Basic React
JSX Fundamentals
JSX, or JavaScript XML, is a syntax extension of JavaScript that allows you to write HTML-like structures directly in JavaScript code. It is one of React's most distinctive features and greatly facilitates the development of user interfaces.
Although JSX looks a lot like HTML, it's important to remember that it's not actually HTML. Under the hood, JSX is converted to React API calls, allowing for efficient interaction with the DOM. In this chapter, we will explore the fundamentals of JSX, its syntax, and how to use it correctly in React.
Basic Syntax of JSX
At first glance, JSX looks like HTML. For example, this JSX code:
jsx
This snippet creates a JSX element that represents an h1
element with the text "Hello, world!". However, JSX also allows you to utilize the power of JavaScript within the markup, as we'll see below.
Embedding JavaScript Expressions in JSX
One of the most powerful features of JSX is the ability to insert JavaScript expressions directly into the code. This is done using curly braces {}
. Let's see an example:
jsx
In this case, the variable name
is inserted into the JSX element. As a result, the text "Hello, John!" will be rendered in the user interface.
JSX is an Expression
In React, JSX is a valid JavaScript expression. This means you can use it within functions, assign it to variables, and return it from functions. For example:
jsx
This code demonstrates how JSX can be used as an expression within functions, allowing for the creation of dynamic and customized interfaces.
Attributes in JSX
JSX also allows you to add attributes to elements, similar to HTML. However, some attribute names in JSX may differ from those used in HTML. For example, class
in HTML becomes className
in JSX:
jsx
This is because class
is a reserved word in JavaScript, so React uses className
to avoid conflicts.
Boolean Attributes
Boolean attributes in JSX work similarly to those in HTML, but in JSX, if a boolean attribute is present, you don't need to specify its value. For example:
jsx
Here, the checkbox will be checked, as the checked
attribute is present. If you wanted it unchecked, you could simply remove the attribute or set checked={false}
.
JSX and Style Objects
Instead of using text strings for styles as in HTML, JSX uses JavaScript style objects. This is useful because it allows you to use JavaScript variables and logic within styles. For example:
jsx
This example applies styles to a div
using a JavaScript object. This way, you can leverage the power of programming to define and modify styles dynamically.
Comments in JSX
Although JSX looks like HTML, comments in JSX are slightly different. They must be placed within curly braces as JavaScript expressions. For example:
jsx
Comments in JSX won't be rendered in the DOM, allowing you to add useful notes within interface code.
Conclusion
JSX is a powerful tool that makes writing interfaces in React simpler and more efficient. Through its ability to mix HTML and JavaScript, JSX provides a declarative way to create dynamic and reusable components.
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps
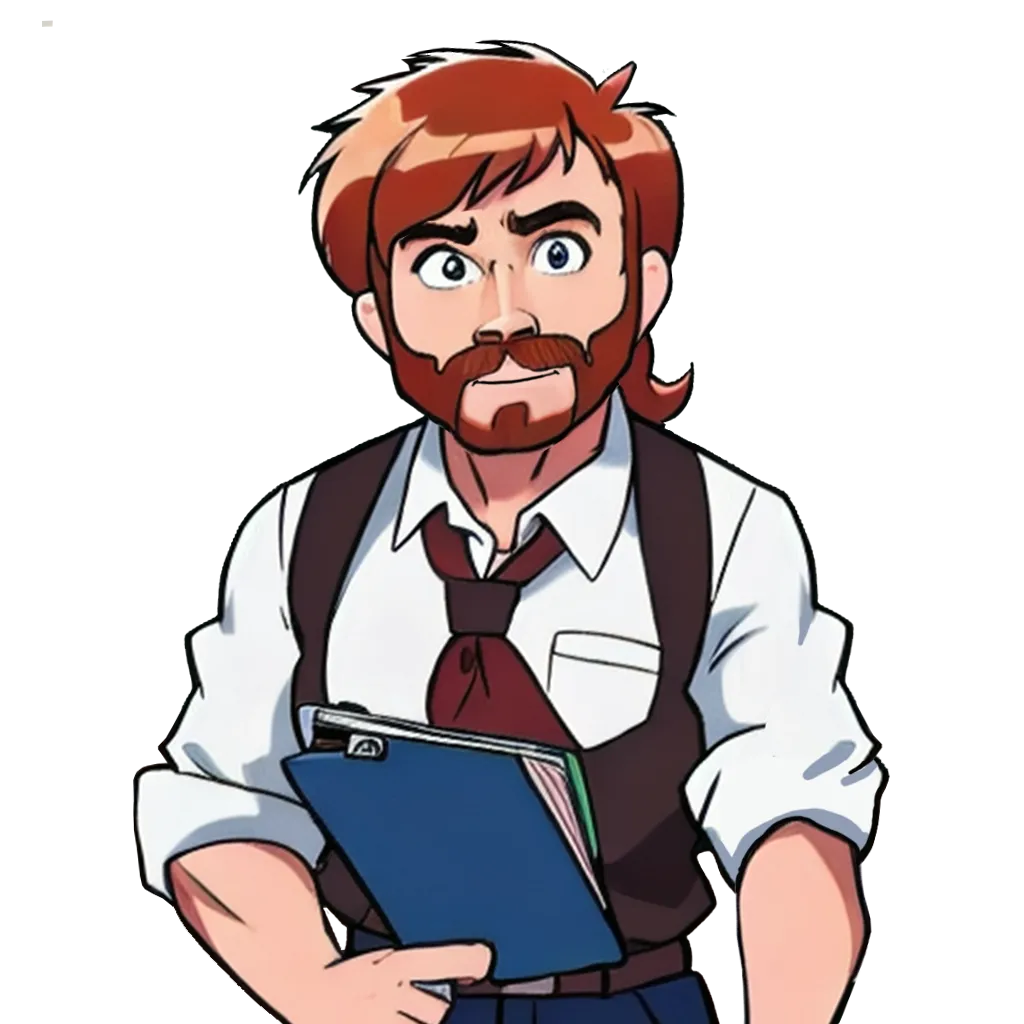