Basic React
State and Lifecycle with Hooks
In React, handling state and lifecycle of components is crucial for building dynamic user interfaces. Before the introduction of hooks, these functionalities were only available in class components. However, with the arrival of hooks in React version 16.8, we can now manage state and side effects declaratively in functional components.
In this chapter, we will explore the two most commonly used hooks: useState
and useEffect
. These hooks allow you to manage the local state of components and handle side effects such as DOM updates or API calls.
State Management with useState
The useState
hook allows you to add state to functional components. In class components, state was managed through this.state
and this.setState()
. With useState
, state is handled more simply and declaratively.
useState
Example
Here is an example of how to use useState
to manage the state of a counter:
jsx
In this example, useState
returns a pair of values: the current state (count
) and a function to update the state (setCount
). Every time the button is pressed, the state is updated, and React re-renders the component with the new value of count
.
Initial State in useState
The value you pass to useState
is the initial state. In the previous example, the initial state is 0
. You can also initialize the state using a function if the initialization is more complex:
jsx
This way, you can optimize performance by calculating the initial state only once when the component mounts.
Handling Side Effects with useEffect
The useEffect
hook allows you to handle side effects in functional components. Side effects are operations that affect external parts of the component, such as DOM updates, API calls, or setting up timers.
The useEffect
runs after the component has been rendered. You can think of it as a combination of the lifecycle methods componentDidMount
, componentDidUpdate
, and componentWillUnmount
from class components.
Basic Example of useEffect
Here is an example of how to use useEffect
to update the page title every time the counter value changes:
jsx
The useEffect
takes two arguments:
- A function containing the code we want to execute as a side effect.
- A list of dependencies (in this case
[count]
) that tells React when to execute the effect. If any of the dependencies change,useEffect
will re-run.
Conditional Effects and Cleanup
Some effects may require cleanup when the component unmounts or when the effect needs to be restarted. This can be done by returning a function from useEffect
:
jsx
In this example, we establish a timer that prints "Tick" to the console every second. When the component unmounts, the timer is cleared using clearInterval
.
Empty Dependencies
If you provide an empty dependencies list ([]
), the effect will only run once, when the component mounts, simulating the behavior of componentDidMount
in class components.
jsx
Conclusion
Handling state and side effects are fundamental concepts in React, and hooks like useState
and useEffect
allow us to implement them declaratively in functional components. These hooks are powerful and flexible, making them essential tools for any React developer.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
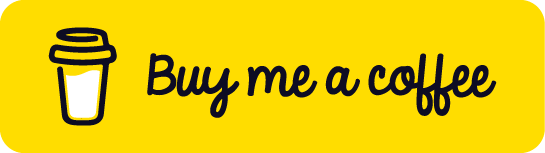
Chat with Chuck
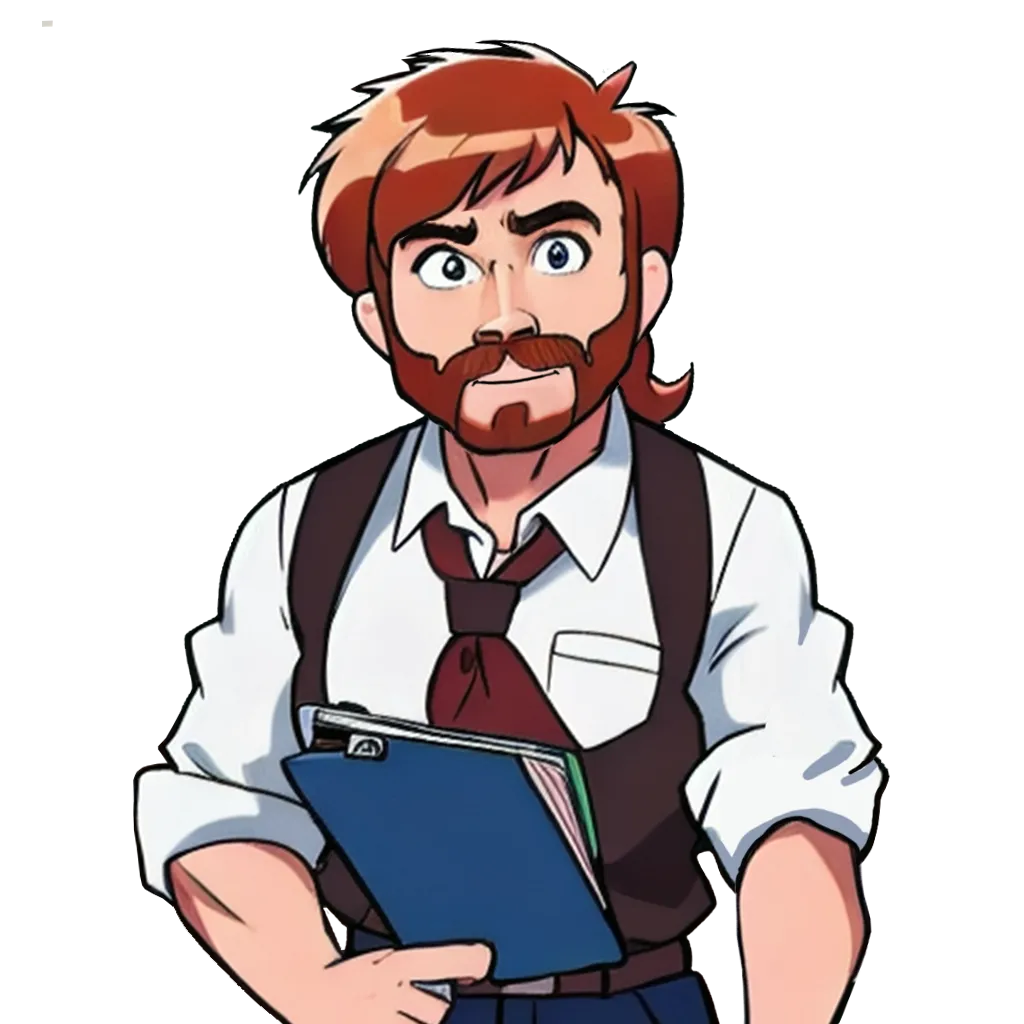
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps