Basic React
Conclusions and Next Steps
Reaching the end of this course is a great achievement, and now you have a solid foundation to work with React and create interactive and efficient web applications. Throughout the chapters, we've covered a wide range of topics, from React fundamentals to advanced topics like API management, performance optimization, WebSocket integration, and development best practices.
In this final chapter, we will summarize what we have learned and explore some of the next steps you can take to continue improving your skills in React.
Summary of What Was Learned
Throughout the course, we covered the following key topics:
- React Fundamentals: Introduction to JSX, functional components, and the component lifecycle.
- State and Hooks: Use of
useState
,useEffect
, and other hooks to manage state and effects in functional components. - API Management: Use of
fetch
and GraphQL to get and send data to and from servers. - Third-Party Components and Useful Libraries: Integration of libraries such as Material-UI, Ant Design, and React Hook Form to enhance application functionality.
- Performance Optimization: Use of tools like
React.memo
,useMemo
, anduseCallback
to optimize React app performance. - Authentication and Authorization: Implementing techniques to protect routes and handle authenticated users.
- Testing and Debugging: Unit and integration testing using Jest and React Testing Library.
- React Applications Deployment: Deployment on platforms like Netlify, Vercel, and GitHub Pages.
- Best Practices: Application of common patterns and recommendations to maintain clean and scalable code.
Next Steps
While this course covers a wide range of important topics in React, there is always more to learn and perfect. Here we suggest some next steps you can take to continue improving your skills:
1. Explore the React Ecosystem
The React ecosystem continues to grow, with new tools and libraries being released regularly. We recommend exploring the following areas:
- Next.js: A React-based framework that facilitates Server-Side Rendering (SSR) and static site generation. If you're interested in more advanced React applications, Next.js is an excellent choice.
- React Native: If you want to take your skills to mobile applications, React Native allows you to develop native mobile apps using React.
- Advanced State Management: Although we covered Redux, there are other state management tools like Recoil or MobX that offer different approaches to managing the application's global state.
2. Participate in Open Source Projects
Contributing to open source projects in the React ecosystem will not only help you improve your skills but also connect you with the community. You can find projects on GitHub or GitLab that use React and start contributing small improvements or bug fixes.
3. Perfecting Testing and Automation
Testing is a crucial skill in modern development. Consider delving into automated testing, including end-to-end (E2E) testing with tools like Cypress or Playwright. This will allow you to cover more aspects of your React applications and detect errors before they reach production.
4. Learn About Performance and Scalability
As React applications grow in size and complexity, performance optimization becomes increasingly important. Study topics like lazy loading, code splitting, and render optimization to ensure your applications remain fast and efficient.
5. Stay Updated
React continues to evolve with new releases, improvements, and best practices. Stay updated by reading the official documentation, community blogs, and participating in online forums or events like React Conf. This will keep you informed about the latest trends and technologies in frontend development.
Final Reflection
React is a powerful tool that has changed the way we build modern web applications. With its reusable components, focus on efficiency, and vast ecosystem, React remains one of the most demanded libraries in web development.
As you continue learning and applying React in your projects, remember that the key lies in practice and experimentation. Take advantage of the resources available, keep exploring new technologies, and don’t be afraid to dive into more challenging projects.
We wish you much success on your journey as a React developer!
You can take the Quiz for this topic:
You can also deepen in the following topics:
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
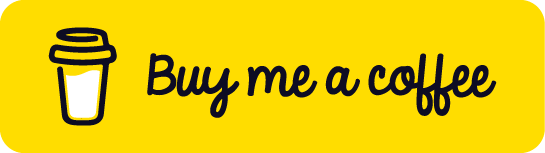
Chat with Chuck
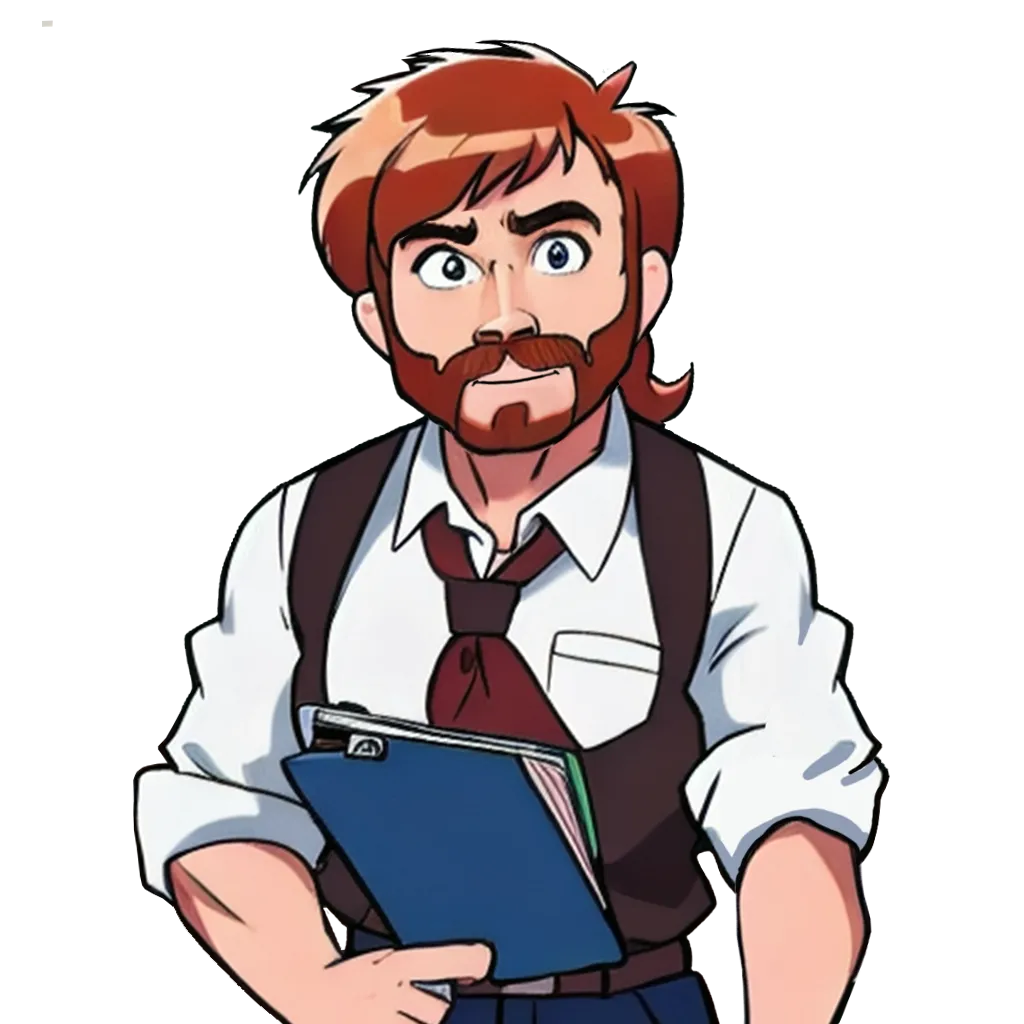
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps