Basic React
Performance Optimization in React
As React applications grow in size and complexity, performance optimization becomes a priority. React offers several tools to minimize unnecessary renders, improve efficiency, and maintain a smooth user interface.
In this chapter, we will explore how to use React.memo
, useMemo
, and useCallback
to optimize the performance of React applications.
Avoiding Unnecessary Renders with React.memo
React.memo
is a higher-order function that you can apply to a functional component to prevent it from re-rendering unnecessarily. React re-renders a component every time its props change. However, if the props don't change, we can use React.memo
to tell React to reuse the previous render result.
Example of React.memo
jsx
Here, the Button
component only renders if its onClick
or label
props change. If there are no changes, React.memo
reuses the previously rendered component.
When to use React.memo
?
Use React.memo
when the component:
- Is heavy in terms of rendering.
- Doesn't need to update unless its props change.
- Is nested in other components that frequently render.
Optimizing Heavy Calculations with useMemo
useMemo
is a hook that allows you to memoize the result of a costly function or heavy calculation. It will only recalculate the value if the specified dependencies change.
Example of useMemo
jsx
In this example, the function inside useMemo
only executes when num
changes. This optimizes performance by avoiding unnecessary calculations on each render.
When to use useMemo
?
Use useMemo
when:
- You have costly or heavy calculations.
- You want to avoid recalculating a value on every render.
Memoizing Functions with useCallback
useCallback
is a hook similar to useMemo
, but it is specifically designed to memoize functions. When you pass functions as props to other components, React recreates them on each render. useCallback
allows you to avoid this constant recreation.
Example of useCallback
jsx
Here, the ChildComponent
only re-renders if the increment
function changes, preventing unnecessary renders.
When to use useCallback
?
Use useCallback
when:
- You pass functions as props to child components.
- You want to avoid constantly recreating functions that haven't changed.
Additional Optimization Techniques
Besides React.memo
, useMemo
, and useCallback
, there are other techniques and tools that can help you optimize your React applications:
Lazy Loading
React allows you to lazily load components using React.lazy
and Suspense
. This is useful for code splitting and only loading the necessary parts when the user needs them.
jsx
This improves performance by reducing the initial bundle size that is loaded in the application.
Render Optimization with Fragments
Use React.Fragment
to group multiple elements without adding extra nodes to the DOM. This reduces DOM overhead by maintaining a cleaner structure.
jsx
Conclusion
Optimizing performance in React is crucial for large-scale applications. Tools like React.memo
, useMemo
, and useCallback
help avoid unnecessary renders and heavy calculations. Alongside other techniques like lazy loading, you can ensure your React application remains fast and efficient.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
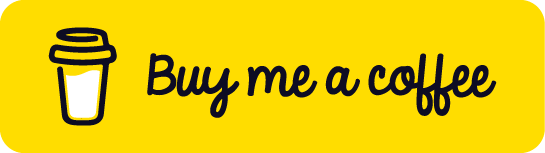
Chat with Chuck
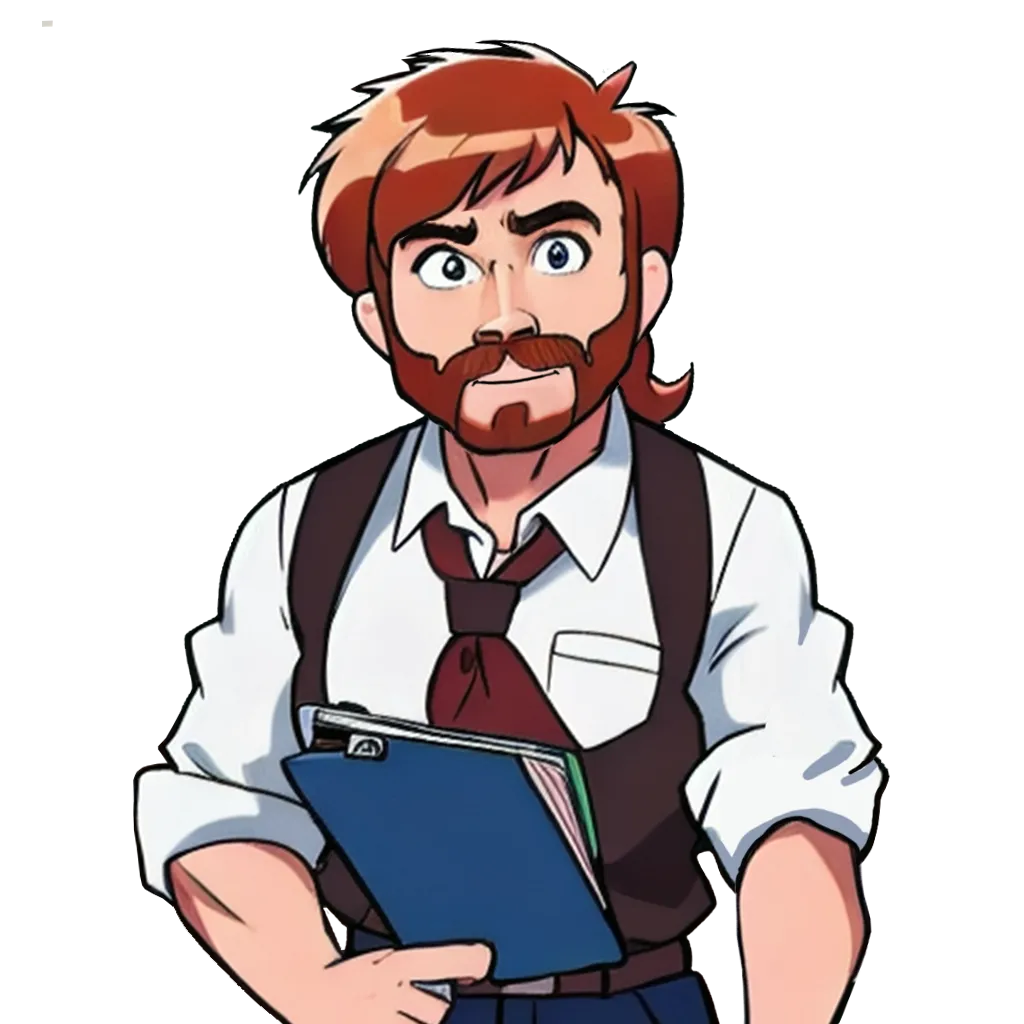
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps