Basic React
Using GraphQL with React
GraphQL is an alternative to REST that allows clients to request exactly the data they need, which can improve the efficiency of web applications. Unlike REST, where each endpoint returns a predefined set of data, GraphQL allows for customized queries, reducing the number of requests and the amount of data transferred.
In this chapter, we'll learn how to integrate GraphQL into a React application using Apollo Client, a popular library that simplifies managing GraphQL queries.
Introduction to GraphQL
GraphQL is a query language for APIs that allows clients to specify exactly the data they need. The GraphQL API exposes a single endpoint, and clients can perform queries, mutations (to modify data), and subscriptions (to receive real-time updates).
Advantages of GraphQL
- Specific Requests: Clients only request the data they need, avoiding the overhead of unnecessary data.
- Fewer Requests: Instead of making multiple requests to different endpoints, you can obtain all the information in a single request.
- Strongly Typed: GraphQL uses a type system that ensures the returned data has the expected structure.
Installing Apollo Client
To start using GraphQL in your React application, you can use Apollo Client, a powerful tool that simplifies integrating GraphQL with React. First, you need to install Apollo Client and graphql
:
bash
Configuring Apollo Client
Once installed, you need to configure Apollo Client in your application. The first step is to wrap your app with the ApolloProvider
component and configure the client:
jsx
In this example, ApolloClient
is configured with the GraphQL endpoint URL and an in-memory cache to improve performance. Then, the client is provided to the entire application via the ApolloProvider
component.
Querying with useQuery
Apollo Client's useQuery
hook allows you to perform GraphQL queries directly within React components. Here's an example of how to execute a simple query to retrieve user data:
jsx
Here, useQuery
is used to execute the GET_USERS
query. The loading state, error state, and resulting data are managed within the component. If the request is successful, the list of users is displayed on the screen.
Performing Mutations with useMutation
In addition to queries, you can also modify data in a GraphQL API using the useMutation
hook. Here's an example of how to use a mutation to create a new user:
jsx
The useMutation
hook is used to execute the CREATE_USER
mutation, which accepts name
and email
variables. When the form is submitted, a new user is created in the API, and the response is displayed.
Real-Time Subscriptions with useSubscription
GraphQL also supports subscriptions, which allow for receiving real-time updates when data changes. Here's an example of how to use the useSubscription
hook to listen to real-time events:
jsx
This component listens to the userAdded
subscription, and when a new user is added, the data is displayed in real-time.
Best Practices with Apollo Client
When working with Apollo Client, it's important to follow some best practices to ensure optimal performance and maintainable code:
- Use Cache: Properly configure the cache to avoid unnecessary requests and improve performance.
- Error Handling: Implement proper error handling to inform the user of issues with requests.
- Query Fragments: Use query fragments to avoid code duplication when multiple queries or mutations use the same fields.
Conclusion
Integrating GraphQL into React applications with Apollo Client allows for efficient and flexible data handling of queries and mutations. GraphQL offers greater granularity in data requests, reducing overhead and improving performance.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
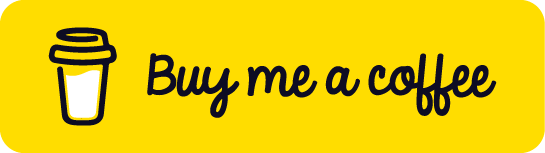
Chat with Chuck
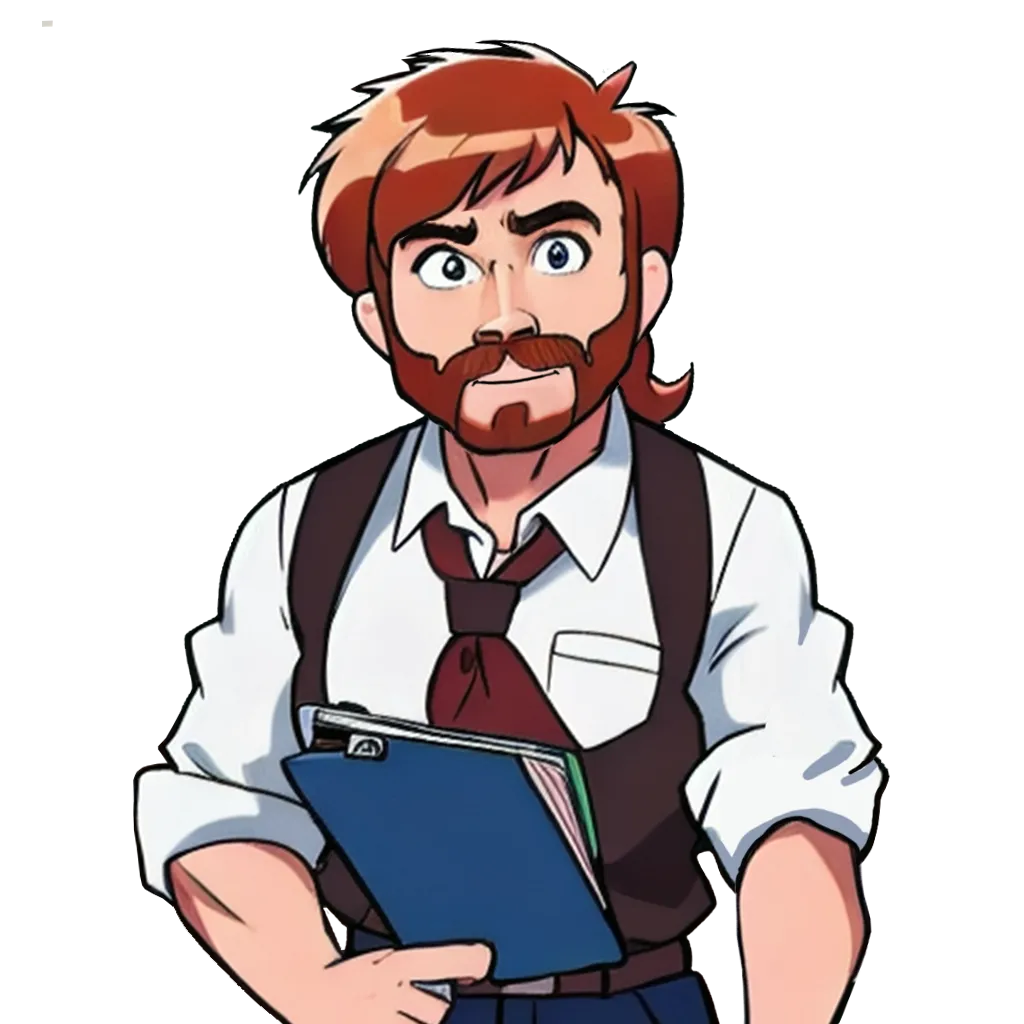
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps