Basic React
Communication between Components
In a React application, components do not operate in isolation; they often need to communicate with each other to share data or coordinate actions. In this chapter, we will learn how React components communicate using props and how to lift the state up to manage shared data.
Passing Props from Parent to Child
The most basic form of communication between components is passing props from a parent component to a child component. Props are simply parameters that are passed to components, allowing a parent component to send data to its children.
Example of Passing Props
Here is an example of how a parent component passes props to a child component:
jsx
In this example, ParentComponent
passes a value of message
as a prop to the ChildComponent
, which displays it in a paragraph. This is the simplest way to pass data from one component to another.
Typing Props with PropTypes
It is advisable to specify the type of props a component expects to receive to ensure that the passed data is correct. This can be done using the prop-types
library:
jsx
By defining propTypes
, you can ensure that the received props are of the correct type, which helps prevent runtime errors.
Bidirectional Communication: Lifting State Up
In some situations, a child component needs to send information back to the parent component. However, props are unidirectional, meaning data only flows from parent to child. To handle this, we can lift the state up to the parent component, known as lifting state up.
Example of Lifting State Up
Suppose we have a parent component that needs to receive data from a child component. We can achieve this by passing a function as a prop to the child, which it will call to send data to the parent.
jsx
In this example, the child component calls the onInputChange
function when the user types in the text field, allowing the parent component to receive and handle the input value.
Context API: Sharing Global State
When multiple components need to access the same data, passing props from one component to another can become tedious, especially if the components are at different levels of the hierarchy. To solve this problem, React provides the Context API, which allows sharing global state between components without prop drilling.
Example of Context API
Here is a basic example of how to use Context API to share state between components:
jsx
In this example, the parent component provides a value through MyContext.Provider
, and the child component consumes it using the useContext
hook, without needing to pass props manually.
Conclusion
Communication between components is an essential part of development with React. Whether passing props from parent to child, lifting up state, or using Context API to share data globally, React provides several efficient ways to coordinate the flow of data between components.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
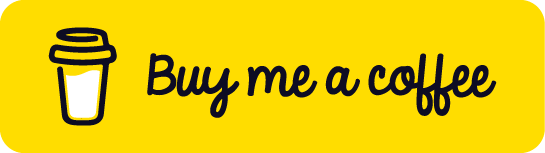
Chat with Chuck
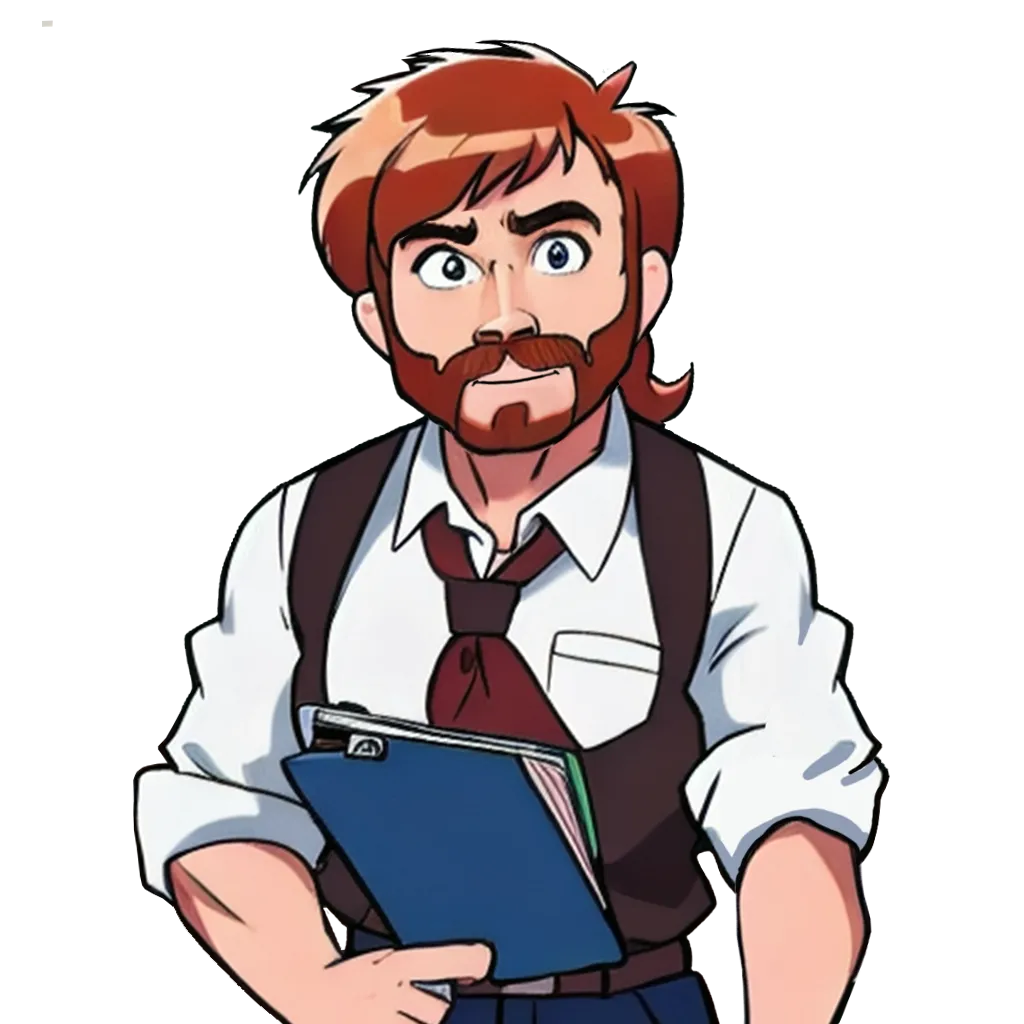
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps