Basic React
React Router: Navigation in Applications
One of the most important features of a web application is the ability to navigate between different pages or views. In React, handling navigation and routes is done using React Router, a popular library that allows creating single-page applications (SPA) with dynamic routes.
In this chapter, we will learn how to set up React Router, handle dynamic routes, and create programmatic navigation.
Installing React Router
To use React Router in your project, you first need to install the library:
bash
React Router DOM is the version of React Router designed for web applications. Once installed, we can start defining routes in our application.
Basic Route Configuration
The first step to using React Router is to wrap your application within the BrowserRouter
component. Then, you can define routes using the Route
and Switch
components.
Basic Configuration Example
jsx
In this example, Switch
ensures that only the first route that matches the current URL is rendered. Route
defines which component to render based on the route.
Navigation Between Routes
To allow users to navigate between routes, you can use the Link
component instead of <a>
tags. This ensures that navigation is handled by React Router without reloading the page.
jsx
The Link
component creates smooth navigation without reloading the page, improving the user experience.
Dynamic Routes
React Router allows creating dynamic routes that can accept parameters, which is useful for pages that depend on specific data, such as user profiles or products.
Dynamic Route Example
jsx
In this example, the route /user/:userId
is dynamic, allowing the UserProfile
component to access the userId
value from the URL and use it to display personalized information.
Programmatic Navigation
Besides using Link
for navigation, you can also navigate programmatically from the code using the useHistory
hook from React Router. This is useful when you need to redirect the user after an action, such as form submission.
Programmatic Navigation Example
jsx
The useHistory
hook provides access to the navigation history, allowing you to redirect the user based on the actions they perform.
Nested Routes
As applications grow, it's common to have nested routes where a page has specific subroutes. React Router allows defining nested routes easily.
Nested Routes Example
jsx
Nested routes allow creating complex navigation structures while maintaining clear code organization.
Redirects and Default Route
React Router also allows redirecting users to a specific route or defining a default route if no matching route is found.
Redirect Example
jsx
The Redirect
component redirects the user from an old route to a new one, while using *
in a route allows handling not-found pages.
Conclusion
React Router is a powerful tool that enables efficient navigation handling in React applications. With features like dynamic routes, programmatic navigation, and nested routes, you can build complex web applications with smooth navigation.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
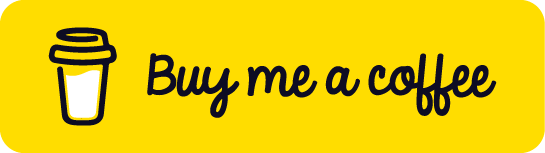
Chat with Chuck
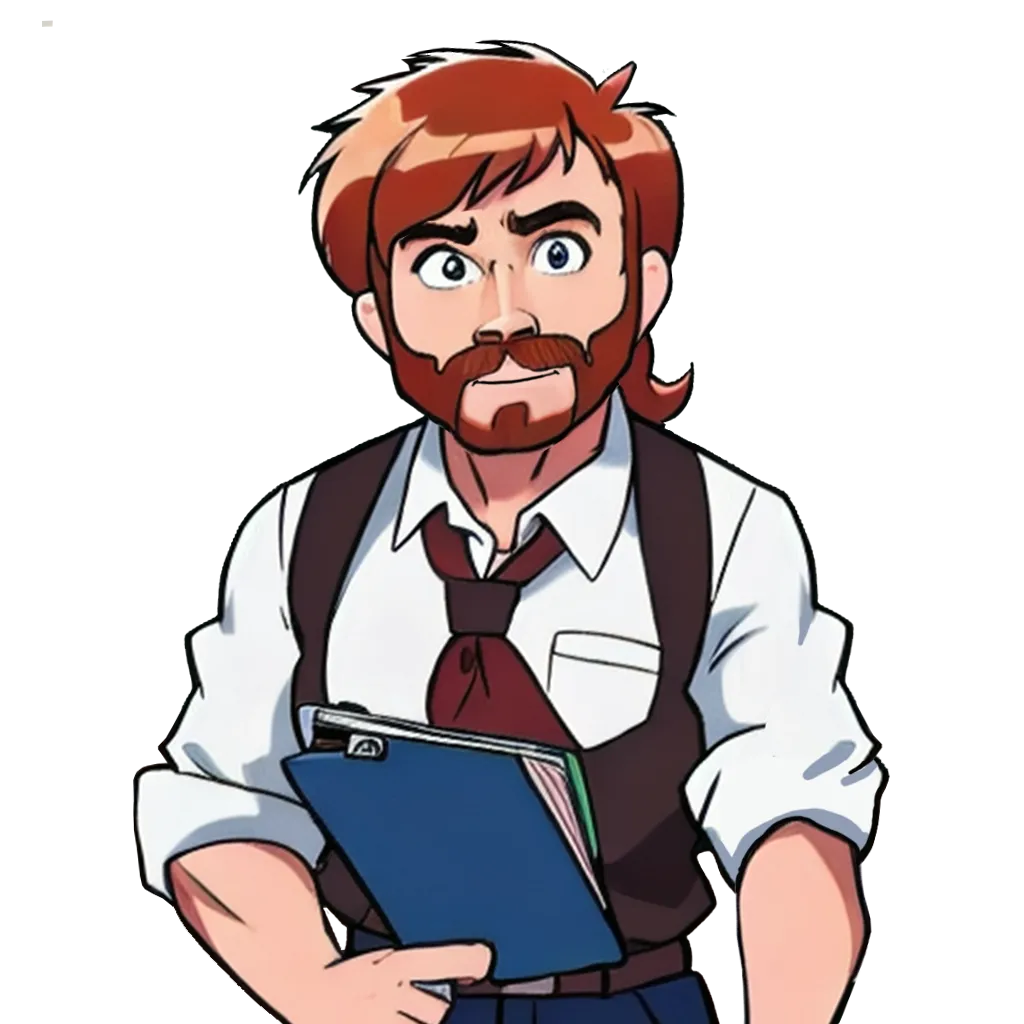
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps