Basic React
Event Handling in React
Handling events in React is fundamental for making our applications responsive to user interaction. In this chapter, we will learn how to handle events such as button clicks, form submissions, and more, using functional components and JSX syntax.
React uses an event system that is very similar to native JavaScript events, but with some key differences that make it more efficient and easier to use. Let's see how it works.
Basic Event Handling
Just like in HTML, in React you can handle events like onClick
, onChange
, and onSubmit
. However, in JSX, instead of using lowercase as in HTML, the event names are written in camelCase.
For example, to handle a button click:
jsx
In this example, the handleClick
function is called when the user clicks the button. The onClick
event in React is similar to the native click event, but it is part of React's event system.
Passing Arguments to Event Handlers
In some cases, you may want to pass arguments to an event handler. This can be done using an arrow function in the event:
jsx
In this example, when the button is clicked, we pass the value 1
to the handleClick
function, which allows us to make the code more dynamic.
Synthetic Events in React
React uses a system of synthetic events, which means that events in React are wrapped by an abstraction layer that makes them work the same way across all browsers. This provides an additional layer of compatibility and efficiency since React manages all events with a single listener at the root of the document.
Preventing Default Behavior
In native JavaScript events, event.preventDefault()
is used to prevent the default behavior of an event, such as form submission. In React, it works the same way:
jsx
Here, the form will not be submitted when the user clicks the submit button, thanks to the event.preventDefault()
call.
Handling Events in Inputs and Forms
Handling events in form inputs is a common case in React applications. You can use the onChange
event to capture changes in the input values.
Example: Handling Text Input
Here's an example of how to handle text input in a form:
jsx
Here, the text
state is updated every time the user types in the text field. The current value of the text field is dynamically shown in a paragraph.
Composed Events
You can handle multiple events in a single component. For example, you can handle both click and submit events in a form:
jsx
In this case, when the button inside the form is clicked, both the onClick
and onSubmit
events are handled efficiently.
Conclusion
Handling events in React is a straightforward yet powerful process. By understanding how to handle events, prevent default behaviors, and update state in response to user interactions, you can create interactive and dynamic applications.
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps
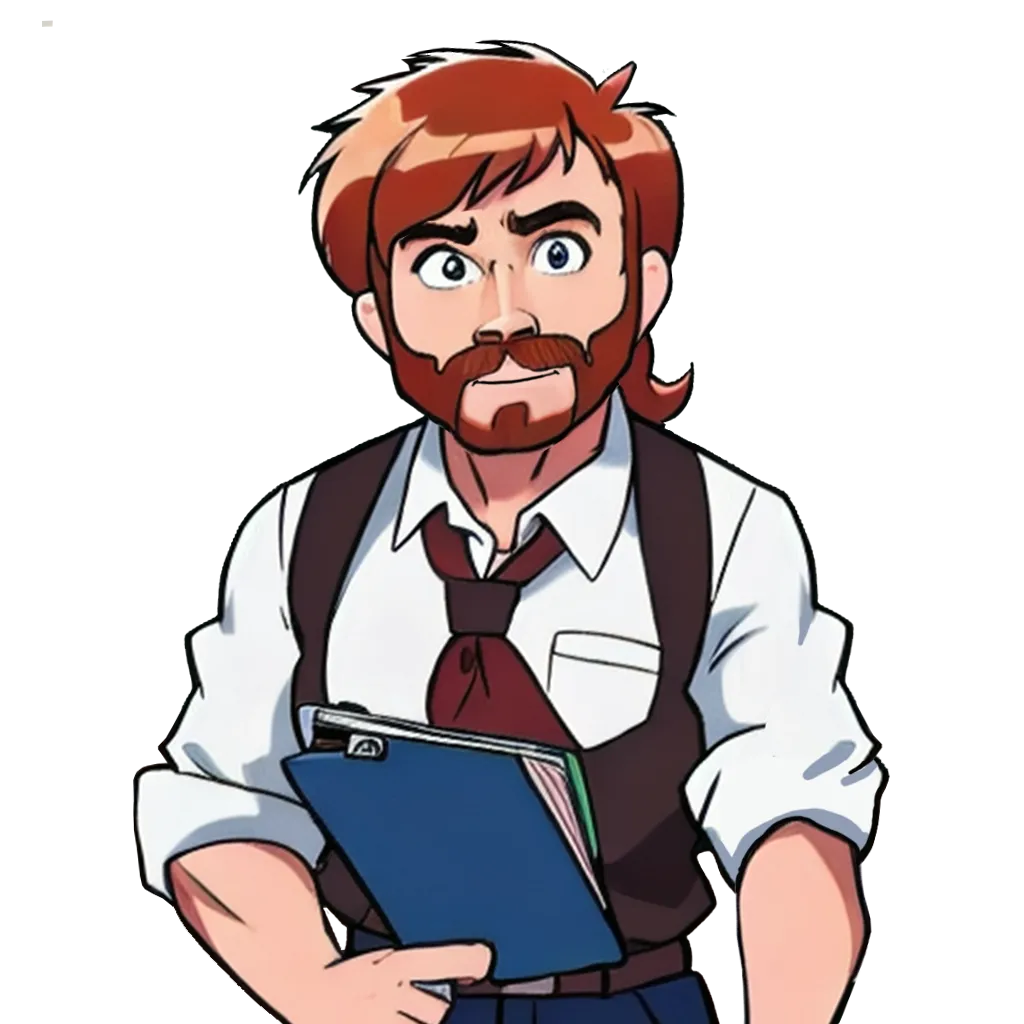