Basic React
Conditional Rendering and Lists
Conditional rendering and list management are two key concepts for building dynamic interfaces in React. In this chapter, we will learn how to use conditional rendering to show or hide interface elements based on certain conditions, and how to efficiently render lists of elements.
Conditional Rendering in React
Conditional rendering in React is similar to conditional expressions in JavaScript. You can use operators like if
, else
, or the ternary operator (? :
) to decide which components or elements to display based on a condition.
Example with if
and else
Here's an example of how to use if
to conditionally render a component:
jsx
This example shows how a component can return different elements based on whether the user is authenticated or not.
Ternary Operator
The ternary operator is a more concise way to handle conditional rendering in React:
jsx
This approach reduces the amount of code and is useful when the conditional logic is simple.
Conditional Rendering with &&
You can use the logical &&
operator to display an element only if a condition is true:
jsx
If props.warn
is false, the component returns null
, which means that nothing is rendered.
List Rendering in React
In React, you can render lists of elements by using JavaScript's map()
method to iterate over an array and return a JSX element for each item.
Basic Example of List Rendering
Here's an example of how to render a list of elements in React:
jsx
It's important to include the key
prop when rendering lists. key
helps React identify which elements have changed, optimizing the rendering process.
Generating Unique Keys for Lists
Keys in React should be unique and stable. You can typically use a unique identifier, such as a database ID, or the array index if no other identifier is available.
jsx
Using the array index is a temporary solution, but whenever possible, it's better to use a unique identifier.
Rendering Components within a List
You're not limited to rendering simple elements like <li>
. You can also render complex components within a list:
jsx
Here, each user in the list is represented by the UserItem
component, which makes the code more modular and reusable.
Handling Lists and Keys with Fragments
In some situations, you might need to render multiple elements from a map()
without an extra wrapper. You can use fragments to avoid adding unnecessary elements to the DOM:
jsx
Using fragments allows the return of multiple elements without adding an extra container like a div
.
Conclusion
Conditional rendering and efficient list handling are key components for creating dynamic and reactive interfaces in React. These concepts will allow you to build interfaces that respond effectively to changes in data and user interactions.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
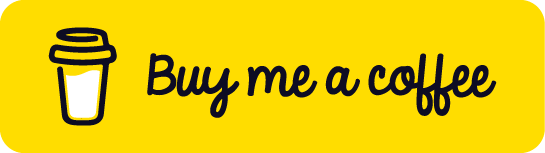
Chat with Chuck
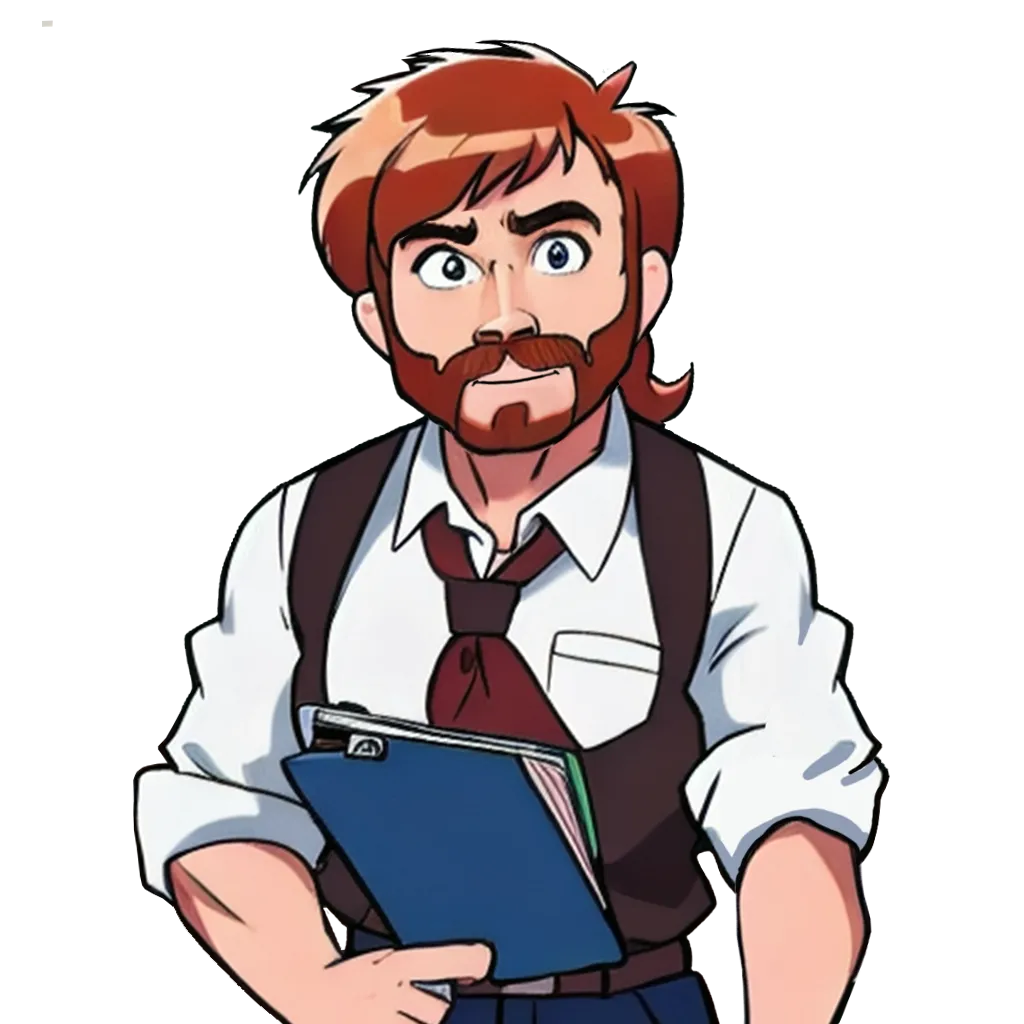
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps