Basic React
Styles in React
Styling an application is an essential part of frontend development, and React offers various ways to do it. In this chapter, we'll explore different ways to apply styles to React components, from using inline styles to using preprocessors like Sass.
Inline Styles
One of the simplest ways to apply styles to a component is by using inline styles. In React, inline styles are defined using a JavaScript object, allowing you to leverage the power of logic and variables within the styles.
Inline Style Example
jsx
Inline styles are convenient for small components or styles dependent on logic but are not ideal for handling complex or large-scale styles.
CSS in Separate Files
Another way to style is by using external CSS stylesheets, as you would in a traditional web application. React allows importing CSS files directly into the components.
CSS Import Example
jsx
This method keeps styles organized and separate from the component logic, making maintenance easier.
CSS Modules
CSS Modules allow styles to be specific to a component, preventing name collisions between different components. CSS Module files usually have the extension .module.css
.
CSS Modules Example
jsx
CSS Modules ensure each class is unique to its component, avoiding large-scale styling issues.
Styled Components and CSS-in-JS
Styled Components is a popular CSS-in-JS library that allows writing CSS directly in JavaScript files, defining stylized components that can be reused. This technique leverages JavaScript's power to apply dynamic styles.
Styled Components Example
jsx
Styled Components allow handling complex and dynamic styles within React components, keeping styles close to the component logic.
Integrating Sass in React
Sass (Syntactically Awesome Stylesheets) is a CSS preprocessor that adds powerful features like variables, nesting, and mixins. You can use Sass in React by configuring your project to compile .scss
or .sass
files.
Sass Setup in React
To use Sass in a React project, you first need to install the sass
dependency:
bash
Once Sass is installed, you can create files with the .scss
extension and use them directly in your React application.
Sass Example in React
scss
jsx
Sass allows writing more organized and reusable styles, using features like variables and mixins to enhance CSS code efficiency.
Conclusion
React offers a variety of options for styling your components, from inline styles to more advanced solutions like CSS Modules, Styled Components, and Sass. Each method has its advantages depending on the size and complexity of your application.
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps
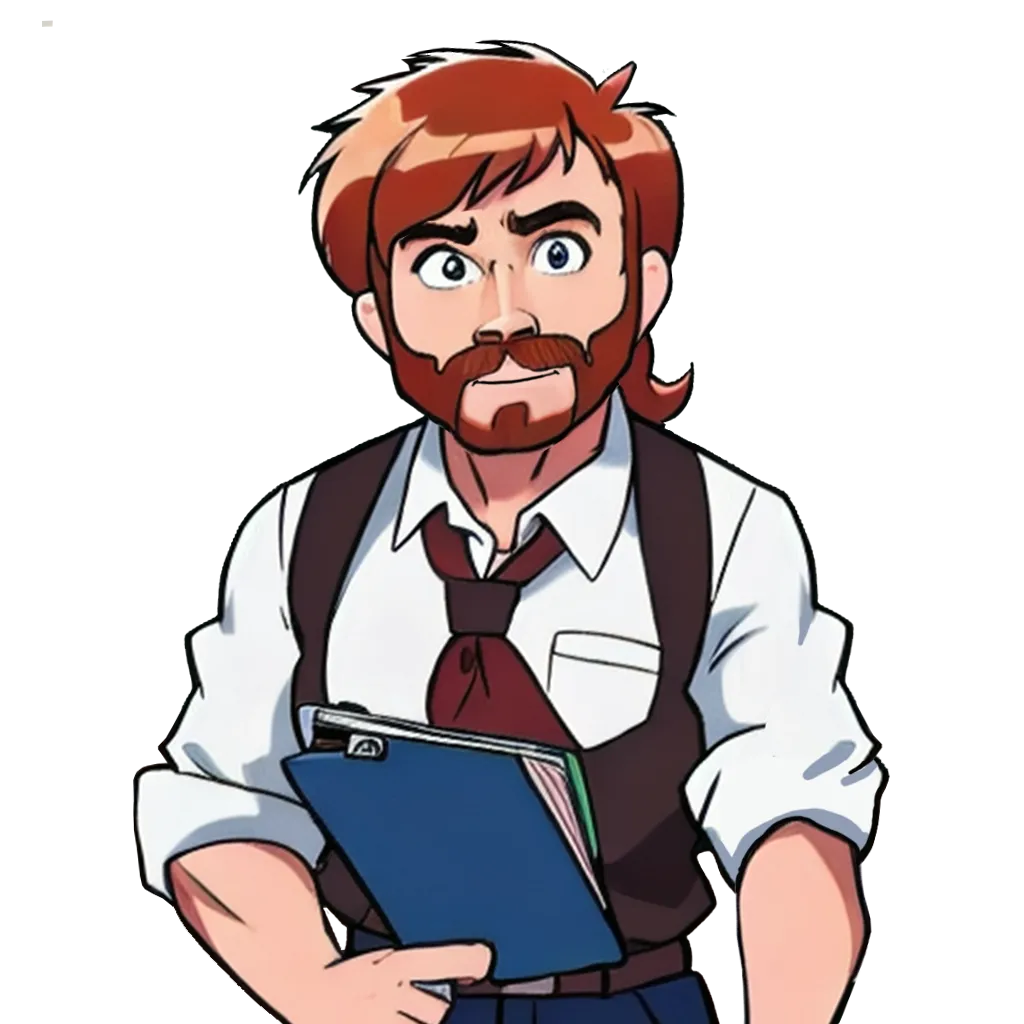