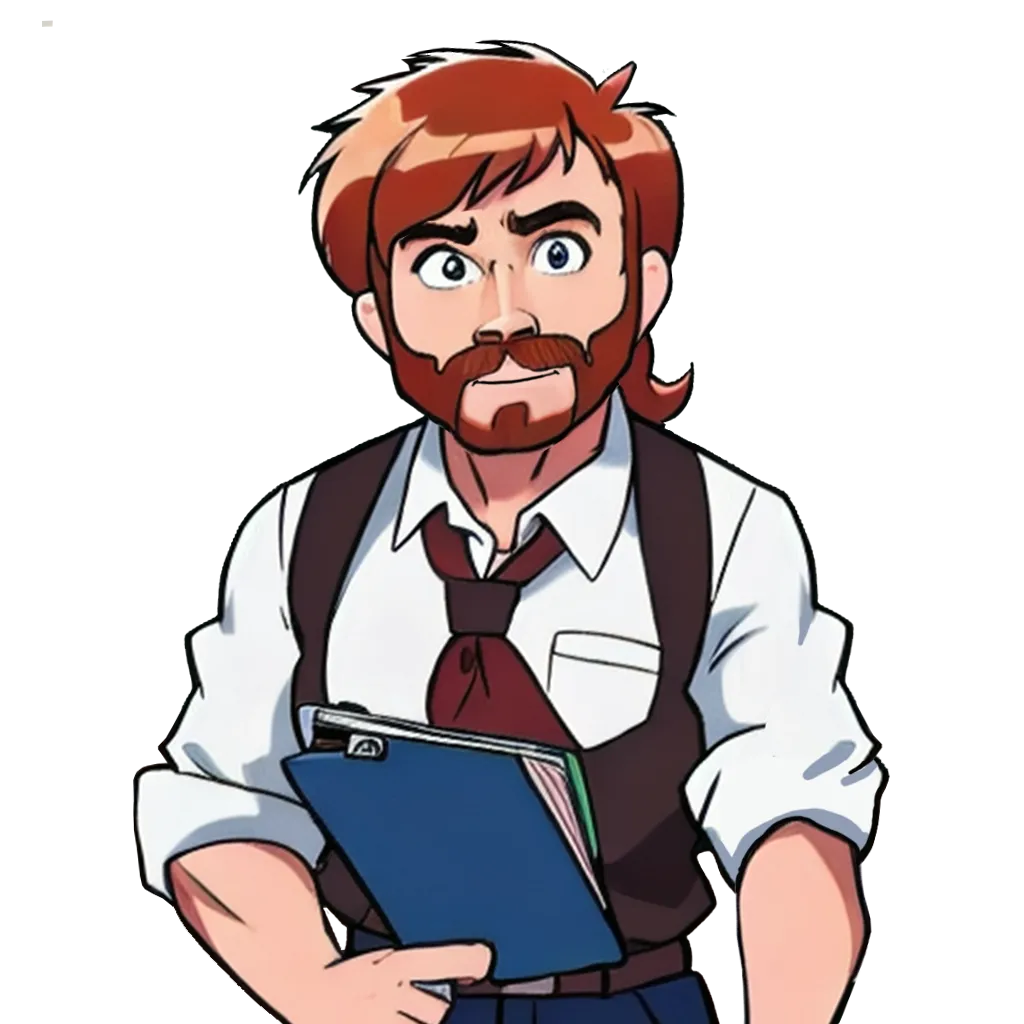
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps