Basic React
Forms and Input Handling in React
Forms are an essential part of many web applications, as they allow users to submit data and interact. In React, handling forms can seem different from traditional HTML, but it offers more precise control over input values and behavior.
In this chapter, we will learn how to handle controlled and uncontrolled forms, basic input validation, and how to submit form data in React.
Controlled Forms
In React, a form is called "controlled" when the input state is managed by the React component. This means that every time a user interacts with the form, the input value is updated in the component's state.
Controlled Form Example
Here is an example of a controlled form where the text input value is updated as the user types:
jsx
In this example, the value of the text field is managed by the component's state. Each time the user types, the state is updated with the new value, allowing full control over the input value.
Multiple Inputs in a Controlled Form
If you have multiple fields in a form, you can manage all values within a single state using an object:
jsx
In this example, multiple inputs are managed within a single formData
object, making it more efficient to handle complex forms.
Uncontrolled Forms
In contrast to controlled forms, an uncontrolled form does not store the input value in the React state. Instead, the value is accessed directly from the DOM using a reference (ref
).
Uncontrolled Form Example
Here is an example of an uncontrolled form in React:
jsx
In this example, the input value is accessed directly from the DOM using a reference (ref
). While this approach can be useful in some cases, controlled forms are generally preferred in React for offering greater control over the data.
Form Validation
Validating form data is crucial to ensure that the user has entered correct information. In React, you can handle validation directly within the form handler.
Form Validation Example
Here is a basic example of validating an email field in a form:
jsx
In this example, the email field is validated to check if it contains an @
character. If it is not valid, an error message is displayed below the input.
Conclusion
Handling forms and inputs in React is an important part of developing interactive applications. By learning how to handle controlled and uncontrolled forms and data validation, you can build more robust and reactive forms.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
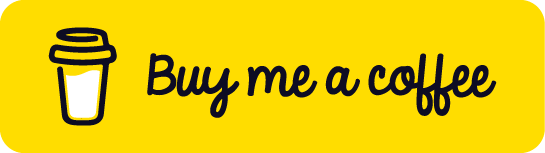
Chat with Chuck
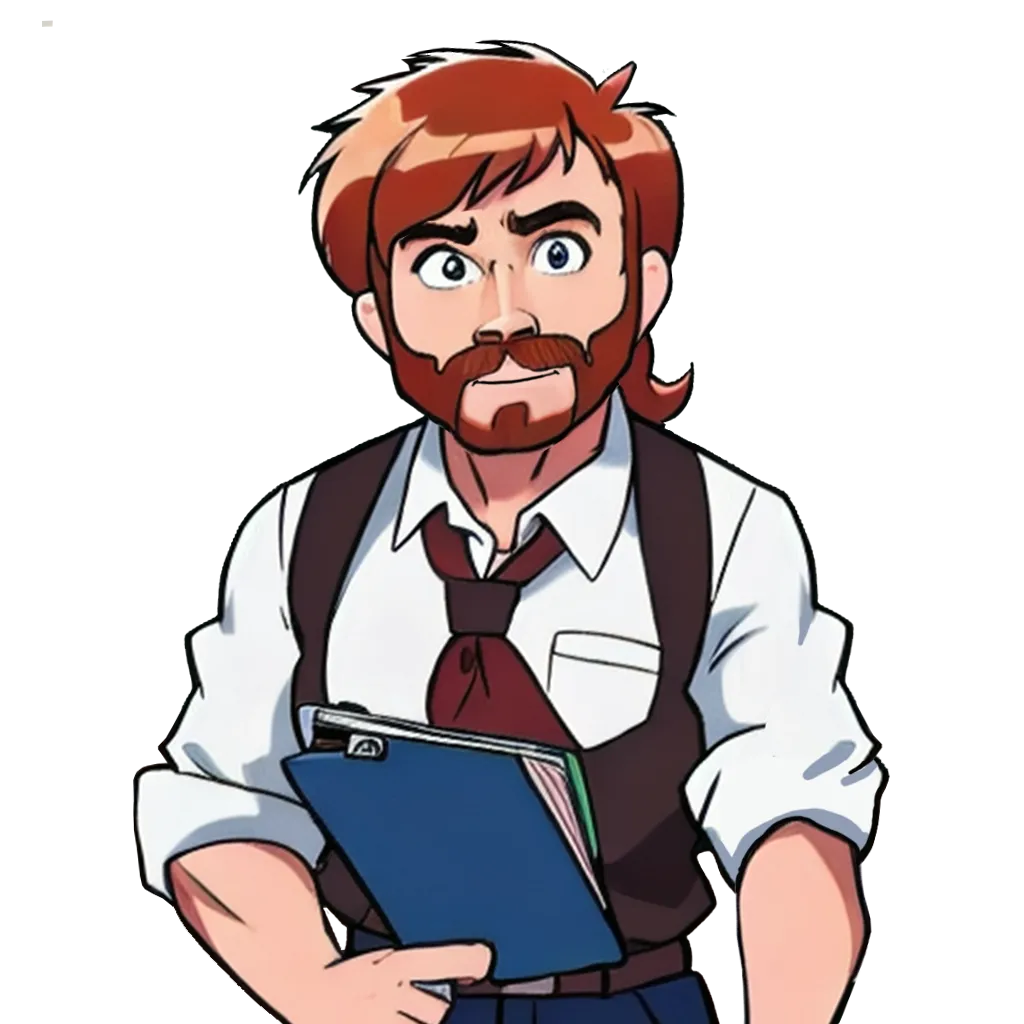
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps