Basic React
Third-Party Components and Useful Libraries
The React ecosystem is vast, and one of its greatest benefits is the number of third-party components and useful libraries available that can simplify application development. From ready-to-use UI components to tools for handling forms, charts, and more, these libraries can save you time and effort.
In this chapter, we will explore some of the most useful and popular libraries that you can incorporate into your React projects to enhance functionality and user experience.
UI Component Libraries
Material-UI (MUI)
Material-UI is one of the most popular component libraries for React. It provides a wide collection of UI components based on Google's Material Design. It is ideal for creating attractive and consistent user interfaces without having to design everything from scratch.
Installation
To install Material-UI, use the following command:
bash
Usage Example
Here is an example of how to use a Material-UI button in React:
jsx
Material-UI also includes customizable themes and a wide variety of components, from buttons to dialogs and tables, which you can integrate into your application.
Ant Design
Ant Design is another popular component library for React, widely used in enterprise applications. It offers sleek, modern design components that include tables, forms, and charts.
Installation
bash
Usage Example
jsx
Ant Design is ideal if you're looking for a complete solution with a rich collection of components and elegant design.
Form Handling
Form handling is a common task in React, and there are several libraries that simplify this task, from validation to state management.
Formik
Formik is one of the most popular libraries for form handling in React. It helps you manage form state, validation, and data submission efficiently.
Installation
bash
Usage Example
Here is a basic example of how to use Formik to handle a form:
jsx
Formik makes it easier to manage state and form validation declaratively.
React Hook Form
Another popular option for handling forms is React Hook Form, which focuses on simplicity and performance. It uses React hooks to manage forms without needing to create additional components or use heavy dependencies.
Installation
bash
Usage Example
jsx
React Hook Form is an excellent option if you want to keep your code lightweight and performance-focused.
Chart Handling
Charts are an important part of many applications, and React has several popular libraries for generating charts and visualizations.
Recharts
Recharts is a React-based charting library that makes it easy to create simple and customizable charts. It supports various types of charts like bar, line, and pie charts.
Installation
bash
Usage Example
jsx
Recharts is ideal for adding interactive and customizable charts to your application easily.
Conclusion
The React ecosystem is filled with third-party components and useful libraries that can speed up your application's development. From UI libraries like Material-UI and Ant Design to solutions for forms and charts, these tools let you focus more on your application's logic without having to reinvent the wheel.
In the next chapter, we will learn about Testing in React, a crucial aspect to ensure the quality and stability of your application.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
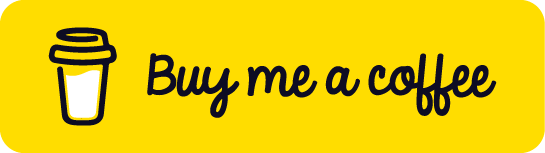
Chat with Chuck
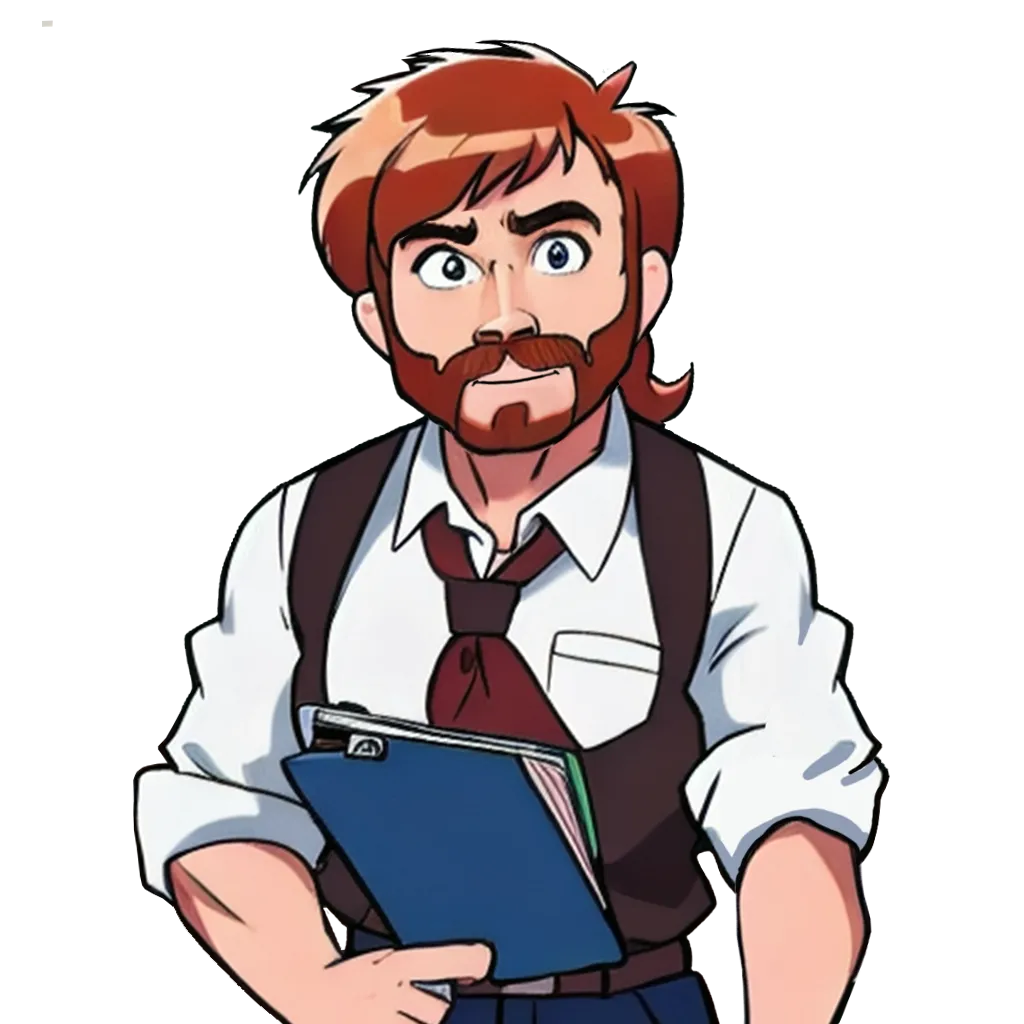
- Introduction to React
- JSX Fundamentals
- Functional Components in React
- State and Lifecycle with Hooks
- Event Handling in React
- Communication between Components
- Conditional Rendering and Lists
- Forms and Input Handling in React
- Styles in React
- React Router: Navigation in Applications
- Advanced State Management
- Performance Optimization in React
- Creating Custom Hooks in React
- Server-Side Rendering (SSR) en React
- Handling APIs and Fetch in React
- Using GraphQL with React
- Testing in React
- Introduction to Class Components
- Third-Party Components and Useful Libraries
- Integration with WebSockets
- Gestión de Archivos y Subidas en React
- Deployment of React Applications
- Best Practices and Patterns in React
- Conclusions and Next Steps