Basic React
Introduction to Class Components
Although functional components and hooks are the recommended way to work in React in the latest versions, class components are still important to understand React's legacy, and they can still be found in many existing applications and libraries.
In this chapter, we will learn what class components are, how they work, and how to manage state and lifecycle within them. Even though their usage has decreased in favor of hooks, knowing class components is useful for working on older projects or to better understand the evolution of React.
This image shows lifecycle methods in React
What is a Class Component?
A class component is a way to define components in React using ES6 class syntax. These components can have their own internal state and handle React's lifecycle through special methods.
Basic Example of a Class Component
Here is a simple example of a class component that displays a greeting message:
jsx
In this example, the Greeting
component extends from React.Component
and must implement a render
method, which is responsible for returning the JSX that is displayed on the screen.
State in Class Components
Unlike functional components, which use the useState
hook to manage state, class components use a property called state
. The state is initialized within the class constructor and updated using the setState
method.
Example of State in a Class Component
jsx
In this example, the Counter
component initializes the count
state in the constructor and updates it using setState
. The setState
method is responsible for re-rendering the component when the state changes.
Lifecycle of Class Components
Class components have several lifecycle methods that allow performing actions at specific points during the component's life, such as when it mounts, updates, or unmounts.
Common Lifecycle Methods
-
componentDidMount
: Executes after the component has mounted in the DOM. Useful for operations like API requests. -
componentDidUpdate
: Executes after the component has updated due to changes in props or state. -
componentWillUnmount
: Executes right before the component unmounts and is removed from the DOM. Useful for cleaning up resources like timers or subscriptions.
Lifecycle Example
jsx
In this example, the Timer
component starts a timer when it mounts in the DOM and stops it when it unmounts. Additionally, componentDidUpdate
logs a message to the console every time the time
state updates.
Differences between Class and Functional Components
With the introduction of hooks in React 16.8, functional components can now handle state and effects similarly to class components. However, there are some key differences:
- Syntax: Class components require more boilerplate (such as the constructor and lifecycle methods), whereas functional components with hooks are more concise.
- Use of Hooks: Hooks offer a more direct and flexible way to manage state and effects in functional components.
- Legacy: Although functional components are more common in modern development, it's important to understand class components due to their presence in older applications and libraries.
Comparison chart of Functional Components and Class Components
Conclusion
Class components have been fundamental in React development before the introduction of hooks, and they are still relevant in many existing applications. While functional components and hooks are now the standard, understanding class components is valuable for maintaining and updating older applications.
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps
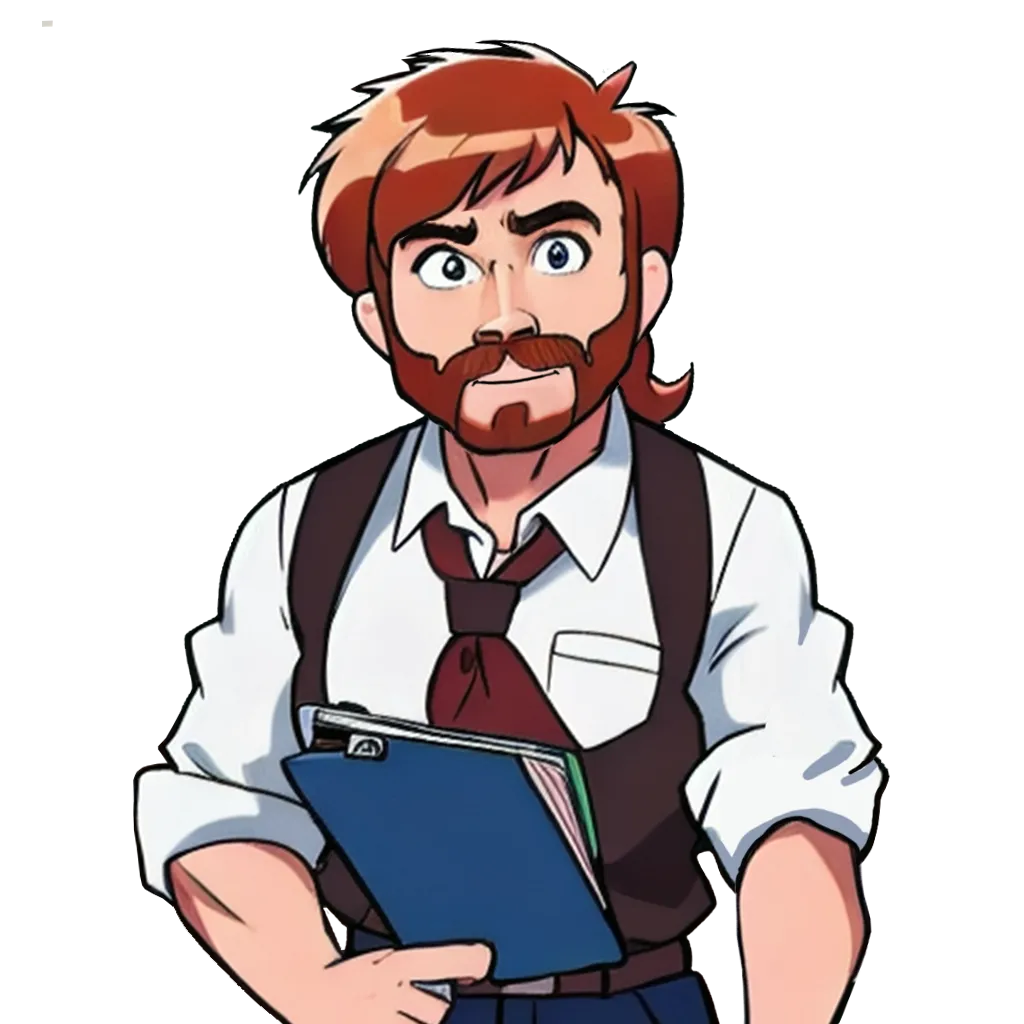