Basic React
Integration with WebSockets
WebSockets are a technology that enables real-time bidirectional communication between the client and the server. Unlike traditional HTTP requests, which are unidirectional, WebSockets allow both the client and server to send data at any time without the need for a prior request.
In this chapter, we will learn how to integrate WebSockets into a React application to handle real-time communication.
What are WebSockets?
WebSockets allow you to maintain an open connection between the client and the server, allowing both to send data in real time. This technology is useful for applications that require constant updates, such as live chats, real-time notifications, or financial applications.
WebSockets Workflow
- The client establishes a connection with the WebSocket server.
- The server accepts the connection, and both can exchange messages at any time.
- The connection stays open until either the client or the server closes it.
Installing a WebSockets Library
Although JavaScript has a native API for WebSockets, there are libraries that simplify their use in React applications. One popular library is socket.io-client, which provides a robust solution for real-time communication.
Installation
To install the Socket.io client library, use the following command:
bash
Connecting to a WebSocket Server in React
To integrate WebSockets into React, you can use useEffect
to establish the connection when the component mounts. Here is a basic example of how to connect to a WebSocket server using Socket.io:
jsx
In this example, the client connects to a WebSocket server at http://localhost:3000
and listens for message events. When a message is received, the component's state is updated.
Sending Messages to the Server
In addition to receiving messages, you can also send data to the server using the WebSocket connection. Here’s an example of how to send a message to the server when a user clicks a button:
jsx
This component allows the user to send a message to the server when they click a button. The server can handle this message and respond to the client if necessary.
Integration with an Express Backend
If you are using a Node.js server with Express, you can easily integrate WebSockets with Socket.io to handle real-time communication.
Server Setup
Here is a basic example of how to set up an Express server that supports WebSockets with Socket.io:
js
In this example, the server listens for WebSocket connections and responds when it receives a message from the client. This allows bidirectional communication between the server and the client.
Common Use Cases
Real-Time Chat
WebSockets are ideal for building real-time chat applications. Each user can send messages that are broadcast to all connected users, creating an instant chat experience.
Real-Time Notifications
Real-time notifications, like social media alerts or event updates, can be easily implemented with WebSockets, allowing the server to send notifications to clients when an event occurs.
Financial Applications
Applications that require real-time data, such as trading platforms or market analysis, also benefit from using WebSockets to receive price updates or other live data.
Performance Considerations
Although WebSockets offer efficient real-time communication, it's important to take some performance considerations into account:
- Scalability: If your application has many concurrent users, ensure that the WebSocket server is optimized to handle multiple simultaneous connections.
- Inactive Connection Management: Implement logic to detect and close inactive connections to free up server resources.
- Security: Use wss (WebSocket Secure) to secure connections, especially if handling sensitive data.
Conclusion
WebSockets are a powerful tool for adding real-time functionality to your React applications. Whether for chats, notifications, or financial applications, WebSockets provide an efficient way to maintain communication between the client and the server.
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps
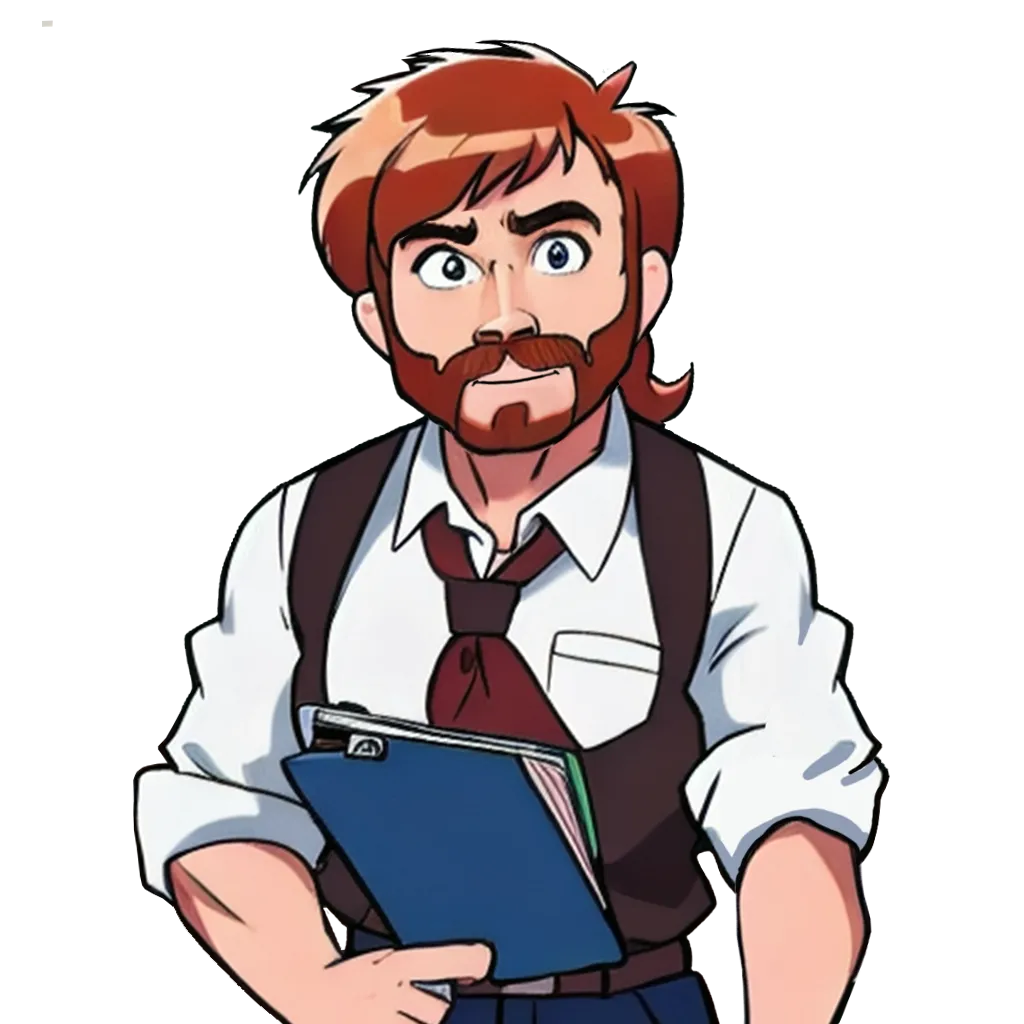