Basic React
Functional Components in React
Components are the cornerstone of React. In this chapter, we will delve deeply into how to create and use functional components, which are the basis for building user interfaces in React. Since version 16.8 of React, functional components are the preferred way to create components, thanks to the introduction of hooks.
What is a Functional Component?
A functional component is simply a JavaScript function that returns JSX, which means it defines a part of the user interface. Unlike class components, functional components do not have internal state or explicit React lifecycle methods, but with hooks, they can handle these functionalities declaratively.
Basic Example of a Functional Component
Let's look at a simple example of a functional component in React:
jsx
In this example, Welcome
is a component that receives a props
object and returns a JSX element displaying a personalized welcome message. The use of props
allows functional components to be reusable, as they can receive different input data.
JSX in Functional Components
As we saw in the previous chapter, JSX is the syntax that allows us to write HTML-like code in React. Within functional components, the use of JSX is essential to define what will be displayed in the user interface.
For example, we can extend the Welcome
component to make it more dynamic:
jsx
This component now returns multiple JSX elements wrapped in a div
. It is good practice to wrap multiple elements in a container like a div
or use React.Fragment
when necessary.
Props in Functional Components
Props (properties) are arguments passed to components, making them dynamic. For example, we could reuse the Welcome
component to display different names:
jsx
Here, the App
component uses the Welcome
component three times, passing different values for the name
property. In this way, functional components can be reused with different inputs, making development more efficient and modular.
Default Props
Sometimes, it is useful to set default values for props if one is not explicitly provided. This can be done using defaultProps
:
jsx
Here, if name
is not provided, the component will by default display "Hello, Stranger." This ensures that there is always a value present, preventing runtime errors.
Fragments in React
React allows for returning multiple elements in a single component without needing an additional container like div
. This is achieved using React.Fragment or its shorthand <>
:
jsx
This is useful when we don't want to add additional nodes to the DOM, keeping the structure clean and semantic.
Nested Components
In React, you can nest functional components within others, allowing for the interface to be divided into small, manageable pieces. Let's see an example of nested components:
jsx
Here, the App
component includes three components: Header
, Welcome
, and Footer
. This approach of dividing the interface into small, reusable components is one of the best practices in React.
Conclusion
Functional components are the core of any React application. They are lightweight, easy to read, and when combined with hooks, allow managing the application's state and lifecycle declaratively.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
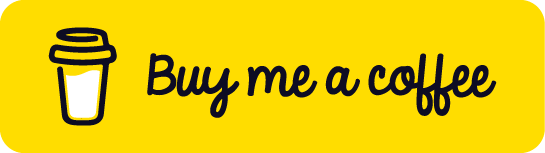
Chat with Chuck
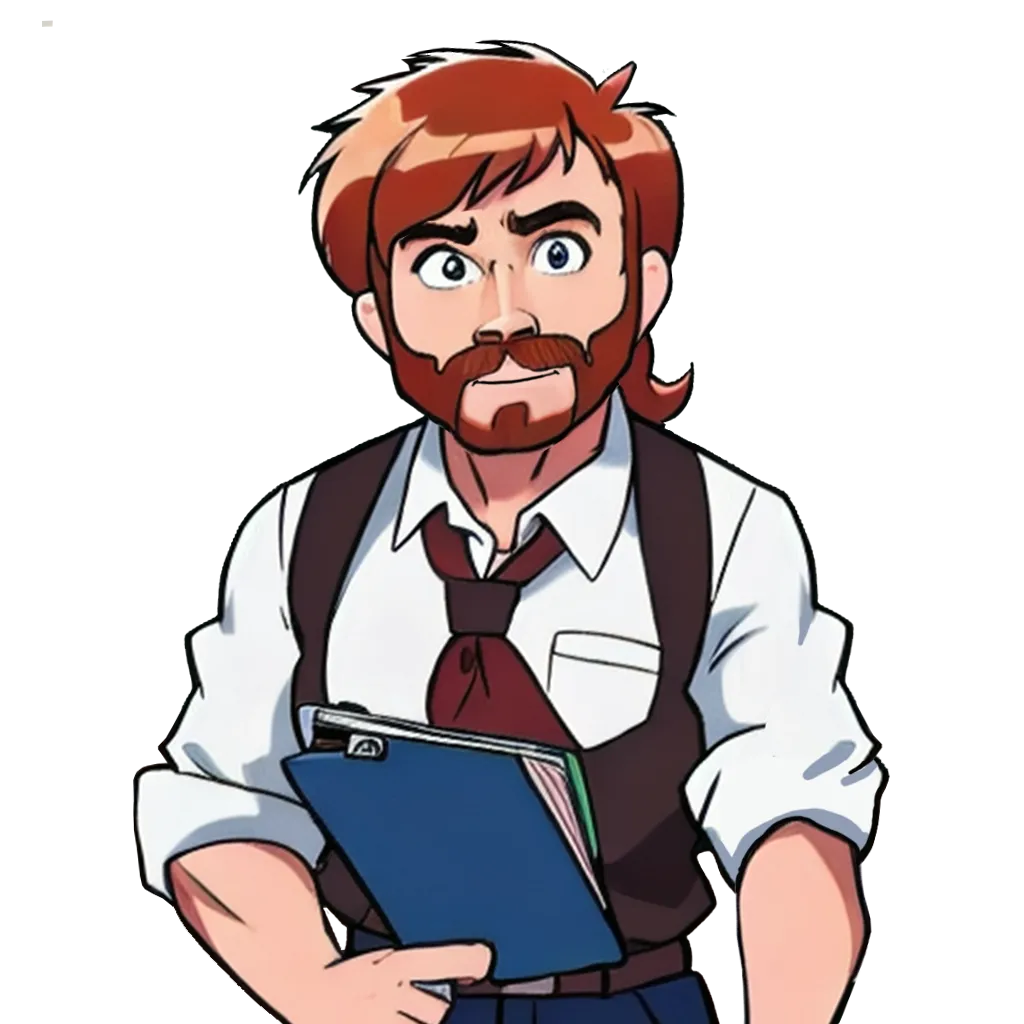
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps