Basic React
Creating Custom Hooks in React
Custom hooks in React allow you to reuse stateful and side effect logic across multiple components. Although React provides built-in hooks like useState
and useEffect
, custom hooks let you create specific functions that encapsulate common logic, making it easier to manage and reuse code.
In this chapter, we will learn how to create custom hooks and see some useful examples to use in React applications.
What are Custom Hooks?
A custom hook is simply a JavaScript function whose name begins with use
and can use other hooks within it. Custom hooks follow the same rules as built-in hooks: they must be called at the top level of a functional component and should not be called conditionally.
Basic Example of a Custom Hook
Here is an example of a custom hook called useToggle
, which handles a boolean value and toggles its state:
jsx
This useToggle
hook returns a boolean value and a function to toggle its state. It can be used in any component where you need this behavior:
jsx
Here, ToggleComponent
uses useToggle
to manage a state that toggles between true and false. This is useful in any situation where you need a boolean state that alternates, such as switches or dropdown menus.
Handling Side Effects with Custom Hooks
Custom hooks can also handle side effects using useEffect
. For example, we can create a hook that makes an HTTP request when the component mounts and stores the data in the state.
Example: Custom Fetch Hook
Here is an example of a custom hook called useFetch
, which makes a fetch
request to an API and manages the data state:
jsx
This useFetch
hook encapsulates the logic for making HTTP requests and managing the loading state, allowing you to reuse this logic in different components:
jsx
In this example, DataComponent
uses the useFetch
hook to get data from an API and display it once the request is complete.
Performance Optimization with Custom Hooks
You can use custom hooks to optimize your application's performance. A common example is handling user input with a delay (debouncing) to avoid API calls on every keystroke.
Example: useDebounce
Hook
Here is an example of a custom hook called useDebounce
, which delays updating a value until the user stops typing:
jsx
The useDebounce
hook is useful when you need to wait for the user to stop typing before performing an action, like making an HTTP request or validating a form:
jsx
This SearchComponent
uses useDebounce
to wait for the user to stop typing before performing a search, avoiding making API requests with every keystroke.
Best Practices for Creating Custom Hooks
When creating custom hooks, follow these best practices to ensure your code is efficient and easy to maintain:
- Name hooks with
use
at the start: This follows the React convention and allows development tools to correctly identify the hook. - Keep hooks simple: Try to ensure that custom hooks have a clear purpose and do only one thing.
- Document and test hooks: Like any other reusable function, document how to use the hook and ensure it is well-tested.
Conclusion
Custom hooks allow you to encapsulate and reuse stateful and side effect logic in different components. By learning to create your own hooks, you can make your code more modular, efficient, and easier to maintain.
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps
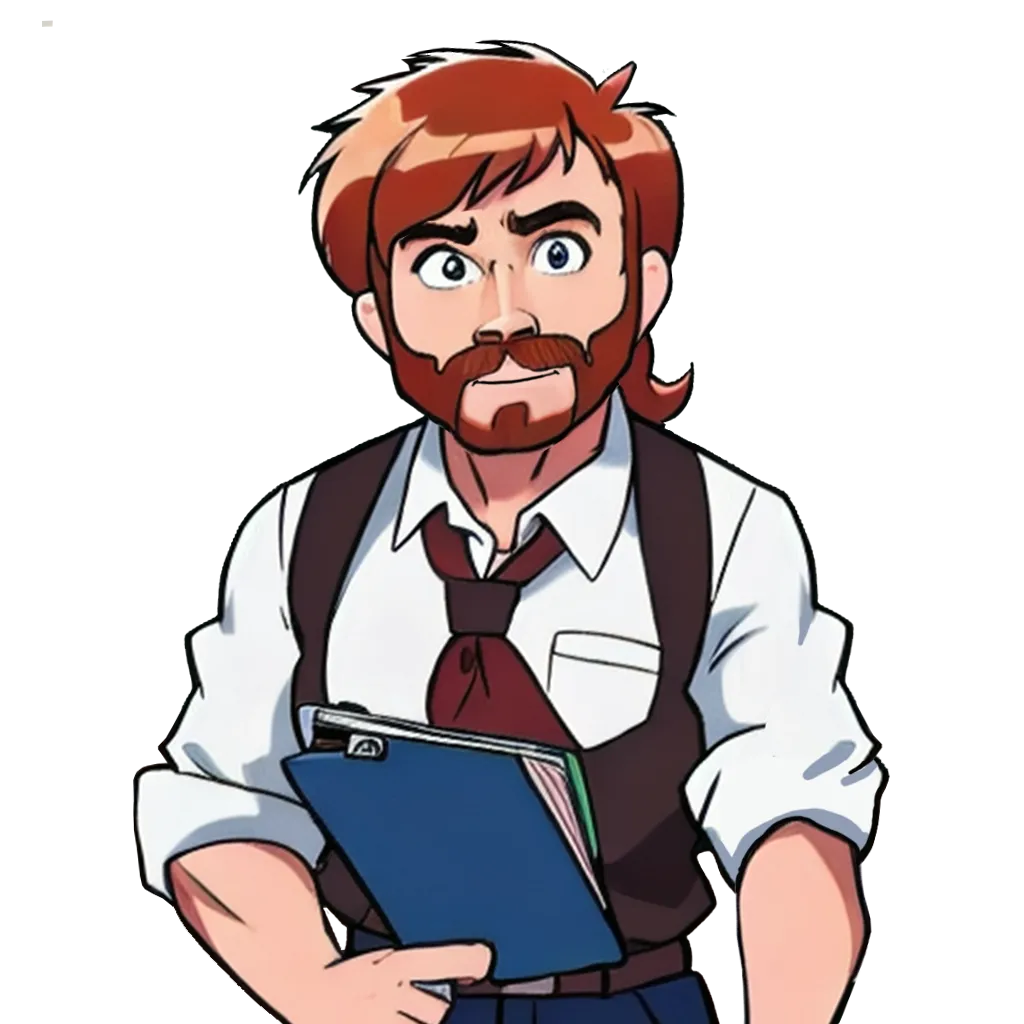